1.轮播图
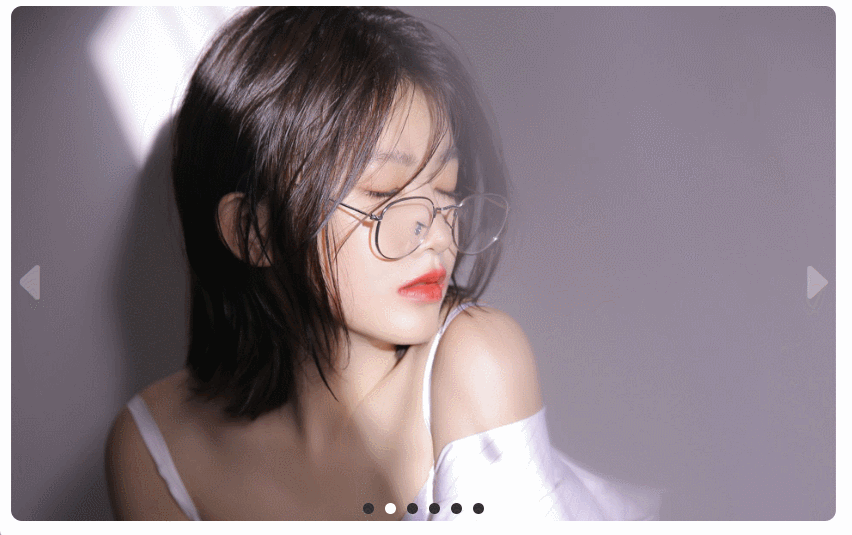
.slider {
max-width: 750px;
min-width: 320px;
margin: auto;
padding: 0 10px;
}
.slider .imgs {
/* 图片容器必须要有高度,否则下面图片不能正常显示 */
max-height: 468px;
}
.slider .imgs img {
/* 图片完全充满父级空间显示 */
width: 100%;
height: 100%;
/* 图片带有圆角 */
border-radius: 10px;
/* 默认图片全部隐藏,只有有active的图片才显示 */
display: none;
}
/* 默认显示第一张 */
.slider .imgs img.active {
display: block;
}
/* 轮播图按钮组 */
.slider .btns {
/* 按钮水平一排显示,用flex,且水平居中 */
display: flex;
place-content: center;
}
.slider .btns span {
/* 鼠标移上去变成小手 */
cursor: pointer;
/* 按钮宽高相同,确定显示成一个正圆 */
width: 10px;
height: 10px;
/* 加上红色背景和数字是为了布局时可以看到,一会更去掉 */
background-color: #333;
/* 50%可确保显示为正圆 */
border-radius: 50%;
/* 按钮上外边距负值,可将它上移,可移动到图片中下方 */
margin: -16px 5px 5px;
}
.slider .btns span.active {
background-color: #fff;
}
.slider .leftRight {
/* 弹性盒子 */
display: flex;
/* 基于剩余空间两端对齐 */
place-content: space-between;
/* 按钮上移 */
margin-top: -234px;
}
.slider .leftRight span {
/* 鼠标移上去变成小手 */
cursor: pointer;
font-size: 35px;
color: rgba(250, 248, 248, 0.3);
}
<div class="slider">
<!-- 图片容器 -->
<div class="imgs"></div>
<!-- 切换按钮数量与图片数量必须一致 -->
<div class="btns"></div>
<div class="leftRight">
<span class="iconfont icon-xiangzuo" onclick="setTurnPage(this)" data-index="1"></span>
<span class="iconfont icon-xiangyou" onclick="setTurnPage(this)" data-index="2"></span>
</div>
</div>
<script>
// 将所有图片放在一个数组中
const imgUrls = [
"images/1.jpeg",
"images/2.jpeg",
"images/3.jpeg",
"images/4.jpeg",
"images/5.jpeg",
"images/6.jpeg",
];
//加载时添加元素的方法 动态添加到页面中
loadElement = () => {
for (let i = 0; i < imgUrls.length; i++) {
document
.querySelector(".imgs")
.insertAdjacentHTML(
"beforeend",
`<a href="#"><img src="${imgUrls[i]}" alt="" data-index="${i + 1}" /></a>`
);
document
.querySelector(".btns")
.insertAdjacentHTML(
"beforeend",
`<span data-index="${i + 1}" onclick="setActive(this)"></span>`
);
}
};
// 页面加载完成后动态添加元素
window.addEventListener("load", loadElement, false);
//声明全局变量
let imgs = "";
let btns = "";
// 因为document.querySelector()执行顺序是在页面加载完成前执行
// 所以需要延迟加载,等动态添加的元素完成后执行默认显示第一项
// 定时器 一次性
setTimeout(() => {
// 默认显示第一项
document.querySelector("img").classList.add("active");
document.querySelector("span").classList.add("active");
// 得到所有图片和按钮
imgs = document.querySelectorAll(".slider .imgs img");
btns = document.querySelectorAll(".slider .btns span");
//定时器 间歇式
setInterval(
(arr) => {
// 从头部取一个
let index = arr.shift();
// 将一个自定义的点击事件,分配给与当前索引对应的按钮上就可以了
btns[index].dispatchEvent(new Event("click"));
// 把头部取出来的,再尾部再追加上去
arr.push(index);
},
2000,
Object.keys(btns)
);
}, 50);
setActive = (ele) => {
// 1. 清空图片和按钮的状态
imgs.forEach((img) => img.classList.remove("active"));
btns.forEach((btn) => btn.classList.remove("active"));
// 2.根据按钮的自定义data-index的值,来确定应该显示哪一张图片
// 激活当前按钮
event.target.classList.add("active");
[...imgs].find((img) =>
img.dataset.index === event.target.dataset.index ? img.classList.add("active") : false
);
};
</script>
2.选项卡
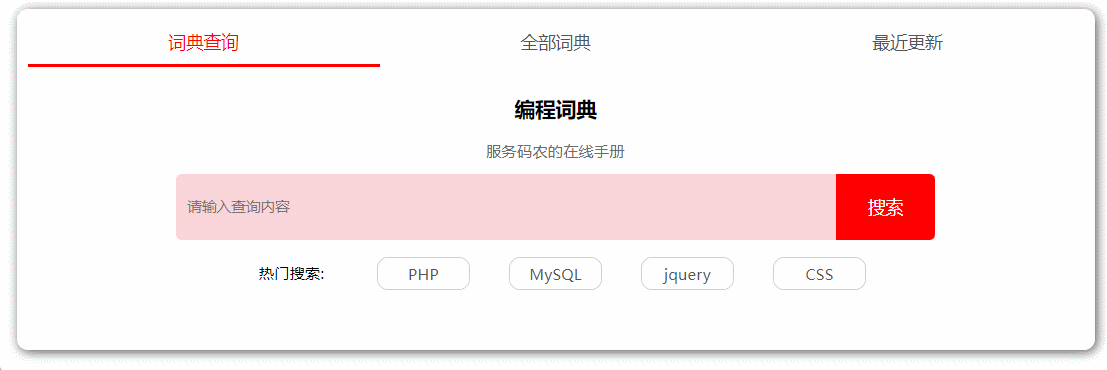
/* 初始化 */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
outline: none;
}
a {
color: #555;
text-decoration: none;
}
li {
list-style: none;
}
.box {
width: 980px;
margin: 10px auto;
border-radius: 10px;
box-shadow: 2px 2px 10px #555;
padding: 10px;
}
.box .menu {
width: 100%;
height: 40px;
display: grid;
grid-template-columns: repeat(3, 1fr);
text-align: center;
line-height: 40px;
}
.box .menu a:hover,
.box .menu a.active {
color: red;
border-bottom: 3px solid red;
}
.box .list {
height: 250px;
padding: 10px;
display: none;
}
.box .list.active {
display: block;
}
.box .list:first-of-type .box1 {
display: grid;
grid-template-rows: 1fr 30px 1fr 1fr;
place-items: center;
}
.box .list:first-of-type .box1 span {
place-self: start center;
font-size: 14px;
color: rgb(104, 103, 103);
height: 30px;
}
.box .list:first-of-type .box1 .serch {
display: flex;
}
.box .list:first-of-type .box1 .serch .serchInput {
width: 600px;
height: 60px;
background-color: rgb(250, 213, 219);
line-height: 60px;
border-radius: 5px 0 0 5px;
padding: 0 10px;
color: rgb(104, 103, 103);
border: none;
}
.box .list:first-of-type .box1 .serch .btn {
width: 90px;
height: 60px;
text-align: center;
color: white;
background-color: red;
line-height: 60px;
border-radius: 0 5px 5px 0;
cursor: pointer;
}
.box .list:first-of-type .box1 .bottom {
width: 600px;
display: flex;
place-content: space-around;
}
.box .list:first-of-type .box1 .bottom a {
width: 85px;
height: 30px;
text-align: center;
border: 1px solid rgb(207, 206, 206);
border-radius: 10px;
font-size: 14px;
line-height: 30px;
}
.box .list:first-of-type .box1 .bottom span {
color: black;
width: 85px;
height: 30px;
text-align: center;
line-height: 30px;
}
.box .list:nth-of-type(2) .box2 {
display: grid;
padding: 20px 0;
grid-template-columns: repeat(4, 200px);
place-content: space-around;
gap: 15px;
}
.box .list:nth-of-type(2) .box2 .item {
height: 100px;
display: grid;
background-color: rgba(219, 217, 217, 0.459);
grid-template-columns: 40px 140px;
padding: 0 5px;
border-radius: 10px;
}
.box .list:nth-of-type(2) .box2 .item a:first-of-type {
place-self: center start;
grid-column: span 2;
}
.box .list:nth-of-type(2) .box2 .item a:nth-of-type(2) img {
width: 40px;
height: 40px;
margin: auto;
border-radius: 40px;
}
.box .list:last-of-type .box3 {
padding: 50px 30px;
display: flex;
flex-wrap: wrap;
gap: 20px;
}
.box .list:last-of-type .box3 a {
padding: 10px;
height: 0 30px;
border: 1px solid rgb(207, 206, 206);
border-radius: 10px;
font-size: 14px;
text-align: center;
}
.box .list:last-of-type .box3 a:hover {
background-color: red;
color: white;
}
<div class="box">
<nav class="menu">
<a href="" class="active" data-index="1">词典查询</a>
<a href="" data-index="2">全部词典</a>
<a href="" data-index="3">最近更新</a>
</nav>
<!-- 2. 内容列表 -->
<ul class="list active" data-index="1">
<div class="box1">
<h3>编程词典</h3>
<span>服务码农的在线手册</span>
<div class="serch">
<input class="serchInput" placeholder="请输入查询内容"></input>
<div class="btn">搜索</div>
</div>
<div class="bottom">
<span>热门搜索:</span>
<a href="#">PHP</a>
<a href="#">MySQL</a>
<a href="#">jquery</a>
<a href="#">CSS</a>
</div>
</div>
</ul>
<ul class="list" data-index="2">
<div class="box2">
<div class="item">
<a href="">【学习php】</a>
<a href=""><img src="images/01.png" alt=""></a>
<a href="">php是一种被广泛应用的开...</a>
</div>
<div class="item">
<a href="">【学习php】</a>
<a href=""><img src="images/01.png" alt=""></a>
<a href="">php是一种被广泛应用的开...</a>
</div> <div class="item">
<a href="">【学习php】</a>
<a href=""><img src="images/01.png" alt=""></a>
<a href="">php是一种被广泛应用的开...</a>
</div> <div class="item">
<a href="">【学习php】</a>
<a href=""><img src="images/01.png" alt=""></a>
<a href="">php是一种被广泛应用的开...</a>
</div> <div class="item">
<a href="">【学习php】</a>
<a href=""><img src="images/01.png" alt=""></a>
<a href="">php是一种被广泛应用的开...</a>
</div> <div class="item">
<a href="">【学习php】</a>
<a href=""><img src="images/01.png" alt=""></a>
<a href="">php是一种被广泛应用的开...</a>
</div> <div class="item">
<a href="">【学习php】</a>
<a href=""><img src="images/01.png" alt=""></a>
<a href="">php是一种被广泛应用的开...</a>
</div> <div class="item">
<a href="">【学习php】</a>
<a href=""><img src="images/01.png" alt=""></a>
<a href="">php是一种被广泛应用的开...</a>
</div>
</div>
</ul>
<ul class="list" data-index="3">
<div class="box3">
<a href="">php arr_diff_assoc()函数</a>
<a href="">js querySelector()方法</a>
<a href="">js preventDefault()方法</a>
<a href="">php arr_diff_assoc()函数</a>
<a href="">length用法</a>
<a href="">php arr_diff_assoc()函数</a>
<a href="">php arr_diff_assoc()函数</a>
<a href="">数组函数</a>
<a href="">js preventDefault()方法</a>
<a href="">php arr_diff_assoc()函数</a>
</div>
</ul>
</div>
show = () => {
// 禁用<a>的默认跳转行为
event.preventDefault();
// 获取事件触发者
const btns = [...event.currentTarget.children];
// 得到所有list模块
const lists = document.querySelectorAll(".list");
// 遍历删除所有active
btns.forEach((item) => item.classList.remove("active"));
lists.forEach((item) => item.classList.remove("active"));
// 给当前事件触发者绑定active
event.target.classList.add("active");
// 遍历 满足list与当前事件触发者的自定义属性相等时 绑定active
[...lists]
.find((list) => list.dataset.index === event.target.dataset.index)
.classList.add("active");
};
// 事件冒泡 得到a元素的父级元素 绑定事件 委托父级操作
const menu = document.querySelector(".menu");
// 鼠标悬浮
menu.addEventListener("mouseover", show, false);
3.数组API
let arr1 = [1, 2, 3, 4, 5];
let arr2 = ["a", "a", "b", "b"];
// 1.concat(): 合并数组
console.log("合并数组: ", arr1.concat(arr2));
// 2.join(): 将数组的元素按指定符号连接,组成一个新的字符串
console.log("连接数组内元素: ", arr1.join("-"));
// 3.reverse(): 数组反序
console.log("数组反序: ", arr1.reverse());
// 4.valueOf(): 数组的默认方法
console.log("数组的默认方法: ", arr2.valueOf());
// 5.toString(): 将数组转为字符串
console.log("将数组转为字符串: ", arr2.toString());
// 6.isArray(): 判读是否为数组
console.log("判读是否为数组: ", Array.isArray(arr2));
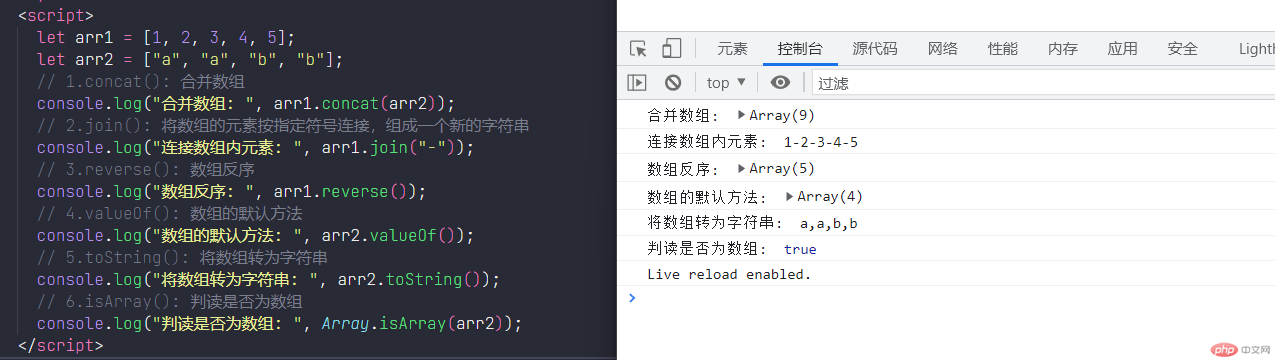