index.html
<!-- @format -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<script src="https://s2.pstatp.com/cdn/expire-1-M/jquery/3.4.0/jquery.min.js"></script>
<script src="data.js"></script>
<style>
table {
border-collapse: collapse;
border: 1px solid;
text-align: center;
margin: auto;
width: 500px;
}
table caption {
font-size: 1.2rem;
margin-bottom: 10px;
}
th,
td {
border: 1px solid;
padding: 5px;
}
thead tr:first-of-type {
background-color: #ddd;
}
p {
text-align: center;
}
p a {
text-decoration: none;
border: 1px solid;
padding: 0 8px;
}
.active {
background-color: #f00;
}
</style>
</head>
<body>
<table>
<caption>
用户信息表
</caption>
<thead>
<tr>
<th>id</th>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
<th>职位</th>
<th>手机号</th>
</tr>
</thead>
<tbody></tbody>
</table>
<p></p>
</body>
</html>
data.js
$(document).ready(function () {
var page = 1;
getData(page);
//发送请求
function getData(page) {
$.ajax({
type: "POST",
url: "data.php",
data: { page: page },
dataType: "json",
success: show,
});
}
//定义数据渲染方法
function show(data) {
// console.log(data);
//填充默认数据
console.log(page);
var str = "";
data.res.forEach(function (item) {
str += "<tr>";
str += "<td>" + item.id + "</td>";
str += "<td>" + item.name + "</td>";
str += "<td>" + item.age + "</td>";
str += "<td>" + item.sex + "</td>";
str += "<td>" + item.position + "</td>";
str += "<td>" + item.mobile + "</td>";
str += "</tr>";
});
$("tbody").html(str);
//分页
var str = "";
for (var i = 1; i < data.pages; i++) {
str += '<a href="" data-index=' + i + ">" + i + "</a>";
}
//为当前的页码添加样式
$("p")
.html(str)
.find("a")
.eq(page - 1)
.first()
.addClass("active");
$("p a").on("click", function (ev) {
console.log(this);
ev.preventDefault();
// // 获取当前要显示的新页面,根据自定义属性
page = $(this).attr("data-index");
getData(page);
});
}
});
data.php
<?php
$num = 10;
$page = $_POST['page'] ?? 1;
$offset = ($page - 1) * $num;
$dsn = "mysql:host=localhost;dbname=phpedu";
$pdo = new PDO($dsn, 'root', 'root');
$sql = "SELECT CEIL(COUNT(`id`)/{$num}) AS `Total` FROM users ";
$pages = $pdo->query($sql)->fetch()['Total'];
$stmt = $pdo->prepare('select * from users limit ? offset ?');
$stmt->bindParam(1, $num, PDO::PARAM_INT);
$stmt->bindParam(2, $offset, PDO::PARAM_INT);
$stmt->execute();
$res = $stmt->fetchAll(PDO::FETCH_ASSOC);
echo json_encode(['pages'=>$pages,'res'=>$res]);
效果如下:
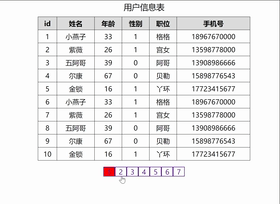
总结:学会使用JQ的ajax方法请求数据,并结合前面的知识将数据动态渲染到页面中.