Dynamo DB 是 AWS 在大量託管資料庫中提供的 NoSQL 產品,作為其提供的服務。與大多數其他服務一樣,它完全無伺服器、靈活且易於擴展。
資料模型
由於我們正在研究 NoSQL,因此對資料結構沒有真正的限制。我們可以使用鍵值對作為表中每個項的屬性進行操作。 讓我們再看看這些關鍵字。
表 - 一個相當熟悉的術語,它本質上是資料的集合,在本例中為項目。這也是在控制台上使用 DynamoDB 的起點。
Item - 表中的條目。您可以將其視為 SQL 等效資料庫中的一行。
屬性 - 構成項目的資料點。它可以包含特定於項目的屬性、元資料或幾乎任何可以與項目關聯的內容。
您可以將 JSON 陣列視為 DynamoDB 中的表格的等效項。我相信當我們創建自己的表格時,事情會變得更加清晰。
設定資料庫
從 AWS 控制台在 DynamoDB 中建立新表簡直就是小菜一碟。您所需要的只是一個名稱和一個分區鍵,在本例中這是您的主鍵。這將幫助您搜尋表中的項目。
我正在為我玩過的所有遊戲創建一個表格,我會給它們評分(滿分 10 分):)
您可以直接從控制台弄亂表格,讓我們嘗試新增一個項目看看它是什麼樣子。
我的第一個作品必須是我最喜歡的 RPG(角色扮演)遊戲 - 《巫師 3》。我將為評分添加一個新屬性,這將是我的 9.8 分:)
設定 API
對了,現在是時候編寫一些 Python 程式碼來在沒有 GUI 的情況下完成所有這些工作了;)
## app.py from flask import Flask, jsonify, request import boto3 from boto3.dynamodb.conditions import Key import uuid # Import uuid module for generating UUIDs app = Flask(__name__) # Initialize DynamoDB client dynamodb = boto3.resource('dynamodb', region_name='ap-south-1') # Replace with your region ## Do keep in mind to save your AWS credentials file in the root directory table = dynamodb.Table('games') # Replace with your table name # Route to get all games @app.route('/games', methods=['GET']) def get_games(): try: response = table.scan() games = response.get('Items', []) return jsonify({'games': games}), 200 except Exception as e: return jsonify({'error': str(e)}), 500 if __name__ == '__main__': app.run(debug=True)
Python 的美妙之處在於您只需幾行程式碼即可設定完整的 API。現在,這段程式碼足以讓我們存取該表並從中獲取資料。我們使用掃描功能從遊戲桌上取得項目。
您可以使用 python3 app.py 啟動應用程式
當您捲曲 /games 端點時,您可以期待如下所示的回應。
建立和更新條目的路由
## app.py from flask import Flask, jsonify, request import boto3 from boto3.dynamodb.conditions import Key import uuid # Import uuid module for generating UUIDs app = Flask(__name__) # Initialize DynamoDB client dynamodb = boto3.resource('dynamodb', region_name='ap-south-1') # Replace with your region ## Do keep in mind to save your AWS credentials file in the root directory table = dynamodb.Table('games') # Replace with your table name # Route to get all games @app.route('/games', methods=['GET']) def get_games(): try: response = table.scan() games = response.get('Items', []) return jsonify({'games': games}), 200 except Exception as e: return jsonify({'error': str(e)}), 500 if __name__ == '__main__': app.run(debug=True)
在這裡,我們使用 put_item 將項目新增到表中。為了更新記錄,我們使用函數 update_item。
如果您仔細觀察,我們正在使用 UpdateExpression 來指定要更新的屬性。這使我們能夠準確控制哪個屬性被更改並避免意外覆蓋。
要刪除記錄,您可以使用類似的操作 -
# Route to create a new game @app.route('/games', methods=['POST']) def create_game(): try: game_data = request.get_json() name = game_data.get('name') rating = game_data.get('rating') hours = game_data.get('hours', 0) # Generate a random UUID for the new game id = str(uuid.uuid4()) if not name or not rating: return jsonify({'error': 'Missing required fields'}), 400 # Store the game in DynamoDB table.put_item(Item={'id': id, 'name': name, 'rating': rating, 'hours': hours}) # Return the created game with the generated UUID created_game = {'id': id, 'name': name, 'rating': rating} return jsonify({'message': 'Game added successfully', 'game': created_game}), 201 except Exception as e: return jsonify({'error': str(e)}), 500 # Route to update an existing game @app.route('/games/<id>', methods=['PUT']) def update_game(id): try: game_data = request.get_json() name = game_data.get('name') rating = game_data.get('rating') hours = game_data.get('hours', 0) if not name and not rating: return jsonify({'error': 'Nothing to update'}), 400 update_expression = 'SET ' expression_attribute_values = {} if name: update_expression += ' #n = :n,' expression_attribute_values[':n'] = name if rating: update_expression += ' #r = :r,' expression_attribute_values[':r'] = rating if hours: update_expression += ' #h = :h,' expression_attribute_values[':h'] = hours update_expression = update_expression[:-1] # remove trailing comma response = table.update_item( Key={'id': id}, UpdateExpression=update_expression, ExpressionAttributeNames={'#n': 'name', '#r': 'rating', '#h': 'hours'}, ExpressionAttributeValues=expression_attribute_values, ReturnValues='UPDATED_NEW' ) updated_game = response.get('Attributes', {}) return jsonify(updated_game), 200 except Exception as e: return jsonify({'error': str(e)}), 500 </id>
好了,你已經完成了,借助 Python,您只需幾分鐘即可為 DynamoDB 設定具有 CRUD 功能的 REST API。
以上是在 Python 中為 DynamoDB 設定 REST API的詳細內容。更多資訊請關注PHP中文網其他相關文章!
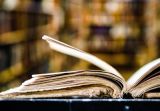
本教程演示如何使用Python處理Zipf定律這一統計概念,並展示Python在處理該定律時讀取和排序大型文本文件的效率。 您可能想知道Zipf分佈這個術語是什麼意思。要理解這個術語,我們首先需要定義Zipf定律。別擔心,我會盡量簡化說明。 Zipf定律 Zipf定律簡單來說就是:在一個大型自然語言語料庫中,最頻繁出現的詞的出現頻率大約是第二頻繁詞的兩倍,是第三頻繁詞的三倍,是第四頻繁詞的四倍,以此類推。 讓我們來看一個例子。如果您查看美國英語的Brown語料庫,您會注意到最頻繁出現的詞是“th
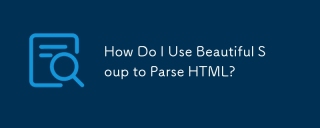
本文解釋瞭如何使用美麗的湯庫來解析html。 它詳細介紹了常見方法,例如find(),find_all(),select()和get_text(),以用於數據提取,處理不同的HTML結構和錯誤以及替代方案(SEL)
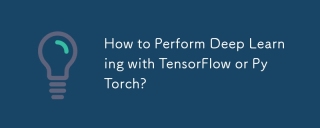
本文比較了Tensorflow和Pytorch的深度學習。 它詳細介紹了所涉及的步驟:數據準備,模型構建,培訓,評估和部署。 框架之間的關鍵差異,特別是關於計算刻度的
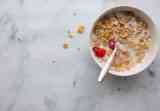
Python 對象的序列化和反序列化是任何非平凡程序的關鍵方面。如果您將某些內容保存到 Python 文件中,如果您讀取配置文件,或者如果您響應 HTTP 請求,您都會進行對象序列化和反序列化。 從某種意義上說,序列化和反序列化是世界上最無聊的事情。誰會在乎所有這些格式和協議?您想持久化或流式傳輸一些 Python 對象,並在以後完整地取回它們。 這是一種在概念層面上看待世界的好方法。但是,在實際層面上,您選擇的序列化方案、格式或協議可能會決定程序運行的速度、安全性、維護狀態的自由度以及與其他系
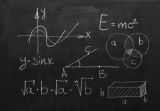
Python的statistics模塊提供強大的數據統計分析功能,幫助我們快速理解數據整體特徵,例如生物統計學和商業分析等領域。無需逐個查看數據點,只需查看均值或方差等統計量,即可發現原始數據中可能被忽略的趨勢和特徵,並更輕鬆、有效地比較大型數據集。 本教程將介紹如何計算平均值和衡量數據集的離散程度。除非另有說明,本模塊中的所有函數都支持使用mean()函數計算平均值,而非簡單的求和平均。 也可使用浮點數。 import random import statistics from fracti
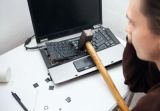
在本教程中,您將從整個系統的角度學習如何處理Python中的錯誤條件。錯誤處理是設計的關鍵方面,它從最低級別(有時是硬件)一直到最終用戶。如果y
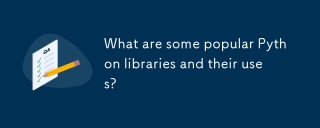
本文討論了諸如Numpy,Pandas,Matplotlib,Scikit-Learn,Tensorflow,Tensorflow,Django,Blask和請求等流行的Python庫,並詳細介紹了它們在科學計算,數據分析,可視化,機器學習,網絡開發和H中的用途
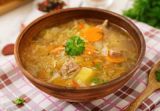
該教程建立在先前對美麗湯的介紹基礎上,重點是簡單的樹導航之外的DOM操縱。 我們將探索有效的搜索方法和技術,以修改HTML結構。 一種常見的DOM搜索方法是EX


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。
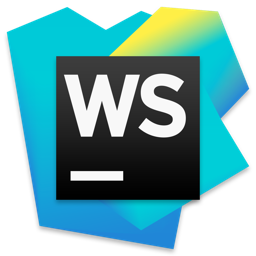
WebStorm Mac版
好用的JavaScript開發工具

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),