迷你的MVC框架
Db.php
<?php
//命名空间
namespace mvc;
//创建类
class Db{
//配置数据库信息
private $db = [
'type' => 'mysql',
'host' => '127.0.0.1',
'dbname' => 'phpcn',
'username' => 'root',
'password' => 'root66',
];
public static $pdo;
//构造方法
private function __construct(...$params)
{
$dsn = $this->db['type'] . ':host=' .$this->db['host'] . ';dbname=' . $this->db['dbname'];
$username = $this->db['username'];
$password = $this->db['password'];
try{
self::$pdo = new \PDO($dsn,$username,$password);
// echo '测试连接成功';
}catch (\PDOException $e){
die('数据库失败 错误信息:' . $e->getMessage());
}
}
//单例模式判断重复连接
public static function connect(...$params){
//判断是否已经实例化
if (is_null(self::$pdo)){
new self(...$params);
}
return self::$pdo;
}
//禁止外部克隆方法
private function __clone()
{
// ****
}
// //新增,更新,删除数据库操作
// public static function exec($sql){
// $stmt = self::$pdo->prepare($sql);
// $stmt->execute();
// }
//获取单条数据
public static function fetch($sql){
$stmt = self::$pdo->prepare($sql);
$stmt->execute();
return $stmt->fetch(\PDO::FETCH_ASSOC);
}
//获取多条数据
public static function fetchAll($sql){
$stmt = self::$pdo->prepare($sql);
$stmt->execute();
return $stmt->fetchAll(\PDO::FETCH_ASSOC);
}
}
//new Db();
View.php
<?php
namespace mvc;
class View{
//获取多条数据
public function fetch($data){
$table = '<style>
table {border-collapse: collapse; border: 1px solid; width: 65%;margin:50px auto;}
caption {font-size: 1.8rem; margin-bottom: 20px;}
tr {height: 40px;}
tr:first-of-type { background-color:lightblue;}
td,th {border: 1px solid}
td{text-align: center}
td:first-of-type {text-align: center}
</style>';
$table .= '<table>';
$table .= '<caption>用户信息表<form action="index.php" method="get">
指定id:<input type="text" name="id">
<button>查询</button>
</form></caption>';
$table .= '<tr><th>ID</th><th>用户名</th><th>密码</th><th>注册时间</th><th>IP</th><th>操作</th></tr>';
//循环数组
foreach ($data as $v) {
$table .= '<tr>';
$table .= '<td>' . $v['id'] . '</td>';
$table .= '<td>' . $v['name'] . '</td>';
$table .= '<td>' . '无权查看' . '</td>';
$table .= '<td>' . date('Y-m-s h:i:s',$v['time']) . '</td>';
$table .= '<td>' . $v['ip'] . '</td>';
$table .= "<td><a href='index.php?id={$v['id']}'>查看</a> </td>";
$table .= '</tr>';
}
//date('Y-m-s h:i:s',time())
$table .= '</table>';
return $table;
}
//获取单条数据
public function find($data){
$table = '<style>
table {border-collapse: collapse; border: 1px solid; width: 65%;margin:50px auto;}
caption {font-size: 1.8rem; margin-bottom: 20px;}
tr {height: 40px;}
tr:first-of-type { background-color:indianred;}
td,th {border: 1px solid}
td{text-align: center}
td:first-of-type {text-align: center}
</style>';
$table .= '<table>';
$table .= '<caption>用户详情表</caption>';
$table .= '<tr><th>ID</th><th>用户名</th><th>密码</th><th>注册时间</th><th>IP</th></tr>';
$table .= '<tr>';
$table .= '<td>' . $data['id'] . '</td>';
$table .= '<td>' . $data['name'] . '</td>';
$table .= '<td>' . $data['pass'] . '</td>';
$table .= '<td>' . date('Y-m-s h:i:s',$data['time']) . '</td>';
$table .= '<td>' . $data['ip'] . '</td>';
$table .= '</tr>';
return $table;
}
}
Model.php
<?php
//命名空间
namespace mvc;
//引入Db文件
require __DIR__ . '/Db.php';
class Model{
public $data;
// 构造方法 连接数据库
public function __construct()
{
Db::connect();
}
//获取单条数据
public function get($id){
$sql = "SELECT * FROM `user` WHERE id= {$id}";
$this->data = Db::fetch($sql);
return $this->data;
}
//获取多条数据
public function getAll(){
$sql = "SELECT * FROM `user`";
$this->data = Db::fetchAll($sql);
return $this->data;
}
}
index.php
<?php
namespace mvc;
require __DIR__ . '/Model.php';
require __DIR__ . '/View.php';
//创建服务容器
class Container{
public $int = [];
public function bind($alias,\Closure $process){
$this->int[$alias] = $process;
}
public function make($alias, $params = []){
return call_user_func_array($this->int[$alias],$params);
}
}
$container = new Container();
$container->bind('model',function (){
return new Model();
});
$container->bind('view',function (){
return new View();
});
//门面模式
class Facade{
//接受实例化容器
protected static $container;
//绑定数据
protected static $data;
protected static $id;
public static function initialize(Container $container){
static::$container = $container;
}
public static function getAll(){
static::$data = static::$container->make('model')->getAll();
}
//查询单条数据
public static function get($id){
static::$id = static::$container->make('model')->get($id);
}
public static function fetch(){
return static::$container->make('view')->fetch(static::$data);
}
public static function find(){
return static::$container->make('view')->find(static::$id);
}
}
//创建控制器
class Controller{
public function __construct(Container $container)
{
Facade::initialize($container);
}
//多条数据
public function getAll(){
Facade::getAll();
return Facade::fetch();
}
//调用单条数据
public function find()
{
//获取数据
Facade::get($_GET['id']);
//渲染模板
return Facade::find();
// return print_r($_GET['id'],true);
}
}
$controller = new Controller($container);
//echo $controller->getAll();
if (is_null($_GET['id'])){
echo $controller->getAll();
}else{
echo $controller->find();
}
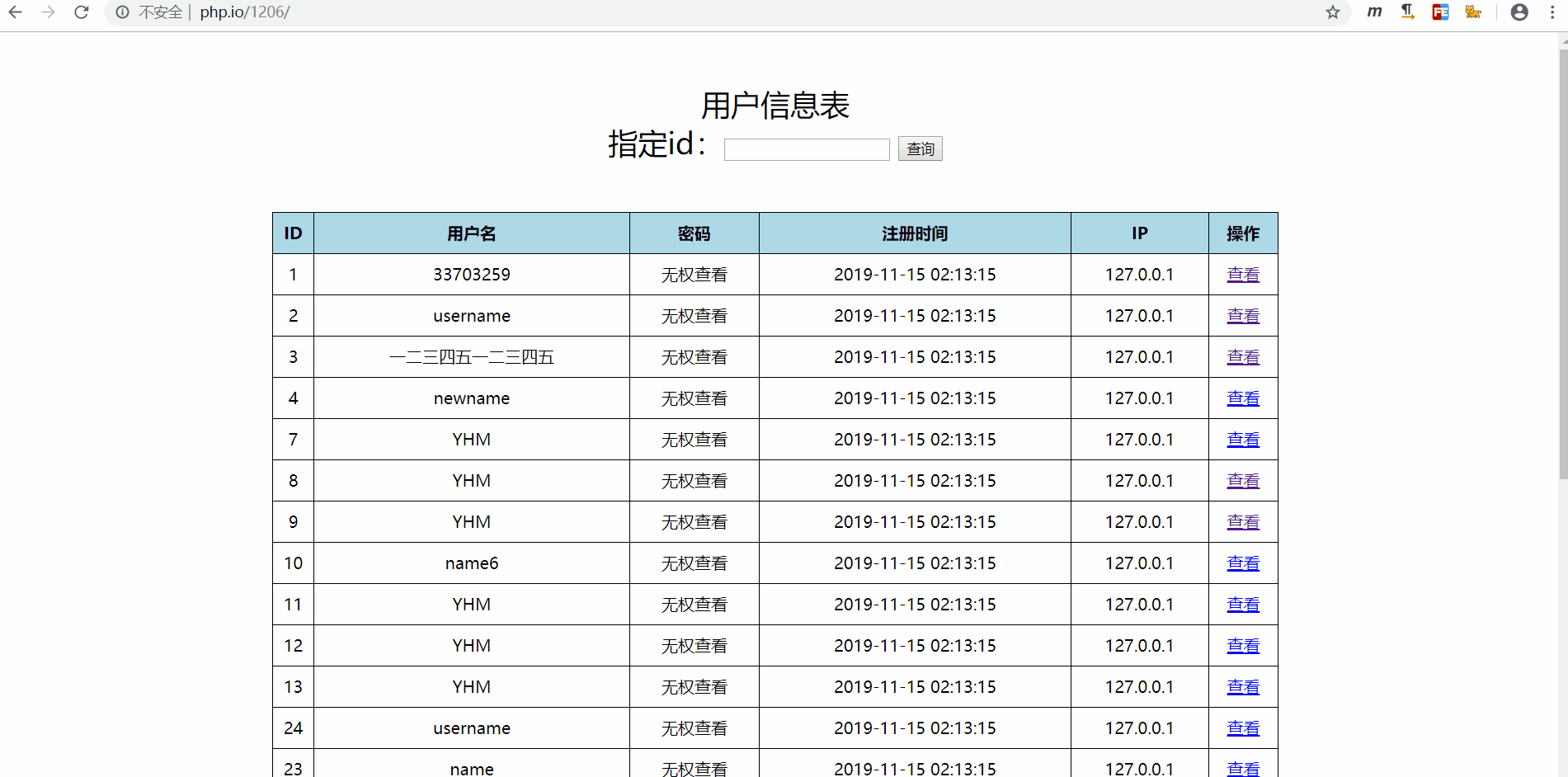