Common types of memory leaks in C++ are: dangling pointers, resource leaks, wild pointers and memory growth. Dangling pointers refer to pointers to freed memory; resource leaks refer to allocated system resources that are not released; wild pointers refer to pointers to uninitialized memory; memory growth is due to gradual, uncontrollable memory accumulation. In practice, even simple classes can cause dangling pointer leaks if not carefully managed.
Common types of memory leaks in C++
1. Dangling Pointers
A dangling pointer refers to a pointer to memory that has been released or destroyed. This leak occurs when there are still pointers referencing the memory pointed to by the pointer after it is freed. For example:
int* ptr = new int; // 分配内存 delete ptr; // 释放内存 *ptr = 10; // 悬垂指针访问已释放的内存
2. Resource Leaks
Resource leaks occur when allocated system resources (such as files, network connections, or database connections) are no longer available When not released when needed. This renders the resources unavailable for other purposes and may eventually cause the system to crash. For example:
FILE* file = fopen("test.txt", "w"); // 打开文件 // ... 对文件进行操作 ... fclose(file); // 文件打开后应立即关闭
3. Wild Pointers
A wild pointer refers to a pointer to uninitialized memory. It may point to any memory address, causing unpredictable behavior, including memory leaks. For example:
int* ptr; // 未经初始化的指针 *ptr = 10; // 野指针访问未定义的内存
4. Memory Bloat
Memory Bloat is a progressive leak in which memory slowly accumulates in an uncontrollable manner. It can be caused by a small leak or by not freeing a temporarily allocated memory block. For example:
for (int i = 0; i < 1000000; i++) { // 为每个迭代分配一个新对象 new Object; }
Practical case
Consider the following code:
class MyClass { public: MyClass() { ptr = new int; } ~MyClass() { delete ptr; } int* ptr; }; int main() { MyClass* obj = new MyClass; delete obj; // 内存泄漏,ptr 指向已释放的内存 return 0; }
In this example, after the object obj
is destroyed, ptr
points to memory that has been freed, causing a dangling pointer leak.
To prevent memory leaks, it is important to follow these best practices:
- Always free allocated memory.
- Automatically manage resources using the RAII (Resource Acquisition Is Initialization) paradigm.
- Use smart pointers or reference counting mechanisms to track allocated memory.
The above is the detailed content of What are the common types of C++ memory leaks?. For more information, please follow other related articles on the PHP Chinese website!
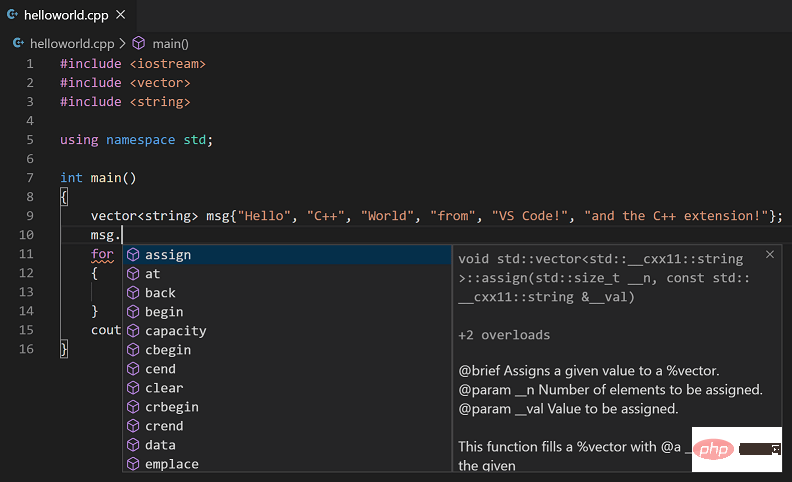
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
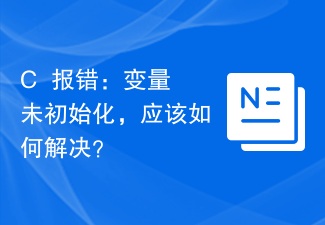
在C++程序开发中,当我们声明了一个变量但是没有对其进行初始化,就会出现“变量未初始化”的报错。这种报错经常会让人感到很困惑和无从下手,因为这种错误并不像其他常见的语法错误那样具体,也不会给出特定的代码行数或者错误类型。因此,下面我们将详细介绍变量未初始化的问题,以及如何解决这个报错。一、什么是变量未初始化错误?变量未初始化是指在程序中声明了一个变量但是没有
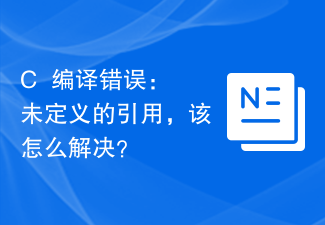
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
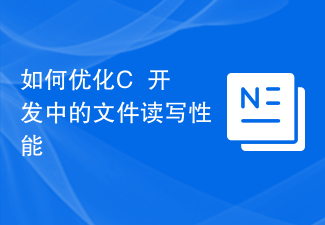
如何优化C++开发中的文件读写性能在C++开发过程中,文件的读写操作是常见的任务之一。然而,由于文件读写是磁盘IO操作,相对于内存IO操作来说会更为耗时。为了提高程序的性能,我们需要优化文件读写操作。本文将介绍一些常见的优化技巧和建议,帮助开发者在C++文件读写过程中提高性能。使用合适的文件读写方式在C++中,文件读写可以通过多种方式实现,如C风格的文件IO
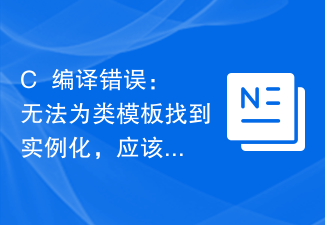
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
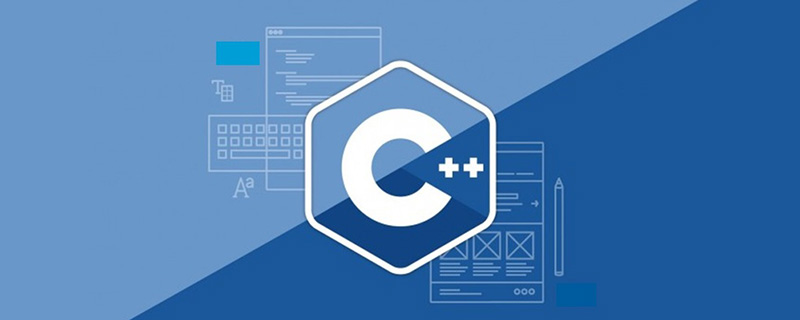
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
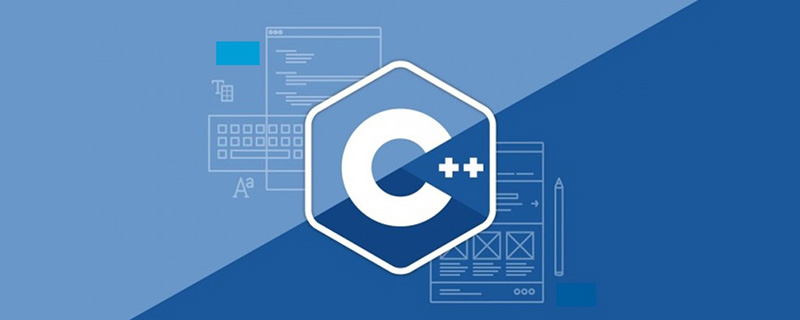
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
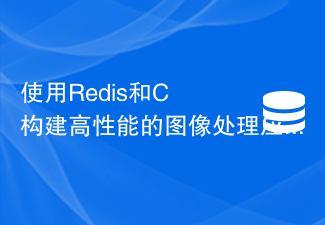
使用Redis和C++构建高性能的图像处理应用图像处理是现代计算机应用中的重要环节之一。由于图像处理的复杂性和计算量大,如何在保证高性能的同时提供稳定的服务是一个挑战。本文将介绍如何使用Redis和C++构建高性能的图像处理应用,并提供一些代码示例。Redis是一个开源的内存数据库,具有高性能和高可用性的特点。它支持各种数据结构,如字符串、哈希表、列表等,同


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
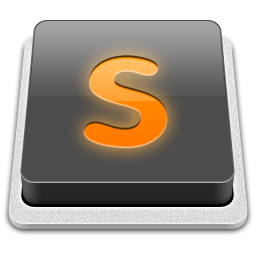
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
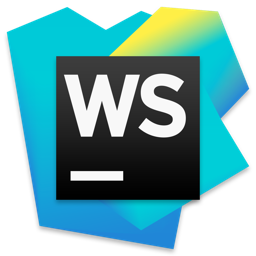
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
