


How Generator Comprehensions Work
Generator comprehensions are a powerful Python feature that allows you to create an iterable that generates elements on an as-needed basis. Unlike list comprehensions, which create a complete list in memory, generator comprehensions stream elements one at a time, making them more memory-efficient for large datasets.
Generator Expression Syntax
A generator expression is enclosed in parentheses and follows a similar syntax to a list comprehension:
generator = (expression for element in iterable if condition)
For example, the following generator comprehension creates a sequence of doubled numbers:
my_generator = (x * 2 for x in [1, 2, 3, 4, 5])
How Generator Comprehensions Work
Generator comprehensions work by yielding elements, one at a time, based on the expression specified. This is in contrast to list comprehensions, which create an entire list of elements in memory before returning the result.
To retrieve elements from a generator, you can use the next() function or iterate over it using a for loop:
next(my_generator) # Yields the first element for element in my_generator: print(element) # Iterates over remaining elements
Memory Efficiency
Generator comprehensions are particularly useful when dealing with large datasets because they stream elements one at a time, without needing to store the entire result in memory. This can significantly reduce memory consumption compared to list comprehensions.
When to Use Generator Comprehensions
Use generator comprehensions when:
- You need to generate elements on an as-needed basis.
- Memory efficiency is a concern for large datasets.
- You need to iterate over a stream of data one element at a time.
Use list comprehensions when:
- You need all elements before proceeding with your program.
- Memory usage is not an issue.
- You need to perform complex operations on the entire collection.
The above is the detailed content of How Do Generator Comprehensions Achieve Memory Efficiency in Python?. For more information, please follow other related articles on the PHP Chinese website!
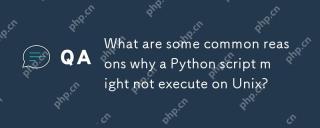
The reasons why Python scripts cannot run on Unix systems include: 1) Insufficient permissions, using chmod xyour_script.py to grant execution permissions; 2) Shebang line is incorrect or missing, you should use #!/usr/bin/envpython; 3) The environment variables are not set properly, and you can print os.environ debugging; 4) Using the wrong Python version, you can specify the version on the Shebang line or the command line; 5) Dependency problems, using virtual environment to isolate dependencies; 6) Syntax errors, using python-mpy_compileyour_script.py to detect.
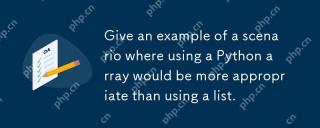
Using Python arrays is more suitable for processing large amounts of numerical data than lists. 1) Arrays save more memory, 2) Arrays are faster to operate by numerical values, 3) Arrays force type consistency, 4) Arrays are compatible with C arrays, but are not as flexible and convenient as lists.
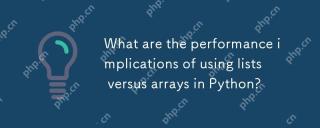
Listsare Better ForeflexibilityandMixdatatatypes, Whilearraysares Superior Sumerical Computation Sand Larged Datasets.1) Unselable List Xibility, MixedDatatypes, andfrequent elementchanges.2) Usarray's sensory -sensical operations, Largedatasets, AndwhenMemoryEfficiency
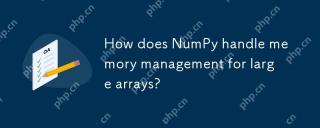
NumPymanagesmemoryforlargearraysefficientlyusingviews,copies,andmemory-mappedfiles.1)Viewsallowslicingwithoutcopying,directlymodifyingtheoriginalarray.2)Copiescanbecreatedwiththecopy()methodforpreservingdata.3)Memory-mappedfileshandlemassivedatasetsb
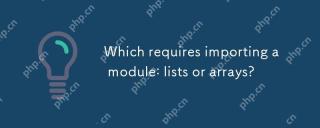
ListsinPythondonotrequireimportingamodule,whilearraysfromthearraymoduledoneedanimport.1)Listsarebuilt-in,versatile,andcanholdmixeddatatypes.2)Arraysaremorememory-efficientfornumericdatabutlessflexible,requiringallelementstobeofthesametype.
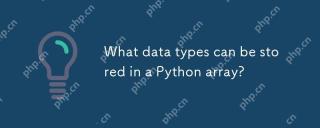
Pythonlistscanstoreanydatatype,arraymodulearraysstoreonetype,andNumPyarraysarefornumericalcomputations.1)Listsareversatilebutlessmemory-efficient.2)Arraymodulearraysarememory-efficientforhomogeneousdata.3)NumPyarraysareoptimizedforperformanceinscient
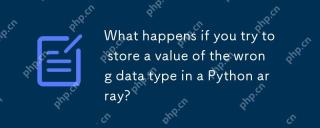
WhenyouattempttostoreavalueofthewrongdatatypeinaPythonarray,you'llencounteraTypeError.Thisisduetothearraymodule'sstricttypeenforcement,whichrequiresallelementstobeofthesametypeasspecifiedbythetypecode.Forperformancereasons,arraysaremoreefficientthanl
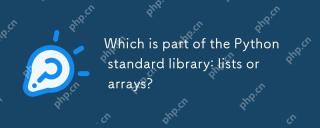
Pythonlistsarepartofthestandardlibrary,whilearraysarenot.Listsarebuilt-in,versatile,andusedforstoringcollections,whereasarraysareprovidedbythearraymoduleandlesscommonlyusedduetolimitedfunctionality.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
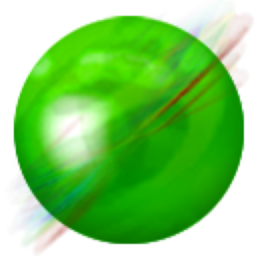
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
