


Shutting Down HTTP Server After Returning Response
In this scenario, you have built a Go command-line bot that interacts with the Instagram API. To obtain the access token, you start a local HTTP server to capture the redirect URI. However, you encounter an issue while attempting to manually shut down the server after retrieving the access token.
Problem:
"Server Closed" and "Invalid Memory Address"Errors Occur When Shutting Down the Server
Analysis:
The errors you encounter indicate that the HTTP server is being shut down prematurely before all connections are closed gracefully. This can occur due to asynchronous behavior in the HTTP handler and the server shutdown process.
Solution 1: Using Context.WithCancel:
Consider using context.WithCancel to create a context that can be canceled when the access token is retrieved. Pass this context to the HTTP handler and use CancelFunc to gracefully shut down the server when the context is closed.
<code class="go">package main import ( "context" "io" "log" "net/http" ) func main() { ctx, cancel := context.WithCancel(context.Background()) http.HandleFunc("/quit", func(w http.ResponseWriter, r *http.Request) { io.WriteString(w, "Bye\n") cancel() }) http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { io.WriteString(w, "Hi\n") }) srv := &http.Server{Addr: ":8080"} go func() { err := srv.ListenAndServe() if err != http.ErrServerClosed { log.Println(err) } }() <h3 id="Solution-Using-Same-Context-Across-Functions">Solution 2: Using Same Context Across Functions:</h3> <p>You can also use the same context across multiple HTTP handlers and the main function to ensure that all functions are notified when the server should shut down.</p> <pre class="brush:php;toolbar:false"><code class="go">package main import ( "context" "io" "log" "net/http" ) func main() { ctx, cancel := context.WithCancel(context.Background()) http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { io.WriteString(w, "Hi\n") }) http.HandleFunc("/quit", func(w http.ResponseWriter, r *http.Request) { io.WriteString(w, "Bye\n") cancel() }) srv := &http.Server{Addr: ":8080"} go func() { if err := srv.ListenAndServe(); err != nil { log.Printf("Httpserver: ListenAndServe() error: %s", err) } }() <h3 id="Conclusion">Conclusion:</h3> <p>By using context.WithCancel or passing the same context across multiple functions, you can ensure that the HTTP server shuts down gracefully after all connections have been closed. This will prevent the errors you are currently encountering.</p></code>
The above is the detailed content of How to Gracefully Shut Down a Go HTTP Server After Returning a Response?. For more information, please follow other related articles on the PHP Chinese website!
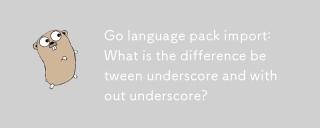
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
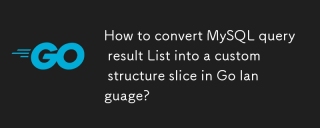
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
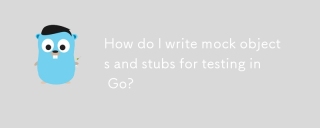
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
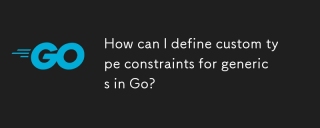
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
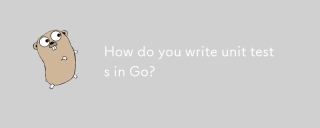
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
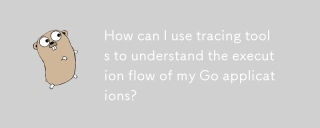
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
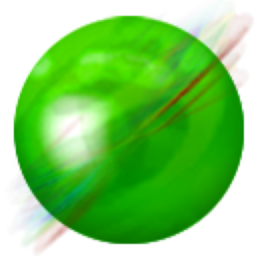
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
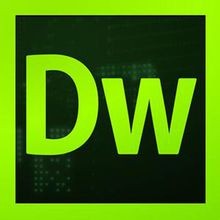
Dreamweaver CS6
Visual web development tools
