


Trigger CSS Animations in JavaScript without jQuery
Problem:
You want to trigger a CSS animation when the user scrolls the page but without using jQuery.
Solution:
One way to trigger CSS animations in JavaScript is by using the classList property. Here's how you can do it:
-
Create a CSS class with the animation properties.
For example:
.animated { animation: my-animation 2s forwards; }
-
Add an event listener to the document.
The scroll event is fired whenever the user scrolls the page. You can use this event to trigger your animation. Here's how:
document.addEventListener('scroll', (event) => { // Get the element you want to animate. const element = document.getElementById('my-element'); // Add the 'animated' class to the element. element.classList.add('animated'); });
-
Remove the 'animated' class when the animation is complete.
You can use the animationend event to detect when the animation has finished. Here's how:
element.addEventListener('animationend', (event) => { // Remove the 'animated' class from the element. element.classList.remove('animated'); });
Example:
Here's a complete example of how to trigger a CSS animation when the user scrolls the page:
<style> .animated { animation: my-animation 2s forwards; } @keyframes my-animation { 0% { opacity: 0; } 100% { opacity: 1; } } </style> <div></div>
The above is the detailed content of How Can I Trigger CSS Animations with JavaScript on Scroll Without jQuery?. For more information, please follow other related articles on the PHP Chinese website!
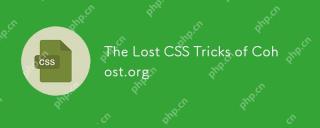
In this post, Blackle Mori shows you a few of the hacks found while trying to push the limits of Cohost’s HTML support. Use these if you dare, lest you too get labelled a CSS criminal.
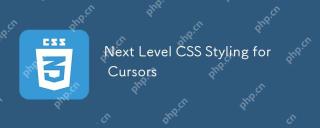
Custom cursors with CSS are great, but we can take things to the next level with JavaScript. Using JavaScript, we can transition between cursor states, place dynamic text within the cursor, apply complex animations, and apply filters.
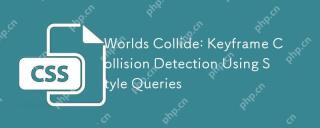
Interactive CSS animations with elements ricocheting off each other seem more plausible in 2025. While it’s unnecessary to implement Pong in CSS, the increasing flexibility and power of CSS reinforce Lee's suspicion that one day it will be a
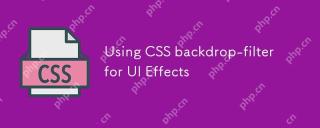
Tips and tricks on utilizing the CSS backdrop-filter property to style user interfaces. You’ll learn how to layer backdrop filters among multiple elements, and integrate them with other CSS graphical effects to create elaborate designs.
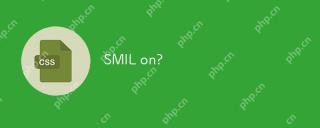
Well, it turns out that SVG's built-in animation features were never deprecated as planned. Sure, CSS and JavaScript are more than capable of carrying the load, but it's good to know that SMIL is not dead in the water as previously
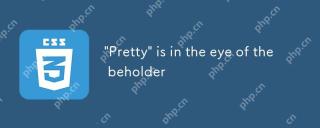
Yay, let's jump for text-wrap: pretty landing in Safari Technology Preview! But beware that it's different from how it works in Chromium browsers.
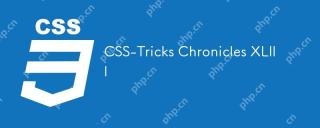
This CSS-Tricks update highlights significant progress in the Almanac, recent podcast appearances, a new CSS counters guide, and the addition of several new authors contributing valuable content.
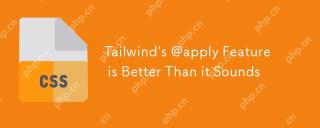
Most of the time, people showcase Tailwind's @apply feature with one of Tailwind's single-property utilities (which changes a single CSS declaration). When showcased this way, @apply doesn't sound promising at all. So obvio


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
