


Deserializing Non-RFC 3339 Time Formats in Go with encoding/json
When using the encoding/json package in Go, the default behavior for unmarshaling time values is to strictly adhere to the RFC 3339 format. However, what if you encounter time formats that deviate from this standard?
Custom Time Type Implementation
To handle such situations, you can implement the json.Marshaler and json.Unmarshaler interfaces on a custom type. This allows you to define custom encoding and decoding logic for your specific time format.
Here's an example of a custom CustomTime type:
type CustomTime struct { time.Time } const ctLayout = "2006/01/02|15:04:05" func (ct *CustomTime) UnmarshalJSON(b []byte) (err error) { s := strings.Trim(string(b), "\"") if s == "null" { ct.Time = time.Time{} return } ct.Time, err = time.Parse(ctLayout, s) return } func (ct *CustomTime) MarshalJSON() ([]byte, error) { if ct.Time.IsZero() { return []byte("null"), nil } return []byte(fmt.Sprintf("\"%s\"", ct.Time.Format(ctLayout))), nil } var nilTime = (time.Time{}).UnixNano() func (ct *CustomTime) IsSet() bool { return !ct.IsZero() }
Usage in a Struct
To use the CustomTime type, you can embed it as a field in a struct:
type Args struct { Time CustomTime }
Example
Here's an example of how to use the Args struct and CustomTime type to unmarshal a JSON string containing a non-RFC 3339 time format:
var data = ` { "Time": "2014/08/01|11:27:18" } ` func main() { a := Args{} fmt.Println(json.Unmarshal([]byte(data), &a)) fmt.Println(a.Time.String()) }
Output:
<nil> 2014-08-01 11:27:18 +0000 UTC</nil>
By implementing the custom CustomTime type, you can handle the deserialization of time formats that are not in RFC 3339 format.
The above is the detailed content of How to Deserialize Non-RFC 3339 Time Formats in Go with `encoding/json`?. For more information, please follow other related articles on the PHP Chinese website!
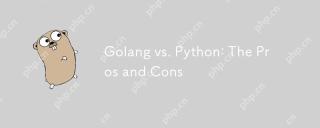
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
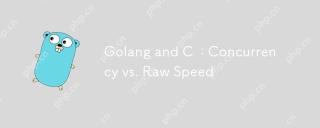
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
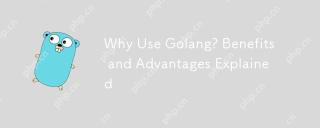
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
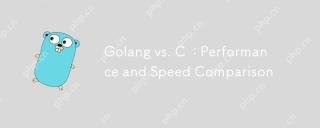
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
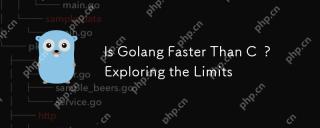
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
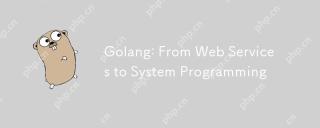
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
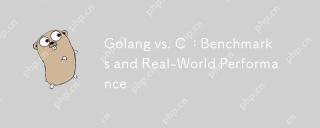
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
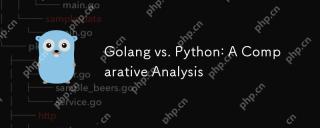
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
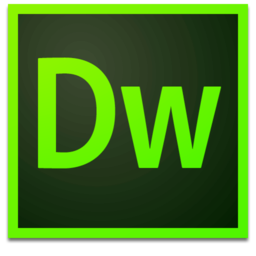
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
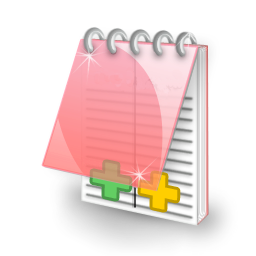
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function