1102作业
1.数组
- 声明: 字面量, […]
- 索引: [0,1,2,3,..], 从0开始递增的”有序”正整数
- 值: 可以是任何类型
- 索引+值: 数组成员 或 数组元素
1.1数组
const arr = ['苹果', 5, true]
console.log('array: ', arr[0], arr[1], arr[2])
// 判断: 不用typeof, 用 Array.isArray(a)
console.log(typeof arr,Array.isArray(a))
1.2对象
- 声明: 字面量 { … }
- 属性: 语义化字符串, 功能与数组索引类似,但”无序”
- 值: 可以是任何类型
- 属性+值: 对象成员 或 对象元素
- 与数组对比, 对象更像是一个”关联数组”
const item = {
name: '手机',
price: 5000,
'in stock': true,
}
console.log('object: ', item['name'], item['price'], item['in stock'])
// 点语法: 属性名是合法的标识符
// 如果属性名是一个非法的标识符, 必须用引号包装,访问时必须使用数组的语法,不能用点
console.log('object: ', item.name, item.price, item['in stock'])
console.log(typeof item)
1.3多维数组
- forEach: 用于迭代遍历数组或对象
- foreach(callback): 参数是一个函数,当函数当参数时,叫”回调”
- arr.forEach(function(item,key,arr){}), 只有第一个参数item是必选
const arr1 = [
[1, '西瓜', 10],
[2, '苹果', 20],
[3, '黄桃', 30],
]
// arr1.forEach(function (item, key, arr1) {
// console.log(item, key, arr1)
// })
// arr1.forEach(function (item) {
// console.log(item)
// })
// 箭头函数
arr1.forEach(item => console.log(item))
2. 对象数组
// 成员是一个对象字面量, 前后端分离开发中, 服务器返回JSON
const fruits = [
{ id: 1, name: '西瓜', price: 10 },
{ id: 2, name: '苹果', price: 20 },
{ id: 3, name: '黄桃', price: 30 },
]
fruits.forEach(item => console.log(item))
3. 类数组
// 不是 class, 类: 类似,像, 类数组->类似一个数组,但不是数组
// 仍然是一个对象, 用对象来模拟一个数组
// dom编程, 浏览器中的对象
const likeArr = {
0: 'admin',
1: 'admin@qq.com',
2: '498668472',
length: 3,
}
/**
* ? 类数组特征
* * 1. 由0开始的递增的正整数的索引/属性
* * 2. 必须要有 `length`,表示成员数量/数组长度
*/
// likeArr.forEach(item => console.log(item))
// 将类数组转为真正的数组
console.log(Array.from(likeArr))
// [('admin', 'admin@qq.com', '498668472')]
// Array.form: 类数组->真数组
Array.from(likeArr).forEach(item => console.log(item))
4. 函数数组
const events = [
function () {
return '准备发射'
},
function () {
return '击中目标'
},
function () {
return '敌人投降'
},
]
// 箭头函数简化
const events = [() => '准备发射', () => '击中目标', () => '敌人投降']
events.forEach(ev => console.log(ev, typeof ev))
// ev 是一个函数, 要调用 ev()
events.forEach(ev => console.log(ev()))
5. 对象方法
/ 对象的方法, 其实就是属性,只不过它的值是一个匿名函数
const user = {
uname: '朱老师',
email: '498668472@qq.com',
getUser: function () {
// this: 当前对象的引用, user
return `${this.uname}: ( ${this.email} )`
},
}
console.log(user.getUser())
console.log('-----------------------')
// 改成数组
const userArr = [
'朱老师',
'498668472@qq.com',
function () {
return `${this[0]}: ( ${this[1]} )`
},
]
console.log(userArr[2]())
- 数组与对象的区别:
- 数组与对象并无本质区别,仅仅是语法上的不同
- 仅仅是成员声明方式和访问方式不同
- 显然对象的语义化更好
- 所以, 可以将数组,看成对象的一个子集或特例
- 函数的本质
- 函数是JS中,最重要的数据类型
- 函数可视为一个普通的值
- 函数可以充当返回值,参数等,凡是用到值的地方
- 如果没有return,则返回 undefined
效果
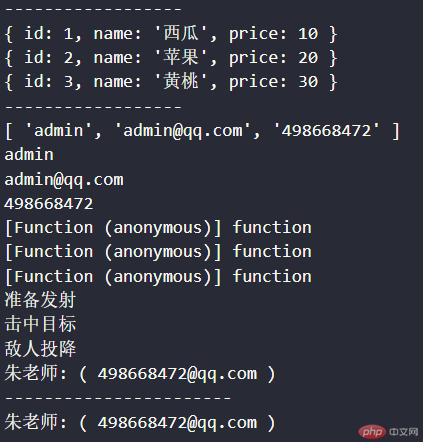