What are the causes of deadlocks in C++ multi-threaded programming?
In C++ multi-threaded programming, the main causes of deadlock are: 1. Improper use of mutex locks; 2. Sequential locking. In actual combat, if multiple threads try to acquire the same set of locks at the same time and acquire them in different orders, deadlock may occur. This can be avoided by always acquiring locks in the same order.
Causes of deadlock in C++ multi-threaded programming
Deadlock is a common error in concurrent programming. Occurs when one or more threads are waiting for another thread to release the lock, and the other thread is waiting for the former to release the lock. This will cause the program to become stuck and unable to continue execution.
In C++, deadlocks are usually caused by the following reasons:
- Improper use of mutex locks: If mutex locks are not used correctly, threads may Will try to acquire the same lock at the same time, resulting in deadlock.
- Sequential locking: If threads need to acquire multiple locks, they should always acquire these locks in the same order. Otherwise, a deadlock may result because one thread may be waiting for another thread to release a lock, and another thread is waiting for that thread to release another lock.
Practical case:
Consider the following code:
class BankAccount { public: std::mutex m_mutex; // 互斥锁 int balance = 0; }; void transfer(BankAccount &from, BankAccount &to, int amount) { std::lock_guard<std::mutex> lock1(from.m_mutex); // 锁定第一个账户 std::lock_guard<std::mutex> lock2(to.m_mutex); // 锁定第二个账户 // 从第一个账户扣除金额 from.balance -= amount; // 将金额添加到第二个账户 to.balance += amount; }
In this example, if two threads call transfer at the same time ()
function and they try to transfer money from different accounts to the same account, a deadlock occurs. This is because one thread will first lock the first account and then wait for another thread to release the second account, and the other thread will first lock the second account and then wait for the first thread to release the first account.
To avoid this, threads should always acquire locks in the same order, for example:
void transfer(BankAccount &from, BankAccount &to, int amount) { // 按照账户 ID 排序账户 if (from.getId() < to.getId()) { std::lock_guard<std::mutex> lock1(from.m_mutex); std::lock_guard<std::mutex> lock2(to.m_mutex); } else { std::lock_guard<std::mutex> lock2(to.m_mutex); std::lock_guard<std::mutex> lock1(from.m_mutex); } // 从第一个账户扣除金额 from.balance -= amount; // 将金额添加到第二个账户 to.balance += amount; }
By sorting accounts by account ID and locking them in the same order, we can prevent this situation occurs.
The above is the detailed content of What are the causes of deadlocks in C++ multi-threaded programming?. For more information, please follow other related articles on the PHP Chinese website!
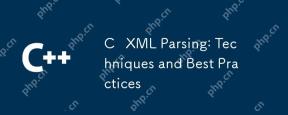
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
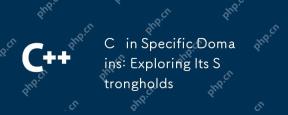
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
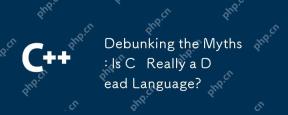
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
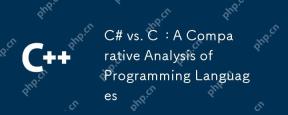
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
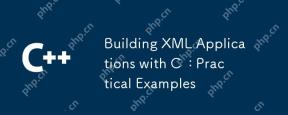
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
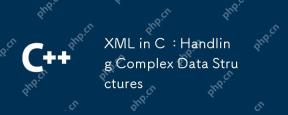
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
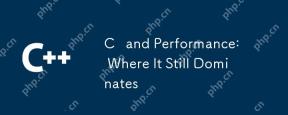
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
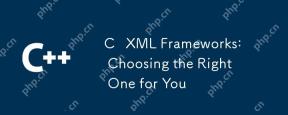
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor
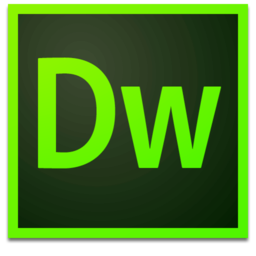
Dreamweaver Mac version
Visual web development tools
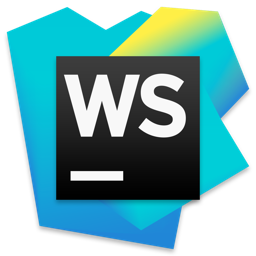
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
