An effective interface in Go is minimal, clear, and promotes loose coupling. 1) Minimize the interface for flexibility and ease of implementation. 2) Use interfaces for abstraction to swap implementations without changing calling code. 3) Design for testability by using interfaces to mock dependencies, enhancing unit testing.
When it comes to designing effective interfaces in Go, the question often arises: what makes an interface truly effective? An effective interface in Go is one that is minimal, clear, and promotes loose coupling between components. It's about defining just enough to achieve the desired functionality without over-specifying, which allows for greater flexibility and easier testing.
In my journey with Go, I've come to appreciate the power of interfaces in crafting clean, maintainable code. The beauty of Go's interfaces lies in their implicit nature—you don't need to explicitly declare that a type implements an interface; it just does if it satisfies the method set. This leads to a fascinating world of duck typing in a statically typed language, which I'll dive into as we explore the nuances of interface design.
Let's dive right into the world of Go interfaces and see how we can harness their potential to create robust, flexible systems.
Go's interfaces are a cornerstone of its type system, enabling polymorphism and abstraction. They allow you to define behaviors that types can implement, which is crucial for writing modular and testable code. When designing interfaces, I've learned to focus on a few key principles that guide the process:
Minimize the Interface: The smaller the interface, the easier it is to implement and the more flexible it becomes. A common pitfall is to create interfaces that are too broad, leading to tight coupling and reduced testability.
Use Interfaces for Abstraction: Interfaces should be used to abstract away the concrete implementation details, allowing you to swap out different implementations without changing the calling code.
Design for Testability: Interfaces make it easier to write unit tests by allowing you to mock dependencies. This is a powerful aspect of Go's interface system that I've leveraged extensively in my projects.
Let's look at a practical example to illustrate these principles. Suppose we're building a simple payment processing system. We want to define an interface for payment methods that can be implemented by different payment providers.
type PaymentMethod interface { Charge(amount float64) error Refund(amount float64) error }
This interface is minimal, focusing only on the essential operations of charging and refunding. Now, let's implement this interface for a credit card payment method:
type CreditCard struct { Number string Expiry string CVV string } func (cc *CreditCard) Charge(amount float64) error { // Implementation for charging a credit card return nil } func (cc *CreditCard) Refund(amount float64) error { // Implementation for refunding a credit card return nil }
And for a PayPal payment method:
type PayPal struct { Email string } func (pp *PayPal) Charge(amount float64) error { // Implementation for charging via PayPal return nil } func (pp *PayPal) Refund(amount float64) error { // Implementation for refunding via PayPal return nil }
Now, we can use these payment methods interchangeably in our system:
func ProcessPayment(method PaymentMethod, amount float64) error { return method.Charge(amount) } func main() { cc := &CreditCard{Number: "1234567890123456", Expiry: "12/2025", CVV: "123"} pp := &PayPal{Email: "user@example.com"} if err := ProcessPayment(cc, 100.0); err != nil { log.Fatal(err) } if err := ProcessPayment(pp, 50.0); err != nil { log.Fatal(err) } }
This example showcases the power of interfaces in Go. By defining a minimal interface, we've created a system that is flexible and easy to extend with new payment methods.
When designing interfaces, it's crucial to consider the trade-offs. While minimal interfaces are desirable, they can sometimes lead to the proliferation of small interfaces, which can be confusing. It's a balancing act—defining interfaces that are just large enough to be useful but not so large that they become cumbersome.
Another aspect to consider is the performance impact of interfaces. In Go, using interfaces can introduce a slight overhead due to the dynamic dispatch mechanism. However, this overhead is usually negligible unless you're dealing with extremely performance-critical code. In most cases, the benefits of using interfaces far outweigh the minor performance costs.
In terms of best practices, I've found the following to be invaluable:
Use Interface Segregation: Instead of having one large interface, break it down into smaller, more focused interfaces. This aligns with the Interface Segregation Principle of SOLID design.
Avoid Interface Pollution: Don't create interfaces for the sake of having them. Only define an interface if you have multiple implementations or if you need to mock it for testing.
Document Your Interfaces: Clear documentation is crucial for interfaces, as they define contracts that other parts of your code will rely on. Use Go's documentation comments to explain the purpose and behavior of each method.
Consider the Zero-Value: In Go, types often have useful zero-values. Ensure that your interfaces work correctly with zero-values of the implementing types, or document why they don't.
Test Your Interfaces: Write tests that verify the behavior of your interfaces. This not only ensures that your implementations are correct but also helps in understanding the interface's contract.
In my experience, one of the most common pitfalls in interface design is over-specification. It's tempting to add methods to an interface that you think might be useful in the future, but this can lead to rigid designs that are hard to change. Instead, start with the minimal set of methods you need and evolve the interface as your system grows.
Another challenge is ensuring that your interfaces are truly abstract. It's easy to fall into the trap of designing interfaces that are too closely tied to a specific implementation. To avoid this, always ask yourself if the interface could be implemented in multiple ways. If not, it might be too specific.
In conclusion, designing effective interfaces in Go is an art that requires a balance of minimalism, abstraction, and foresight. By following these best practices and being mindful of the trade-offs, you can create interfaces that make your Go code more flexible, maintainable, and testable. Remember, the goal is to define just enough to achieve the desired functionality without over-specifying, allowing your system to evolve gracefully over time.
The above is the detailed content of Best Practices for Designing Effective Interfaces in Go. For more information, please follow other related articles on the PHP Chinese website!
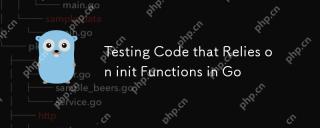
WhentestingGocodewithinitfunctions,useexplicitsetupfunctionsorseparatetestfilestoavoiddependencyoninitfunctionsideeffects.1)Useexplicitsetupfunctionstocontrolglobalvariableinitialization.2)Createseparatetestfilestobypassinitfunctionsandsetupthetesten
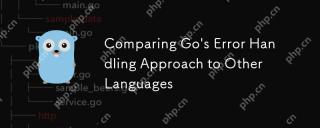
Go'serrorhandlingreturnserrorsasvalues,unlikeJavaandPythonwhichuseexceptions.1)Go'smethodensuresexpliciterrorhandling,promotingrobustcodebutincreasingverbosity.2)JavaandPython'sexceptionsallowforcleanercodebutcanleadtooverlookederrorsifnotmanagedcare
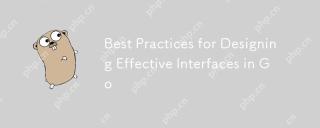
AneffectiveinterfaceinGoisminimal,clear,andpromotesloosecoupling.1)Minimizetheinterfaceforflexibilityandeaseofimplementation.2)Useinterfacesforabstractiontoswapimplementationswithoutchangingcallingcode.3)Designfortestabilitybyusinginterfacestomockdep
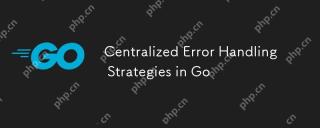
Centralized error handling can improve the readability and maintainability of code in Go language. Its implementation methods and advantages include: 1. Separate error handling logic from business logic and simplify code. 2. Ensure the consistency of error handling by centrally handling. 3. Use defer and recover to capture and process panics to enhance program robustness.
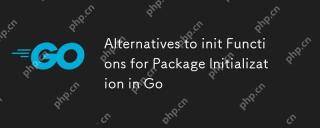
InGo,alternativestoinitfunctionsincludecustominitializationfunctionsandsingletons.1)Custominitializationfunctionsallowexplicitcontroloverwheninitializationoccurs,usefulfordelayedorconditionalsetups.2)Singletonsensureone-timeinitializationinconcurrent
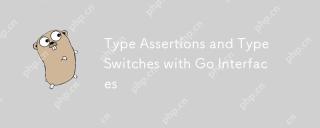
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
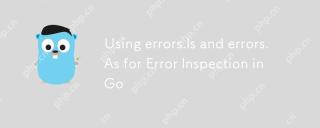
Go language error handling becomes more flexible and readable through errors.Is and errors.As functions. 1.errors.Is is used to check whether the error is the same as the specified error and is suitable for the processing of the error chain. 2.errors.As can not only check the error type, but also convert the error to a specific type, which is convenient for extracting error information. Using these functions can simplify error handling logic, but pay attention to the correct delivery of error chains and avoid excessive dependence to prevent code complexity.
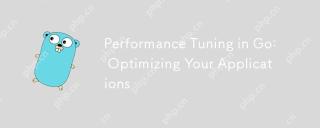
TomakeGoapplicationsrunfasterandmoreefficiently,useprofilingtools,leverageconcurrency,andmanagememoryeffectively.1)UsepprofforCPUandmemoryprofilingtoidentifybottlenecks.2)Utilizegoroutinesandchannelstoparallelizetasksandimproveperformance.3)Implement


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
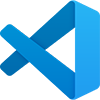
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
