The following article provides an outline of Deserialization in C#. Let us first see the process of serialization. Serialization is the process of converting an object into a form so that it can be written in a stream and stored in memory, a database, or a file. Its main purpose is to store the state of the object.
Now, deserialization is the process opposite of serialization. It is the process of reading or converting the byte stream back to the object so that it can be loaded into the memory. This process enables us to reconstruct an object whenever required.
Syntax with Explanation:
The syntax for deserialization using BinaryFormatter is as follows:
FileStream fileStream = new FileStream(filePath, FileMode.Open); BinaryFormatter binaryFormatter = new BinaryFormatter(); ClassName objectName = (ClassName)binaryformatter.Deserialize(fileStream);
In the above syntax, first, we created an object of FileStream (fileStream) by giving the path of the file (filePath) from where we will get the information to reconstruct the object. After this, we created an object of BinaryFormatter. BinaryFormatter is a class present under System.Runtime.Serialization.Formatters.Binary namespace and is used to serialize and deserialize an object. Then, we deserialized the object using Deserialize() method of BinaryFormatter, which takes an object of FileStream as input and returns an object which we converted to the object of type ClassName and then stored it in objectName.
How does Deserialization Work in C#?
For deserialization in C#, we need to first import System.IO namespace in our code to open the file containing data that will be used to reconstruct the object. Then we need to import System.Runtime.Serialization.Formatters.Binary namespace to work with BinaryFormatter class which will be responsible for serializing and deserializing the object.
Let’s say we have a class called ‘Student’ with two properties i.e., ‘Name’ and ‘RollNo’. We will write the class ‘Student’ data properties to a file using the serialization process. Then by calling the Deserialize() method of BinaryFormatter class, we can read data from that file and reconstruct the object of class ‘Student’, which we call deserialization of the object.
The steps followed to deserialize an object in C# using BinaryFormatter are as follows:
- First, we must create a stream object to read the serialized information or data.
- Then, we will create an object of the class BinaryFormatter.
- After that, we will call the Deserialize() method of BinaryFormatter class to deserialize the object. This method will return an object we can cast to the appropriate type.
In C#, there are three types of serialization:
- Binary serialization
- XML serialization
- JSON serialization
Thus, according to the serialization done, we can deserialize objects in three ways. For binary serialization and deserialization, we use BinaryFormatter class as discussed above; for XML serialization and deserialization, we use XMLSerializer class; and for JSON serialization and deserialization, we use JsonSerializer class.
Pictorial representation of serialization and deserialization in C#:
Examples of Deserialization in C#
Given below are the examples:
Example #1
Example showing binary serialization and deserialization.
Code:
using System; using System.IO; using System.Runtime.Serialization.Formatters.Binary; namespace ConsoleApp4 { class Program { public static void SerializingData() { string str = "Hello world!"; FileStream fileStream = new FileStream(@"E:\Content\content.txt", FileMode.Create); BinaryFormatter binaryFormatter = new BinaryFormatter(); binaryFormatter.Serialize(fileStream, str); fileStream.Close(); } public static void DeserializingData() { FileStream fileStream = new FileStream(@"E:\Content\content.txt", FileMode.Open); BinaryFormatter binaryFormatter = new BinaryFormatter(); string content = ""; content = (string)binaryFormatter.Deserialize(fileStream); fileStream.Close(); Console.WriteLine("Deserialized data: "); Console.WriteLine(content); } static void Main(string[] args) { SerializingData(); DeserializingData(); Console.ReadLine(); } } }<strong> </strong>
Output:
Example #2
Example showing binary serialization and deserialization of custom class.
Code:
using System; using System.IO; using System.Runtime.Serialization.Formatters.Binary; namespace ConsoleApp4 { [Serializable] public class Student { public int RollNo; public string Name; public string Address; public Student(int rollNo, string name, string address) { RollNo = rollNo; Name = name; Address = address; } } public class Program { public static void SerializingData() { Student student = new Student(1, "Sneha", "Kasarwadi, Pune"); //creating file to store data FileStream fileStream = new FileStream(@"E:\Content\Student.txt", FileMode.Create); BinaryFormatter binaryFormatter = new BinaryFormatter(); //serializing data using Serialize() method binaryFormatter.Serialize(fileStream, student); fileStream.Close(); } public static void DeserializingData() { Student student; //opening file to read data FileStream fileStream = new FileStream(@"E:\Content\Student.txt", FileMode.Open); BinaryFormatter binaryFormatter = new BinaryFormatter(); //creating object to store deserialized data student = (Student)binaryFormatter.Deserialize(fileStream); int rollNo = student.RollNo; string name = student.Name; string address = student.Address; fileStream.Close(); //displaying deserialized data Console.WriteLine("Deserialized data: "); Console.WriteLine("Roll No = " + rollNo); Console.WriteLine("Student Name = " + name); Console.WriteLine("Student Address = " + address); } public static void Main(string[] args) { SerializingData(); DeserializingData(); Console.ReadLine(); } } }
Output:
Example #3
Example showing XML serialization and deserialization of custom class.
Code:
using System; using System.IO; using System.Xml.Serialization; namespace ConsoleApp4 { [Serializable] public class Student { public int RollNo { get; set; } public string Name { get; set; } public string Address { get; set; } public Student() { RollNo = 0; Name = "N/A"; Address = "N/A"; } } public class Program { public static void SerializingData(Student student) { //creating file to store data. FileStream fileStream = new FileStream(@"E:\Content\Student.txt", FileMode.Create); XmlSerializer xmlSerializer = new XmlSerializer(typeof(Student)); //calling serialize() method to serialize data to file xmlSerializer.Serialize(fileStream, student); fileStream.Close(); } public static void DeserializingData() { //opening file to read data FileStream fileStream = new FileStream(@"E:\Content\Student.txt", FileMode.Open); XmlSerializer xmlSerializer = new XmlSerializer(typeof(Student)) //calling Deserialize() to deserialize data from the file Student student = (Student)xmlSerializer.Deserialize(fileStream); fileStream.Close(); Console.WriteLine("Deserialized data: "); Console.WriteLine("Student Roll No = " + student.RollNo); Console.WriteLine("Student Name = " + student.Name); Console.WriteLine("Student Address = " + student.Address); } public static void Main(string[] args) { Student student = new Student(); Console.WriteLine("Enter student Roll No"); student.RollNo = Convert.ToInt32(Console.ReadLine()); Console.WriteLine("Enter student name"); student.Name = Console.ReadLine(); Console.WriteLine("Enter student address"); student.Address = Console.ReadLine(); SerializingData(student); DeserializingData(); Console.ReadLine(); } } }
Output:
Conclusion
Deserialization is the process of reconstructing an object from a previously serialized sequence of bytes. It allows us to recover the object whenever it is required. It is the reverse process of serialization. Deserialize() method of BinaryFormatter class is used for deserialization from a binary stream.
The above is the detailed content of Deserialization in C#. For more information, please follow other related articles on the PHP Chinese website!
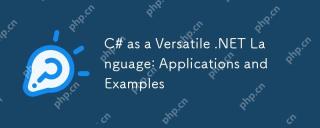
C# is widely used in enterprise-level applications, game development, mobile applications and web development. 1) In enterprise-level applications, C# is often used for ASP.NETCore to develop WebAPI. 2) In game development, C# is combined with the Unity engine to realize role control and other functions. 3) C# supports polymorphism and asynchronous programming to improve code flexibility and application performance.
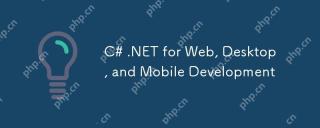
C# and .NET are suitable for web, desktop and mobile development. 1) In web development, ASP.NETCore supports cross-platform development. 2) Desktop development uses WPF and WinForms, which are suitable for different needs. 3) Mobile development realizes cross-platform applications through Xamarin.
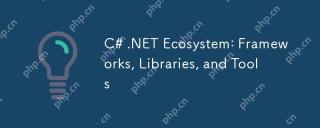
The C#.NET ecosystem provides rich frameworks and libraries to help developers build applications efficiently. 1.ASP.NETCore is used to build high-performance web applications, 2.EntityFrameworkCore is used for database operations. By understanding the use and best practices of these tools, developers can improve the quality and performance of their applications.

How to deploy a C# .NET app to Azure or AWS? The answer is to use AzureAppService and AWSElasticBeanstalk. 1. On Azure, automate deployment using AzureAppService and AzurePipelines. 2. On AWS, use Amazon ElasticBeanstalk and AWSLambda to implement deployment and serverless compute.
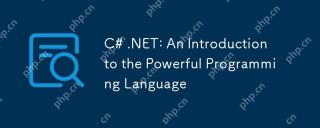
The combination of C# and .NET provides developers with a powerful programming environment. 1) C# supports polymorphism and asynchronous programming, 2) .NET provides cross-platform capabilities and concurrent processing mechanisms, which makes them widely used in desktop, web and mobile application development.
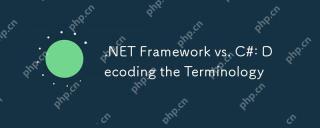
.NETFramework is a software framework, and C# is a programming language. 1..NETFramework provides libraries and services, supporting desktop, web and mobile application development. 2.C# is designed for .NETFramework and supports modern programming functions. 3..NETFramework manages code execution through CLR, and the C# code is compiled into IL and runs by CLR. 4. Use .NETFramework to quickly develop applications, and C# provides advanced functions such as LINQ. 5. Common errors include type conversion and asynchronous programming deadlocks. VisualStudio tools are required for debugging.
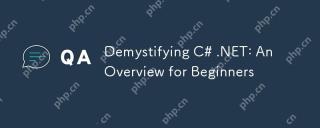
C# is a modern, object-oriented programming language developed by Microsoft, and .NET is a development framework provided by Microsoft. C# combines the performance of C and the simplicity of Java, and is suitable for building various applications. The .NET framework supports multiple languages, provides garbage collection mechanisms, and simplifies memory management.
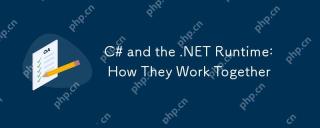
C# and .NET runtime work closely together to empower developers to efficient, powerful and cross-platform development capabilities. 1) C# is a type-safe and object-oriented programming language designed to integrate seamlessly with the .NET framework. 2) The .NET runtime manages the execution of C# code, provides garbage collection, type safety and other services, and ensures efficient and cross-platform operation.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
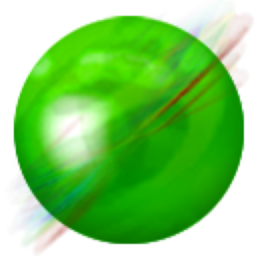
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
