Comparing Go's Error Handling Approach to Other Languages
Go's error handling returns errors as values, unlike Java and Python which use exceptions. 1) Go's method ensures explicit error handling, promoting robust code but increasing verbosity. 2) Java and Python's exceptions allow for cleaner code but can lead to overlooked errors if not managed carefully.
When we dive into the world of programming, one aspect that often stirs up heated debates is error handling. Go, with its unique approach, has certainly added a new flavor to this discussion. So, how does Go's error handling compare to other languages? Let's unpack this.
Go's philosophy on error handling is centered around explicitness and simplicity. Unlike many other languages, Go doesn't use exceptions. Instead, it returns errors as values. This method forces developers to deal with errors directly, which can lead to more robust code but also requires more verbosity. When we compare this to languages like Java or Python, which use exceptions, the difference in approach becomes stark.
In languages like Java, exceptions are thrown when errors occur, and they can be caught and handled using try-catch blocks. This can make the code cleaner at first glance, but it also means that errors can be easily ignored if not properly managed. Python takes a similar approach but with a more flexible syntax, allowing for both explicit error handling and the use of exceptions.
Now, let's explore how Go's approach stands out and what we can learn from it.
Go's error handling is all about returning errors as values. Here's a simple example:
func divide(a, b float64) (float64, error) { if b == 0 { return 0, errors.New("division by zero") } return a / b, nil } <p>func main() { result, err := divide(10, 0) if err != nil { fmt.Println(err) // 输出: division by zero return } fmt.Println(result) }</p>
This approach ensures that errors are always visible in the function signature and must be dealt with explicitly. It's like having a constant reminder to handle errors, which can be both a blessing and a curse.
The beauty of Go's method lies in its simplicity and explicitness. It's straightforward to understand where errors might occur and how they're handled. But it also means more lines of code and potentially more clutter, especially in functions that might return multiple values.
On the flip side, languages like Java and Python can hide errors behind try-catch blocks, which can lead to cleaner code but also to overlooked errors. In Java, you might see something like this:
public class Calculator { public static double divide(double a, double b) { if (b == 0) { throw new ArithmeticException("Division by zero"); } return a / b; } <pre class='brush:php;toolbar:false;'>public static void main(String[] args) { try { double result = divide(10, 0); System.out.println(result); } catch (ArithmeticException e) { System.out.println(e.getMessage()); // 输出: Division by zero } }
}
Python's approach is similar but often more concise:
def divide(a, b): if b == 0: raise ZeroDivisionError("Division by zero") return a / b <p>try: result = divide(10, 0) print(result) except ZeroDivisionError as e: print(e) # 输出: Division by zero</p>
Each of these methods has its pros and cons. Go's approach might feel more cumbersome at first, but it encourages a culture of error handling that can lead to more reliable software. Java and Python's exception-based systems can make code more readable but require discipline to ensure errors are not ignored.
In my experience, Go's error handling can be a double-edged sword. On one hand, it forces you to think about errors at every step, which can lead to more robust applications. On the other hand, it can make your code more verbose, which might not be ideal for quick prototypes or scripts.
One trick I've learned with Go is to use custom error types to provide more context about errors. For example:
type DivisionError struct { numerator, denominator float64 } <p>func (e *DivisionError) Error() string { return fmt.Sprintf("cannot divide %v by %v", e.numerator, e.denominator) }</p><p>func divide(a, b float64) (float64, error) { if b == 0 { return 0, &DivisionError{a, b} } return a / b, nil }</p><p>func main() { result, err := divide(10, 0) if err != nil { fmt.Println(err) // 输出: cannot divide 10 by 0 return } fmt.Println(result) }</p>
This approach allows you to provide more detailed error messages, which can be incredibly helpful in debugging and understanding the context of errors.
When comparing Go's error handling to other languages, it's important to consider the trade-offs. Go's method might not be the most elegant, but it's effective in promoting error awareness. Languages like Java and Python, with their exception-based systems, offer flexibility and readability but require careful management to ensure errors aren't overlooked.
In the end, the choice of error handling approach depends on your project's needs, your team's preferences, and the kind of software you're building. Go's method might be perfect for systems programming where reliability is paramount, while Java or Python's exception-based systems might be better suited for applications where readability and ease of use are key.
As a seasoned developer, I've learned to appreciate the nuances of each approach. Go's explicit error handling has taught me to be more diligent about error management, while working with exceptions in Java and Python has shown me the value of concise and readable code. The key is to understand the strengths and weaknesses of each method and choose the one that best fits your project's goals.
The above is the detailed content of Comparing Go's Error Handling Approach to Other Languages. For more information, please follow other related articles on the PHP Chinese website!
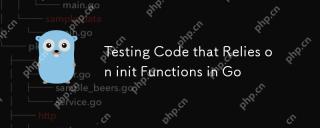
WhentestingGocodewithinitfunctions,useexplicitsetupfunctionsorseparatetestfilestoavoiddependencyoninitfunctionsideeffects.1)Useexplicitsetupfunctionstocontrolglobalvariableinitialization.2)Createseparatetestfilestobypassinitfunctionsandsetupthetesten
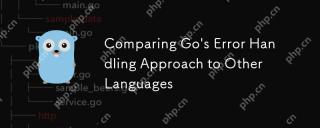
Go'serrorhandlingreturnserrorsasvalues,unlikeJavaandPythonwhichuseexceptions.1)Go'smethodensuresexpliciterrorhandling,promotingrobustcodebutincreasingverbosity.2)JavaandPython'sexceptionsallowforcleanercodebutcanleadtooverlookederrorsifnotmanagedcare
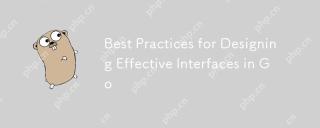
AneffectiveinterfaceinGoisminimal,clear,andpromotesloosecoupling.1)Minimizetheinterfaceforflexibilityandeaseofimplementation.2)Useinterfacesforabstractiontoswapimplementationswithoutchangingcallingcode.3)Designfortestabilitybyusinginterfacestomockdep
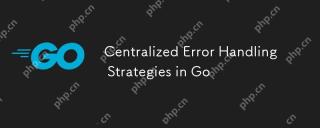
Centralized error handling can improve the readability and maintainability of code in Go language. Its implementation methods and advantages include: 1. Separate error handling logic from business logic and simplify code. 2. Ensure the consistency of error handling by centrally handling. 3. Use defer and recover to capture and process panics to enhance program robustness.
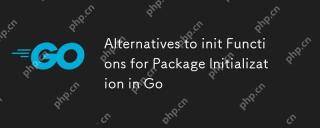
InGo,alternativestoinitfunctionsincludecustominitializationfunctionsandsingletons.1)Custominitializationfunctionsallowexplicitcontroloverwheninitializationoccurs,usefulfordelayedorconditionalsetups.2)Singletonsensureone-timeinitializationinconcurrent
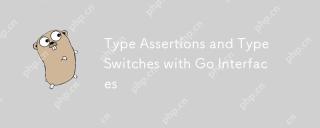
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
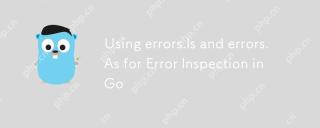
Go language error handling becomes more flexible and readable through errors.Is and errors.As functions. 1.errors.Is is used to check whether the error is the same as the specified error and is suitable for the processing of the error chain. 2.errors.As can not only check the error type, but also convert the error to a specific type, which is convenient for extracting error information. Using these functions can simplify error handling logic, but pay attention to the correct delivery of error chains and avoid excessive dependence to prevent code complexity.
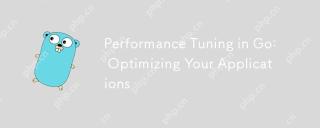
TomakeGoapplicationsrunfasterandmoreefficiently,useprofilingtools,leverageconcurrency,andmanagememoryeffectively.1)UsepprofforCPUandmemoryprofilingtoidentifybottlenecks.2)Utilizegoroutinesandchannelstoparallelizetasksandimproveperformance.3)Implement


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
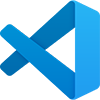
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
