


Flattening Nested JSON
Flattening nested JSON data into a single level structure is a common task in data processing. Here's how you can achieve this in Go using a custom UnmarshalJSON function:
UnmarshalJSON Function
Custom UnmarshalJSON functions allow Go structs to handle the unmarshalling of JSON data. Here's your updated Social struct with the UnmarshalJSON implementation:
<code class="go">type Social struct { GooglePlusPlusOnes uint32 `Social:"GooglePlusOne"` TwitterTweets uint32 `json:"Twitter"` LinkedinShares uint32 `json:"LinkedIn"` PinterestPins uint32 `json:"Pinterest"` StumbleuponStumbles uint32 `json:"StumbleUpon"` DeliciousBookmarks uint32 `json:"Delicious"` // Custom unmarshalling for the Facebook fields FacebookLikes uint32 `json:"-"` FacebookShares uint32 `json:"-"` FacebookComments uint32 `json:"-"` FacebookTotal uint32 `json:"-"` } // UnmarshalJSON implements the Unmarshaler interface for custom JSON unmarshalling func (s *Social) UnmarshalJSON(data []byte) error { type FacebookAlias Facebook aux := &struct { Facebook FacebookAlias `json:"Facebook"` }{} if err := json.Unmarshal(data, aux); err != nil { return err } s.FacebookLikes = aux.Facebook.FacebookLikes s.FacebookShares = aux.Facebook.FacebookShares s.FacebookComments = aux.Facebook.FacebookComments s.FacebookTotal = aux.Facebook.FacebookTotal return nil }</code>
Usage
With the updated Social struct, you can now unmarshal your JSON document and flatten it:
<code class="go">package main import ( "encoding/json" "fmt" ) type Social struct { // ... (same as before) } func (s *Social) UnmarshalJSON(data []byte) error { // ... (same as before) } func main() { var jsonBlob = []byte(`[ {"StumbleUpon":0,"Reddit":0,"Facebook":{"commentsbox_count":4691,"click_count":0,"total_count":298686,"comment_count":38955,"like_count":82902,"share_count":176829},"Delicious":0,"GooglePlusOne":275234,"Buzz":0,"Twitter":7346788,"Diggs":0,"Pinterest":40982,"LinkedIn":0} ]`) var social []Social err := json.Unmarshal(jsonBlob, &social) if err != nil { fmt.Println("error:", err) } fmt.Printf("%+v", social) }</code>
This code will output your desired flattened JSON structure, with Facebook fields merged into the top-level Social struct.
The above is the detailed content of How can I flatten nested JSON data into a single level structure using custom UnmarshalJSON functions in Go?. For more information, please follow other related articles on the PHP Chinese website!
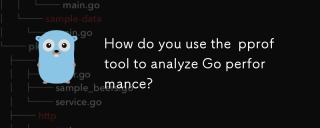
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
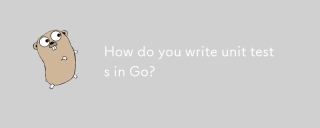
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
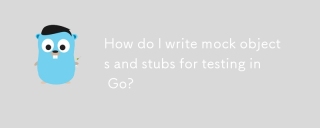
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
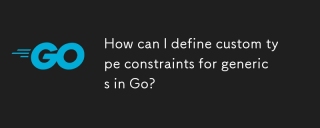
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
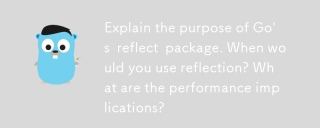
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
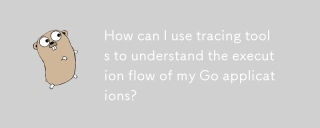
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
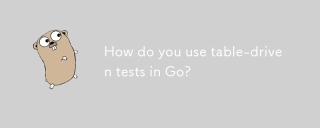
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
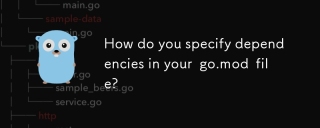
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
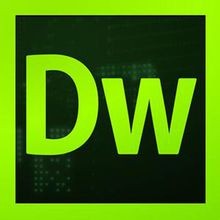
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
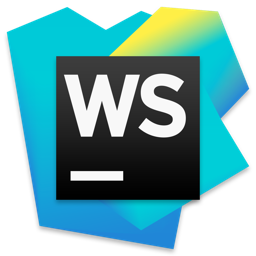
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
