轮播图演示代码
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>图片轮播</title>
<style>
.lb-box {
width: 98%;
margin: auto;
position: relative;
overflow: hidden;
}
@media (max-width: 568px) {
.lb-box {
width: 98%;
}
}
.lb-content {
width: 100%;
height: 100%;
}
.lb-item {
width: 100%;
height: 100%;
display: none;
position: relative;
}
.lb-item > a {
width: 100%;
height: 100%;
display: block;
}
.lb-item > a > img {
width: 100%;
height: 100%;
}
.lb-item > a > span {
width: 100%;
display: block;
position: absolute;
bottom: 0px;
padding: 15px;
color: #fff;
background-color: rgba(0, 0, 0, 0.7);
}
@media (max-width: 568px) {
.lb-item > a > span {
padding: 10px;
}
}
.lb-item.active {
display: block;
left: 0%;
}
.lb-item.active.left {
left: -100%;
}
.lb-item.active.right {
left: 100%;
}
/* */
.lb-item.next,
.lb-item.prev {
display: block;
position: absolute;
top: 0px;
}
.lb-item.next {
left: 100%;
}
.lb-item.prev {
left: -100%;
}
.lb-item.next.left,
.lb-item.prev.right {
left: 0%;
}
.lb-sign {
position: absolute;
bottom: 0;
left: 35%;
padding: 5px 3px;
border-radius: 6px;
list-style: none;
user-select: none;
background-color: rgba(0, 0, 0, 0.7);
}
.lb-sign li {
width: 22px;
height: 20px;
font-size: 14px;
font-weight: 500;
line-height: 20px;
text-align: center;
float: left;
color: #aaa;
margin: auto 4px;
border-radius: 3px;
cursor: pointer;
}
.lb-sign li:hover {
color: #fff;
}
.lb-sign li.active {
color: #000;
background-color: #ebebeb;
}
.lb-ctrl {
position: absolute;
top: 50%;
transform: translateY(-50%);
font-size: 50px;
font-weight: 900;
user-select: none;
background-color: rgba(0, 0, 0, 0.7);
color: #fff;
border-radius: 5px;
cursor: pointer;
transition: all 0.1s linear;
}
@media (max-width: 568px) {
.lb-ctrl {
font-size: 30px;
}
}
.lb-ctrl.left {
left: -50px;
}
.lb-ctrl.right {
right: -50px;
}
.lb-box:hover .lb-ctrl.left {
left: 10px;
}
.lb-box:hover .lb-ctrl.right {
right: 10px;
}
.lb-ctrl:hover {
background-color: #000;
}
</style>
<script>
class Lb {
constructor(options) {
this.lbBox = document.getElementById(options.id);
this.lbItems = this.lbBox.querySelectorAll(".lb-item");
this.lbSigns = this.lbBox.querySelectorAll(".lb-sign li");
this.lbCtrlL = this.lbBox.querySelectorAll(".lb-ctrl")[0];
this.lbCtrlR = this.lbBox.querySelectorAll(".lb-ctrl")[1];
// 当前图片索引
this.curIndex = 0;
// 轮播盒内图片数量
this.numItems = this.lbItems.length;
// 是否可以滑动
this.status = true;
// 轮播速度
this.speed = options.speed || 600;
// 等待延时
this.delay = options.delay || 3000;
// 轮播方向
this.direction = options.direction || "left";
// 是否监听键盘事件
this.moniterKeyEvent = options.moniterKeyEvent || false;
// 是否监听屏幕滑动事件
this.moniterTouchEvent = options.moniterTouchEvent || false;
this.handleEvents();
this.setTransition();
}
// 开始轮播
start() {
const event = {
srcElement: this.direction == "left" ? this.lbCtrlR : this.lbCtrlL,
};
const clickCtrl = this.clickCtrl.bind(this);
// 每隔一段时间模拟点击控件
this.interval = setInterval(clickCtrl, this.delay, event);
}
// 暂停轮播
pause() {
clearInterval(this.interval);
}
/**
* 设置轮播图片的过渡属性
* 在文件头内增加一个样式标签
* 标签内包含轮播图的过渡属性
*/
setTransition() {
const styleElement = document.createElement("style");
document.head.appendChild(styleElement);
const styleRule = `.lb-item {transition: left ${this.speed}ms ease-in-out}`;
styleElement.sheet.insertRule(styleRule, 0);
}
// 处理点击控件事件
clickCtrl(event) {
if (!this.status) return;
this.status = false;
if (event.srcElement == this.lbCtrlR) {
var fromIndex = this.curIndex,
toIndex = (this.curIndex + 1) % this.numItems,
direction = "left";
} else {
var fromIndex = this.curIndex;
(toIndex = (this.curIndex + this.numItems - 1) % this.numItems),
(direction = "right");
}
this.slide(fromIndex, toIndex, direction);
this.curIndex = toIndex;
}
// 处理点击标志事件
clickSign(event) {
if (!this.status) return;
this.status = false;
const fromIndex = this.curIndex;
const toIndex = parseInt(event.srcElement.getAttribute("slide-to"));
const direction = fromIndex < toIndex ? "left" : "right";
this.slide(fromIndex, toIndex, direction);
this.curIndex = toIndex;
}
// 处理各类事件
handleEvents() {
// 鼠标移动到轮播盒上时继续轮播
this.lbBox.addEventListener("mouseleave", this.start.bind(this));
// 鼠标从轮播盒上移开时暂停轮播
this.lbBox.addEventListener("mouseover", this.pause.bind(this));
// 点击左侧控件向右滑动图片
this.lbCtrlL.addEventListener("click", this.clickCtrl.bind(this));
// 点击右侧控件向左滑动图片
this.lbCtrlR.addEventListener("click", this.clickCtrl.bind(this));
// 点击轮播标志后滑动到对应的图片
for (let i = 0; i < this.lbSigns.length; i++) {
this.lbSigns[i].setAttribute("slide-to", i);
this.lbSigns[i].addEventListener(
"click",
this.clickSign.bind(this)
);
}
}
slide(fromIndex, toIndex, direction) {
this.lbSigns[fromIndex].className = "";
this.lbSigns[toIndex].className = "active";
setTimeout(
(() => {
this.lbItems[fromIndex].className = "lb-item";
this.lbItems[toIndex].className = "lb-item active";
this.status = true; // 设置为可以滑动
}).bind(this),
this.speed + 50
);
}
}
window.onload = function () {
// 轮播选项
const options = {
id: "lb-1", // 轮播盒ID
speed: 600, // 轮播速度(ms)
};
const lb = new Lb(options);
lb.start();
};
</script>
</head>
<body>
<div class="lb-box" id="lb-1">
<!-- 轮播内容 -->
<div class="lb-content">
<div class="lb-item active">
<img src="images/t1.png" alt="" style="width: 100%" />
</div>
<div class="lb-item">
<img src="images/t2.png" alt="" style="width: 100%" />
</div>
<div class="lb-item">
<img src="images/t3.png" alt="" style="width: 100%" />
</div>
<div class="lb-item">
<img src="images/t4.png" alt="" style="width: 100%" />
</div>
</div>
<!-- 轮播标志 -->
<ol class="lb-sign">
<li class="active">1</li>
<li>2</li>
<li>3</li>
<li>4</li>
</ol>
<!-- 轮播控件 -->
<div class="lb-ctrl left"><</div>
<div class="lb-ctrl right">></div>
</div>
</body>
</html>
运行效果
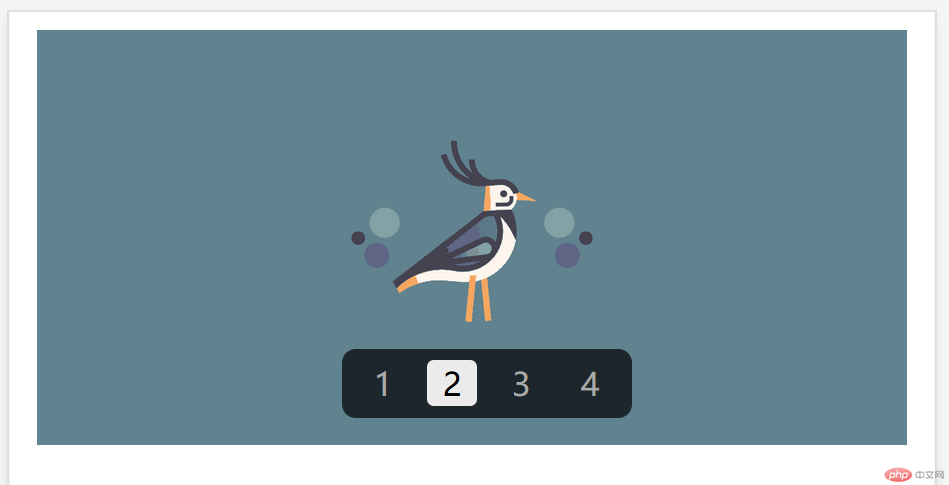
选项卡演示代码
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>选项卡</title>
<style>
* {
margin: 0;
padding: 0;
list-style: none;
}
body {
font-size: 12px;
color: #666;
text-align: left;
}
h1 {
text-align: center;
}
.zzsc {
width: 500px;
height: 200px;
margin: 100px auto;
background: #f0f0f0;
font-family: "微软雅黑";
}
.zzsc .tab {
overflow: hidden;
background: #ccc;
}
.zzsc .tab a {
display: block;
padding: 10px 20px;
float: left;
text-decoration: none;
color: #333;
}
.zzsc .tab a:hover {
background: #e64e3f;
color: #fff;
text-decoration: none;
}
.zzsc .tab a.on {
background: #e64e3f;
color: #fff;
text-decoration: none;
}
.zzsc .list {
display: none;
}
.zzsc .list.on {
display: block;
}
/* .zzsc .list li {
display: none;
} */
</style>
</head>
<body>
<div class="zzsc">
<!-- 标签 -->
<div class="tab">
<a href="" class="on" data-index="1">国际新闻</a>
<a href="" data-index="2">国内新闻</a>
<a href="" data-index="3">社会民生</a>
<a href="" data-index="4">本地新闻</a>
</div>
<!-- 内容列表 -->
<ul class="list on" data-index="1">
<li>1.国际新闻</li>
<li>2.国际新闻</li>
<li>3.国际新闻</li>
<li>4.国际新闻</li>
</ul>
<ul class="list" data-index="2">
<li>1.国内新闻</li>
<li>2.国内新闻</li>
<li>3.国内新闻</li>
<li>4.国内新闻</li>
</ul>
<ul class="list" data-index="3">
<li>1.社会民生</li>
<li>2.社会民生</li>
<li>3.社会民生</li>
<li>4.社会民生</li>
</ul>
<ul class="list" data-index="4">
<li>1.本地新闻</li>
<li>2.本地新闻</li>
<li>3.本地新闻</li>
<li>4.本地新闻</li>
</ul>
</div>
<script>
const tab = document.querySelector(".tab");
// console.log(tab);
tab.addEventListener("click", show, false);
tab.addEventListener("mouseover", show, false);
function show() {
event.preventDefault();
const btns = [...event.currentTarget.children];
// console.log(btns);
const on = document.querySelectorAll(".list");
// console.log(on);
btns.forEach((item) => item.classList.remove("on"));
event.target.classList.add("on");
on.forEach((item) => item.classList.remove("on"));
[...on]
.find((list) => list.dataset.index === event.target.dataset.index)
.classList.add("on");
}
</script>
</body>
</html>
运行效果
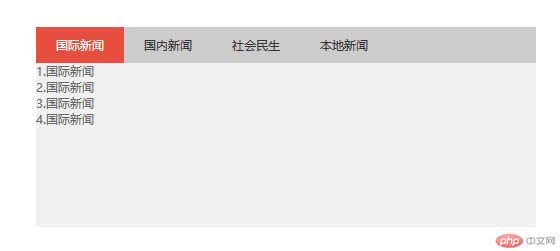