Lambdas vs. Bind in C : Polymorphism and Efficiency
When comparing the usage of C 0x lambdas and std::bind for similar tasks, the choice between the two can be influenced by factors such as polymorphism and efficiency.
Bind vs. Lambda in Detail
Consider the following example where both lambda and bind are used to generate random numbers using a distribution and engine:
// Using lambda auto dice = [&]() { return distribution(engine); }; // Using bind auto dice = bind(distribution, engine);
Polymorphism
Unlike lambdas, which are monomorphic (i.e., have fixed types), std::bind allows for polymorphic behavior. This means that bind can be used to create functions with unknown types, as demonstrated below:
struct foo { typedef void result_type; template <typename a typename b> void operator()(A a, B b) { cout <p>In this example, the types of a and b are deduced at runtime when f is invoked. This flexibility is unavailable with lambdas.</p> <p><strong>Efficiency</strong></p> <p>In general, lambdas tend to be more efficient than bind when the captured variables are used by value. This is because lambdas capture variables directly, while bind creates a closure object that references the captured variables. However, bind may offer advantages when the captured variables are large or when the function is called frequently.</p> <p><strong>Conclusion</strong></p> <p>The choice between lambdas and bind depends on the specific requirements of the application. Lambdas provide polymorphism and are efficient for capturing small, value-type variables. Bind offers greater flexibility and may be preferable when dealing with large or frequently called functions.</p></typename>
The above is the detailed content of Lambdas vs. Bind in C : When Should You Choose Each?. For more information, please follow other related articles on the PHP Chinese website!
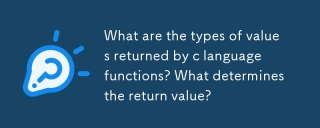
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
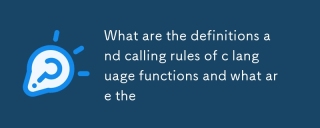
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi
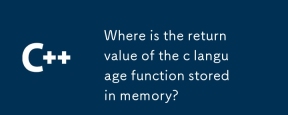
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
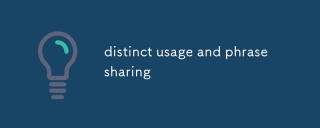
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
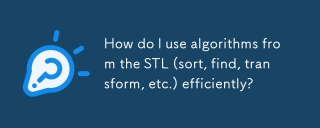
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
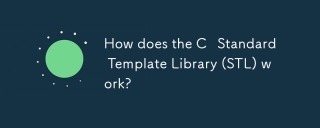
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
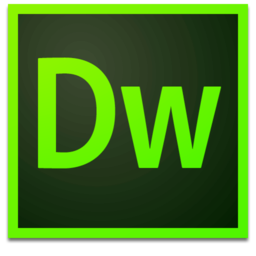
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
