在本教學中,我們將逐步使用 Python 和 OpenAI API 建立生成式 AI 聊天機器人。我們將建立一個聊天機器人,它可以進行自然對話,同時保持上下文並提供有用的回應。
先決條件
- Python 3.8
- Python 程式設計的基本了解
- OpenAI API 金鑰
- RESTful API 基礎
設定環境
首先,讓我們設定我們的開發環境。建立一個新的Python專案並安裝所需的依賴項:
pip install openai python-dotenv streamlit
專案結構
我們的聊天機器人將具有乾淨的模組化結構:
chatbot/ ├── .env ├── app.py ├── chat_handler.py └── requirements.txt
執行
讓我們從 chat_handler.py 中的核心聊天機器人邏輯開始:
import openai from typing import List, Dict import os from dotenv import load_dotenv load_dotenv() class ChatBot: def __init__(self): openai.api_key = os.getenv("OPENAI_API_KEY") self.conversation_history: List[Dict[str, str]] = [] self.system_prompt = """You are a helpful AI assistant. Provide clear, accurate, and engaging responses while maintaining a friendly tone.""" def add_message(self, role: str, content: str): self.conversation_history.append({"role": role, "content": content}) def get_response(self, user_input: str) -> str: # Add user input to conversation history self.add_message("user", user_input) # Prepare messages for API call messages = [{"role": "system", "content": self.system_prompt}] + \ self.conversation_history try: # Make API call to OpenAI response = openai.ChatCompletion.create( model="gpt-3.5-turbo", messages=messages, max_tokens=1000, temperature=0.7 ) # Extract and store assistant's response assistant_response = response.choices[0].message.content self.add_message("assistant", assistant_response) return assistant_response except Exception as e: return f"An error occurred: {str(e)}"
現在,讓我們在 app.py 中使用 Streamlit 建立一個簡單的 Web 介面:
import streamlit as st from chat_handler import ChatBot def main(): st.title("? AI Chatbot") # Initialize session state if "chatbot" not in st.session_state: st.session_state.chatbot = ChatBot() # Chat interface if "messages" not in st.session_state: st.session_state.messages = [] # Display chat history for message in st.session_state.messages: with st.chat_message(message["role"]): st.write(message["content"]) # Chat input if prompt := st.chat_input("What's on your mind?"): # Add user message to chat history st.session_state.messages.append({"role": "user", "content": prompt}) with st.chat_message("user"): st.write(prompt) # Get bot response response = st.session_state.chatbot.get_response(prompt) # Add assistant response to chat history st.session_state.messages.append({"role": "assistant", "content": response}) with st.chat_message("assistant"): st.write(response) if __name__ == "__main__": main()
主要特點
- 對話記憶:聊天機器人透過儲存對話歷史記錄來維持上下文。
- 系統提示:我們透過系統提示定義聊天機器人的行為和個性。
- 錯誤處理:實作包含 API 呼叫的基本錯誤處理。
- 使用者介面:使用 Streamlit 的乾淨、直覺的 Web 介面。
運行聊天機器人
- 使用您的 OpenAI API 金鑰建立 .env 檔案:
OPENAI_API_KEY=your_api_key_here
- 運行應用程式:
streamlit run app.py
潛在的增強功能
- 對話持久化:新增資料庫整合來儲存聊天歷史記錄。
- 自訂個性:讓使用者選擇不同的聊天機器人個性。
- 輸入驗證:增加更強大的輸入驗證和清理。
- API 速率限制:實作速率限制來管理 API 使用。
- 回應流:新增串流回應以獲得更好的使用者體驗。
結論
此實作示範了一個基本但實用的生成式 AI 聊天機器人。模組化設計可以根據特定需求輕鬆擴展和自訂。雖然此範例使用 OpenAI 的 API,但相同的原理也可以應用於其他語言模型或 API。
請記住,部署聊天機器人時,您應該考慮:
- API 成本與使用限制
- 使用者資料隱私與安全
- 反應延遲與最佳化
- 輸入驗證與內容審核
資源
- OpenAI API 文件
- 精簡文件
- Python 環境管理
以上是建立簡單的生成式人工智慧聊天機器人:實用指南的詳細內容。更多資訊請關注PHP中文網其他相關文章!
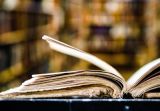
本教程演示如何使用Python處理Zipf定律這一統計概念,並展示Python在處理該定律時讀取和排序大型文本文件的效率。 您可能想知道Zipf分佈這個術語是什麼意思。要理解這個術語,我們首先需要定義Zipf定律。別擔心,我會盡量簡化說明。 Zipf定律 Zipf定律簡單來說就是:在一個大型自然語言語料庫中,最頻繁出現的詞的出現頻率大約是第二頻繁詞的兩倍,是第三頻繁詞的三倍,是第四頻繁詞的四倍,以此類推。 讓我們來看一個例子。如果您查看美國英語的Brown語料庫,您會注意到最頻繁出現的詞是“th
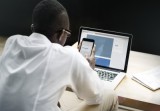
Python 提供多種從互聯網下載文件的方法,可以使用 urllib 包或 requests 庫通過 HTTP 進行下載。本教程將介紹如何使用這些庫通過 Python 從 URL 下載文件。 requests 庫 requests 是 Python 中最流行的庫之一。它允許發送 HTTP/1.1 請求,無需手動將查詢字符串添加到 URL 或對 POST 數據進行表單編碼。 requests 庫可以執行許多功能,包括: 添加表單數據 添加多部分文件 訪問 Python 的響應數據 發出請求 首
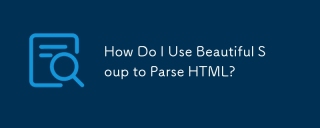
本文解釋瞭如何使用美麗的湯庫來解析html。 它詳細介紹了常見方法,例如find(),find_all(),select()和get_text(),以用於數據提取,處理不同的HTML結構和錯誤以及替代方案(SEL)
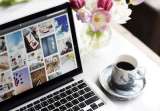
處理嘈雜的圖像是一個常見的問題,尤其是手機或低分辨率攝像頭照片。 本教程使用OpenCV探索Python中的圖像過濾技術來解決此問題。 圖像過濾:功能強大的工具圖像過濾器
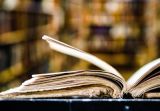
PDF 文件因其跨平台兼容性而廣受歡迎,內容和佈局在不同操作系統、閱讀設備和軟件上保持一致。然而,與 Python 處理純文本文件不同,PDF 文件是二進製文件,結構更複雜,包含字體、顏色和圖像等元素。 幸運的是,借助 Python 的外部模塊,處理 PDF 文件並非難事。本文將使用 PyPDF2 模塊演示如何打開 PDF 文件、打印頁面和提取文本。關於 PDF 文件的創建和編輯,請參考我的另一篇教程。 準備工作 核心在於使用外部模塊 PyPDF2。首先,使用 pip 安裝它: pip 是 P

本教程演示瞭如何利用Redis緩存以提高Python應用程序的性能,特別是在Django框架內。 我們將介紹REDIS安裝,Django配置和性能比較,以突出顯示BENE
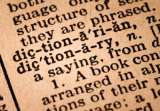
自然語言處理(NLP)是人類語言的自動或半自動處理。 NLP與語言學密切相關,並與認知科學,心理學,生理學和數學的研究有聯繫。在計算機科學
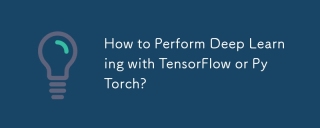
本文比較了Tensorflow和Pytorch的深度學習。 它詳細介紹了所涉及的步驟:數據準備,模型構建,培訓,評估和部署。 框架之間的關鍵差異,特別是關於計算刻度的


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

SublimeText3漢化版
中文版,非常好用
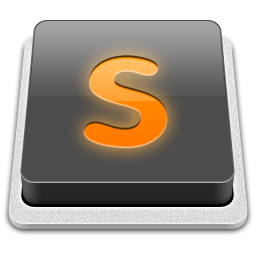
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。
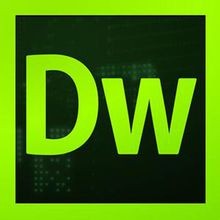
Dreamweaver CS6
視覺化網頁開發工具

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中