How to delete files in C++? Use the remove function to delete files, its prototype is int remove(const char* filename); use std::filesystem::remove function to delete files, its prototype is std::error_code remove(const std::filesystem::path& path);
How to delete files in C++
C++ provides various functions and methods to delete files. In this article, we will introduce how to use the remove
function and the std::filesystem::remove
function to delete files, and provide a practical case.
remove
Function
remove
function is a function defined in the C++ standard library and is used to delete files. Its prototype is as follows:
int remove(const char* filename);
where:
-
filename
is the path of the file to be deleted.
remove
The function returns an integer indicating the status of the operation:
-
0
indicates successful deletion. - A non-0 value indicates deletion failure.
Practical case: Use the remove
function to delete files
The following is a example of using the remove
function to delete files Code example:
#include <cstdio> int main() { // 要删除的文件路径 const char* filename = "test.txt"; // 删除文件 int result = remove(filename); // 检查操作结果 if (result == 0) { printf("文件 %s 删除成功!\n", filename); } else { printf("文件 %s 删除失败,错误码:%d\n", filename, result); } return 0; }
std::filesystem::remove
Function
Introduced in the C++ standard library of C++17 and later versions Added the std::filesystem
header file, which provides a more modern and high-level API for file system operations. We can use the std::filesystem::remove
function to delete files. Its prototype is as follows:
std::error_code remove(const std::filesystem::path& path);
where:
-
path
is the path of the file to be deleted.
std::filesystem::remove
The function returns a std::error_code
object representing the status of the operation. We can use the std::error_code::value()
method to get the error code.
Practical case: Use std::filesystem::remove
function to delete files
The following is a usestd::filesystem: Code example for :remove
function to delete files:
#include <filesystem> int main() { // 要删除的文件路径 std::filesystem::path filename = "test.txt"; // 删除文件 std::error_code ec; std::filesystem::remove(filename, ec); // 检查操作结果 if (!ec) { std::cout << "文件 " << filename << " 删除成功!" << std::endl; } else { std::cout << "文件 " << filename << " 删除失败,错误码:" << ec.value() << std::endl; } return 0; }
The above is the detailed content of How to delete a file using C++?. For more information, please follow other related articles on the PHP Chinese website!
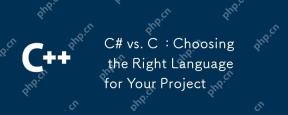
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
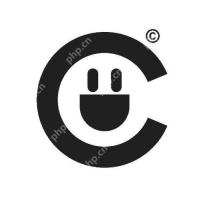
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
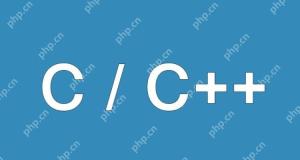
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
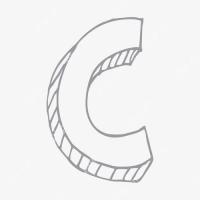
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.
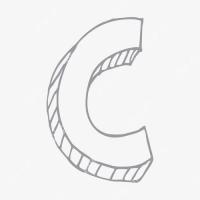
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
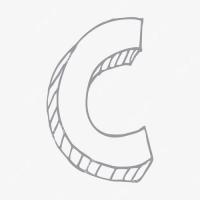
C performs well in real-time operating system (RTOS) programming, providing efficient execution efficiency and precise time management. 1) C Meet the needs of RTOS through direct operation of hardware resources and efficient memory management. 2) Using object-oriented features, C can design a flexible task scheduling system. 3) C supports efficient interrupt processing, but dynamic memory allocation and exception processing must be avoided to ensure real-time. 4) Template programming and inline functions help in performance optimization. 5) In practical applications, C can be used to implement an efficient logging system.
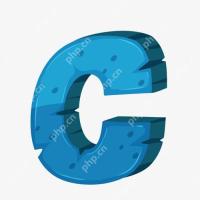
ABI compatibility in C refers to whether binary code generated by different compilers or versions can be compatible without recompilation. 1. Function calling conventions, 2. Name modification, 3. Virtual function table layout, 4. Structure and class layout are the main aspects involved.
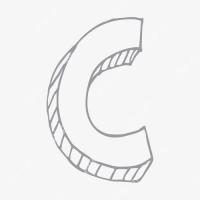
DMA in C refers to DirectMemoryAccess, a direct memory access technology, allowing hardware devices to directly transmit data to memory without CPU intervention. 1) DMA operation is highly dependent on hardware devices and drivers, and the implementation method varies from system to system. 2) Direct access to memory may bring security risks, and the correctness and security of the code must be ensured. 3) DMA can improve performance, but improper use may lead to degradation of system performance. Through practice and learning, we can master the skills of using DMA and maximize its effectiveness in scenarios such as high-speed data transmission and real-time signal processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
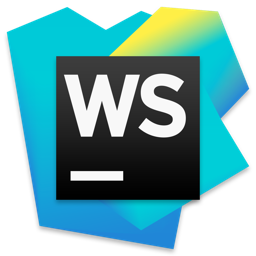
WebStorm Mac version
Useful JavaScript development tools
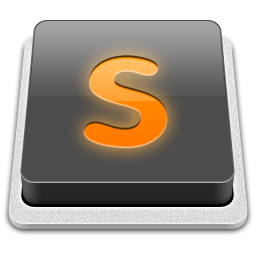
SublimeText3 Mac version
God-level code editing software (SublimeText3)
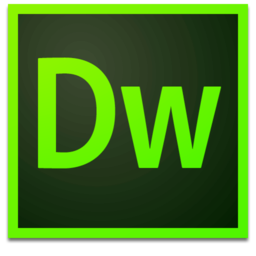
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
