


Concurrent programming is implemented in Go through goroutine, allowing multiple tasks to be executed at the same time to improve efficiency. Its use cases include: parallel processing event processing I/O intensive operations HTTP service task scheduling
Detailed explanation of use cases and scenarios of Go concurrent programming
Introduction
Concurrent programming is a programming paradigm that allows us to perform multiple tasks at the same time. In the Go language, concurrent programming is implemented through goroutines, which are lightweight threads. This article will explore the use cases and scenarios of concurrent programming in Go and provide practical examples.
Use cases and scenarios
1. Parallel processing
- Decompose large tasks into smaller subtasks and process them in parallel for greater efficiency.
- Example: Use Goroutines to paralleize image processing tasks.
2. Event processing
- #Listen to incoming events and use goroutine to process each event in parallel.
- Example: Use Goroutines to handle incoming messages from WebSocket connections.
3. I/O-intensive operations
- For I/O-intensive operations, such as file reading or network calls, use Goroutines Can improve performance.
- Example: Use Goroutines to read data from multiple files in parallel.
4. HTTP service
- In HTTP service, using Goroutines to handle incoming requests can improve concurrency.
- Example: Use Goroutines to handle incoming HTTP requests from a web server.
5. Task Scheduling
- Use Goroutines to manage and schedule tasks that need to be executed at a specific time or periodically.
- Example: Use Goroutine to implement Cron timer to schedule jobs.
Practical example
Example 1: Concurrent image processing
package main import ( "fmt" "image" "image/color" "image/draw" "runtime" ) func main() { width, height := 1000, 1000 images := []image.Image{} // 并行创建 100 个图像 for i := 0; i < 100; i++ { img := image.NewRGBA(image.Rect(0, 0, width, height)) draw.Draw(img, img.Bounds(), &image.Uniform{color.RGBA{0, 0, 0, 255}}, image.ZP, draw.Src) images = append(images, img) } // 计算创建图像所花费的时间 numCPUs := runtime.NumCPU() start := time.Now() for i := 0; i < 100; i++ { go createImage(images[i]) } // 等待所有 Goroutine 完成 time.Sleep(10 * time.Second) elapsed := time.Since(start) fmt.Printf("Creating %d images using %d CPUs took %s\n", len(images), numCPUs, elapsed) } func createImage(img image.Image) { // 模拟耗时的图像处理操作 time.Sleep(500 * time.Millisecond) }
Example 2: Processing WebSocket Message
package main import ( "errors" "fmt" "net/http" "sync/atomic" "github.com/gorilla/websocket" ) type client struct { conn *websocket.Conn name string } var ( upgrader = websocket.Upgrader{} messages = make(chan string) ) var connectedClients uint64 func main() { http.HandleFunc("/websocket", serveWebSocket) // 启动 Goroutine 来处理传入消息 go handleMessage() if err := http.ListenAndServe(":8080", nil); err != nil { fmt.Println(err) } } func serveWebSocket(w http.ResponseWriter, r *http.Request) { conn, err := upgrader.Upgrade(w, r, nil) if err != nil { fmt.Println(err) return } atomic.AddUint64(&connectedClients, 1) go handleConnection(conn) } func handleConnection(conn *websocket.Conn) { defer func() { conn.Close() atomic.AddUint64(&connectedClients, -1) }() // 监听来自客户端的消息 for { _, message, err := conn.ReadMessage() if err != nil { if websocket.IsUnexpectedCloseError(err, websocket.CloseGoingAway, websocket.CloseAbnormalClosure) { fmt.Println(err) } return } messages <- message } } func handleMessage() { for message := range messages { // 处理消息逻辑 fmt.Println("Received message:", message) // 例如,将消息广播给所有已连接的客户端 for clients.Range(func(_, v interface{}) bool { client := v.(client) if err := client.conn.WriteMessage(websocket.TextMessage, []byte(message)); err != nil { if errors.Is(err, websocket.ErrCloseSent) { clients.Delete(client.name) fmt.Printf("Client %s disconnected\n", client.name) } } return true }) { } } }
The above is the detailed content of Detailed explanation of use cases and scenarios of Go concurrent programming. For more information, please follow other related articles on the PHP Chinese website!
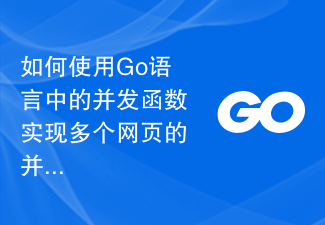
如何使用Go语言中的并发函数实现多个网页的并行抓取?在现代Web开发中,经常需要从多个网页中抓取数据。一般的做法是逐个发起网络请求并等待响应,这样效率较低。而Go语言提供了强大的并发功能,可以通过并行抓取多个网页来提高效率。本文将介绍如何使用Go语言的并发函数实现多个网页的并行抓取,以及一些注意事项。首先,我们需要使用Go语言内置的go关键字创建并发任务。通
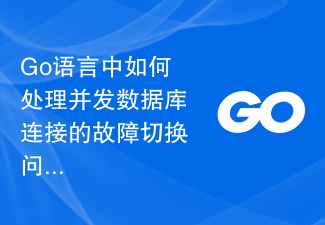
Go语言中如何处理并发数据库连接的故障切换问题?在处理并发数据库连接时,我们通常会遇到数据库连接的故障切换问题。当一个数据库连接发生故障时,我们需要考虑如何及时切换到一个可用的数据库连接,以确保系统的正常运行。下面将详细介绍在Go语言中如何处理并发数据库连接的故障切换问题,并提供一些具体的代码示例。使用连接池:在Go语言中,我们可以使用连接池来管理数据库连接
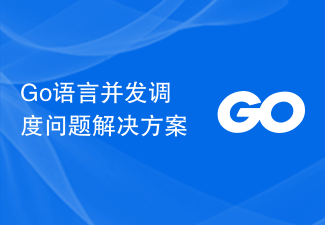
解决Go语言开发中的并发调度问题的方法随着互联网的发展和技术的进步,越来越多的开发者转向了Go语言这种简洁、高效的编程语言。Go语言以其良好的并发性能而闻名,它提供了丰富的并发编程特性,使得开发者可以轻松地实现多任务并发执行。然而,在实际的开发中,我们还是会遇到一些并发调度的问题。本文将介绍一些解决这些问题的方法。Go语言提供了goroutine和chann
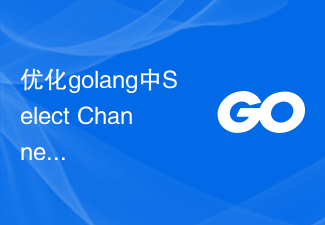
优化golang中SelectChannelsGo并发式编程的性能调优策略引言:随着现代计算机处理器的多核心和并行计算能力的提高,Go语言作为一门并发式编程语言,被广泛采用来开发高并发的后端服务。在Go语言中,使用goroutine和channel可以轻松实现并发编程,提高程序的性能和响应速度。而在并发编程中,使用select语句与channel配合使用
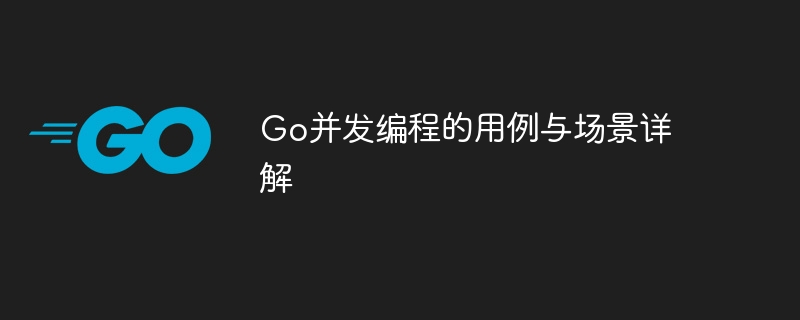
并发编程在Go中通过goroutine实现,允许同时执行多个任务,提高效率。其用例包括:并行处理事件处理I/O密集型操作HTTP服务任务调度
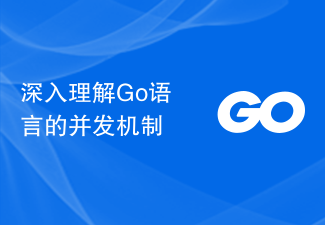
深入理解Go语言的并发机制在Go语言中,通过goroutine和channel实现并发是一种非常高效和简洁的方式。并发是指程序中的多个任务可以同时执行,而不需要等待其它任务结束。通过利用CPU的多核资源,可以提高程序的运行效率。本文将深入探讨Go语言并发机制,并通过具体的代码示例来帮助读者更好地理解。一、goroutine在Go语言中,goroutine是一
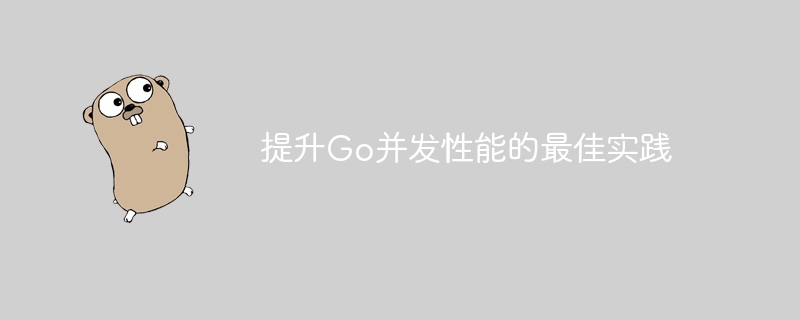
提升Go并发性能的最佳实践:优化Goroutine调度:调整GOMAXPROCS、SetNumGoroutine和SetMaxStack参数以优化性能。使用Channel同步:利用无缓冲和有缓冲channel以安全有效的方式同步协程执行。代码并行化:识别可并行执行的代码块并通过goroutine并行执行它们。减少锁争用:使用读写锁、无锁通信和局部变量以最小化对共享资源的竞争。实战案例:优化图像处理程序的并发性能,通过调整调度器、使用channel和并行处理显著提高了吞吐量。
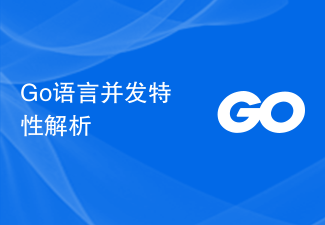
Go语言并发特性解析Go语言作为一种由Google开发的开源编程语言,在处理并发编程方面拥有独特的优势。由于其简洁、高效和强大的并发机制,Go语言越来越受到开发者的青睐。本文将深入探讨Go语言的并发特性,包括goroutine、channel和并发原语,并结合具体的代码示例进行解析。一、goroutine在Go语言中,goroutine是其并发的基本单元,


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
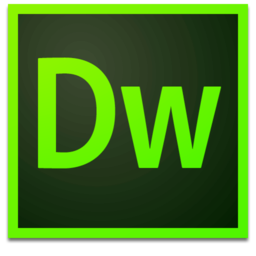
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
