


What is the difference between (R, 1) and (R,) shapes in NumPy?
In NumPy, a single-dimension array can be represented in two ways: as a shape (R, 1) (a list of numbers) or as a shape (R,) (a list of lists). Both of these shapes represent the same underlying data, but they have different implications for matrix multiplication.
When you multiply two matrices, their shapes must be compatible. If one matrix has a shape (R, 1) and the other matrix has a shape (R,), NumPy will raise an error because the shapes are not aligned. This is because (R, 1) is a two-dimensional shape, while (R,) is a one-dimensional shape.
To fix this error, you can explicitly reshape one of the matrices. For example:
import numpy as np M = np.array([[1, 2, 3], [4, 5, 6]]) ones = np.ones((M.shape[0], 1)) result = np.dot(M[:,0].reshape((M.shape[0], 1)), ones)
In this example, we reshape the first column of M (a shape (R,)) to a shape (R, 1) using the reshape() method. This makes the shapes of the two matrices compatible, and the multiplication can be performed successfully.
Are there better ways to do the above example without explicitly reshaping?
Yes, there are better ways to do the above example without explicitly reshaping. One way is to use the sum() method with the axis argument. For example:
import numpy as np M = np.array([[1, 2, 3], [4, 5, 6]]) ones = np.ones((M.shape[0], 1)) result = np.dot(M[:,0], ones) + M[:,1:]
In this example, we use the sum() method to sum the first column of M with the remaining columns. This gives us a matrix with the same shape as M. We can then perform the multiplication without any errors.
Another way to do the above example without explicitly reshaping is to use the broadcast() function. For example:
import numpy as np M = np.array([[1, 2, 3], [4, 5, 6]]) ones = np.ones((M.shape[0], 1)) result = np.dot( np.broadcast_to(M[:,0], M.shape), ones)
In this example, we use the broadcast() function to broadcast the first column of M to the shape of M. This makes the shapes of the two matrices compatible, and the multiplication can be performed successfully.
The above is the detailed content of What's the Difference Between NumPy's (R, 1) and (R,) Shapes, and How Can Matrix Multiplication Issues Be Resolved?. For more information, please follow other related articles on the PHP Chinese website!
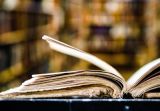
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
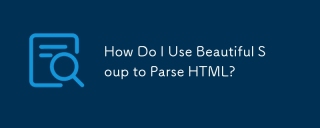
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
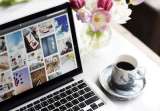
Dealing with noisy images is a common problem, especially with mobile phone or low-resolution camera photos. This tutorial explores image filtering techniques in Python using OpenCV to tackle this issue. Image Filtering: A Powerful Tool Image filter
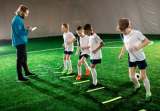
Python, a favorite for data science and processing, offers a rich ecosystem for high-performance computing. However, parallel programming in Python presents unique challenges. This tutorial explores these challenges, focusing on the Global Interprete
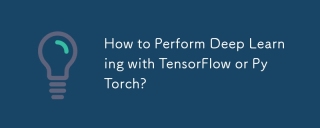
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
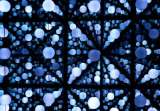
This tutorial demonstrates creating a custom pipeline data structure in Python 3, leveraging classes and operator overloading for enhanced functionality. The pipeline's flexibility lies in its ability to apply a series of functions to a data set, ge
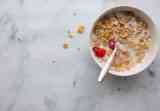
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
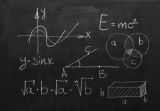
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
