1.html
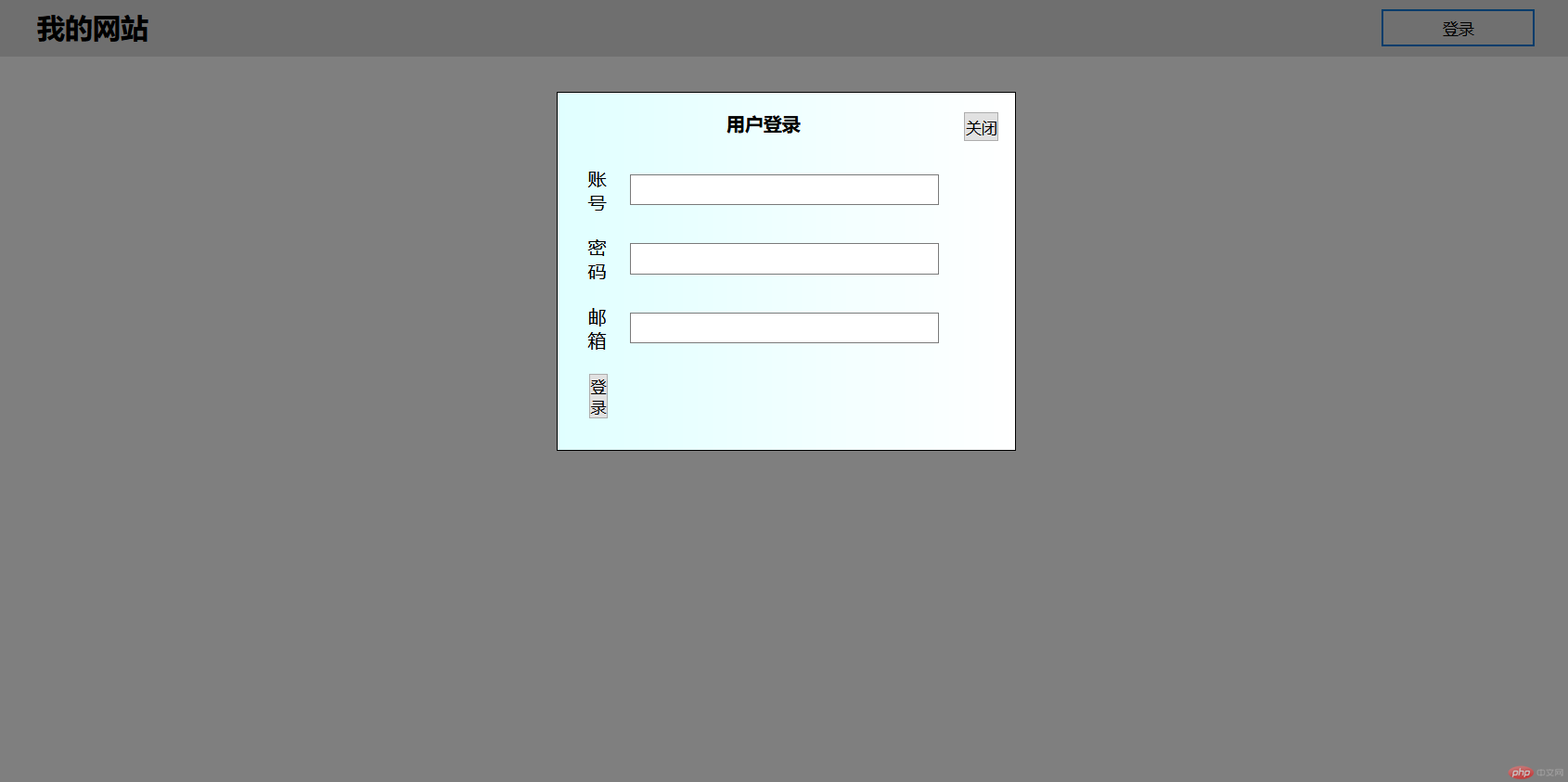
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>固定定位实战:模态框</title>
<link rel="stylesheet" href="modal.css" />
</head>
<body>
<header>
<!-- 页眉 -->
<h2>我的网站</h2>
<button>登录</button>
</header>
<div class="modal">
<!-- 半透明的蒙版遮罩盖住模态框弹层的后面内容 -->
<div class="modal-backdrop"></div>
<!-- 主体 -->
<div class="modal-body">
<!-- 关闭按钮 -->
<button class="close">关闭</button>
<form action="" method="POST">
<table>
<caption>
用户登录
</caption>
<tr>
<td><label for="usernamel">账号</label></td>
<td><input type="username" name="usernamel" id="username" /></td>
</tr>
<tr>
<td><label for="password">密码</label></td>
<td><input type="password" name="password" id="password" /></td>
</tr>
<tr>
<td><label for="email">邮箱</label></td>
<td><input type="email" name="email" id="email" /></td>
</tr>
<tr>
<td><button>登录</button></td>
</tr>
</table>
</form>
</div>
</div>
<script src="modal.js"></script>
</body>
</html>
2 css
/* 初始化 */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
/* 页眉 */
header {
background-color: #dedede;
padding: 0.5em 2em;
overflow: hidden;
}
header h2 {
float: left;
}
header button {
float: right;
width: 10em;
height: 2.5em;
}
header button:hover {
cursor: pointer;
background-color: azure;
}
/* 模态框 */
/* 遮罩 */
.modal .modal-backdrop {
/* 蒙版必须将弹层的后面全部盖住,意味着与容器大小一样 */
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
background-color: rgb(0, 0, 0, 0.5);
}
/* 模态框主体 */
.modal .modal-body {
border: 1px solid #000;
padding: 1em;
min-width: 20em;
background-color: lightcyan;
background: linear-gradient(to right, lightcyan, #fff);
/* 将一个块在另一个块中垂直居中 */
position: fixed;
top: 5em;
left: 30em;
right: 30em;
}
.modal .modal-body form {
width: 80%;
}
.modal .modal-body form caption {
font-weight: bolder;
margin-bottom: 1em;
}
.modal .modal-body form td {
padding: 0.5em;
}
.modal .modal-body form table td:first-of-type {
width: 5em;
}
.modal .modal-body form input {
width: 20em;
height: 2em;
}
.modal {
position: relative;
}
.modal .modal-body .close {
position: absolute;
right: 1em;
width: 4eem;
height: 2em;
}
.modal button:hover {
cursor: pointer;
background-color: azure;
}
/* 当页面刚打开的时候,应该将弹窗隐藏 */
.modal {
display: none;
}
3.js
const btn = document.querySelector("header button");
const modal = document.querySelector(".modal");
const close = document.querySelector(".close");
btn.addEventListener("click", setModal, false);
close.addEventListener("click", setModal, false);
function setModal(ev) {
ev.preventDefault();
let status = window.getComputedStyle(modal, null).getPropertyValue("display");
modal.style.display = status === "none" ? "block" : "none";
}