리액트 컴포넌트 간의 상호 호출에서 호출자를 부모 컴포넌트, 호출 수신자를 자식 컴포넌트라고 합니다. 부모 컴포넌트와 자식 컴포넌트 사이에 값을 전달할 수 있습니다. 1. 부모 컴포넌트가 자식 컴포넌트에 값을 전달하면 전달할 값이 먼저 자식 컴포넌트에 전달되고, 그 다음 자식 컴포넌트에서는 props를 사용하여 2. 자식 컴포넌트 부모 컴포넌트에 값을 전달할 때, 트리거 메소드를 통해 부모 컴포넌트에 값을 전달해야 합니다.
이 튜토리얼의 운영 환경: Windows 7 시스템, React18 버전, Dell G3 컴퓨터.
React 구성 요소는 자체 정의된 HTML이 아닌 태그입니다. React 구성 요소는 첫 글자를 대문자로 사용해야 합니다
: 首字母大写
:
class App extends Component{ } <app></app>
组件的相互调用中,把调用者
称为父组件,被调用者
称为子组件:
import React from 'react'; import Children from './Children'; class Up extends React.Component { constructor(props){ super(props); this.state = { } } render(){ console.log("render"); return( <div> up <children></children> </div> ) } } export default Up;
import React from 'react'; class Children extends React.Component{ constructor(props){ super(props); this.state = { } } render(){ return ( <div> Children </div> ) } } export default Children;
父组件向子组件传值使用props。父组件向子组件传值时,先将需要传递的值传递给子组件,然后在子组件中,使用props来接收父组件传递过来的值。
父组件在调用子组件的时候定义一个属性:
<children></children>
这个值msg
会绑定在子组件的props
this.props.msg
호출자
를 상위 구성 요소라고 하고 호출자
를 하위 구성 요소라고 합니다. import React from 'react'; import Children from './Children'; class Up extends React.Component { constructor(props){ super(props); this.state = { } } render(){ console.log("render"); return( <div> up <Children msg="父组件传值给子组件" /> </div> ) } } export default Up;
import React from 'react'; class Children extends React.Component{ constructor(props){ super(props); this.state = { } } render(){ return ( <div> Children <br> {this.props.msg} </div> ) } } export default Children;
상위 구성 요소는 하위 구성 요소를 호출할 때 속성을 정의합니다.
import React from 'react'; import Children from './Children'; class Up extends React.Component { constructor(props){ super(props); this.state = { } } run = () => { console.log("父组件run方法"); } render(){ console.log("render"); return(이 값up <children></children>) } } export default Up;
msg
는 하위 구성 요소의 props
속성에 바인딩되고 하위 구성 요소는 직접 사용할 수 있습니다.import React from 'react'; class Children extends React.Component{ constructor(props){ super(props); this.state = { } } run = () => { this.props.run(); } render(){ return ( <div> Children <br> <button>Run</button> </div> ) } } export default Children;상위 구성 요소는 값과 메소드를 구성 요소에 전달할 수 있으며, 심지어 자신을 하위 구성 요소에 전달할 수도 있습니다.
3.1 값 전달
import React from 'react'; import Children from './Children'; class Up extends React.Component { constructor(props){ super(props); this.state = { } } run = () => { console.log("父组件run方法"); } render(){ console.log("render"); return( <div> up <Children msg={this}/> </div> ) } } export default Up;
import React from 'react'; class Children extends React.Component{ constructor(props){ super(props); this.state = { } } run = () => { console.log(this.props.msg); } render(){ return ( <div> Children <br> <button>Run</button> </div> ) } } export default Children;
3.2 메소드 전달
import React from 'react'; import Children from './Children'; class Up extends React.Component { constructor(props){ super(props); this.state = { } } getChildrenData = (data) => { console.log(data); } render(){ console.log("render"); return(up <children></children>) } } export default Up;
import React from 'react'; class Children extends React.Component{ constructor(props){ super(props); this.state = { } } render(){ return ( <div> Children <br> <button> {this.props.upFun("子组件数据")}}>Run</button> </div> ) } } export default Children;
import React from 'react'; import Children from './Children'; class Up extends React.Component { constructor(props){ super(props); this.state = { } } clickButton = () => { console.log(this.refs.children); } render(){ console.log("render"); return(rrreee🎜🎜4 하위 구성 요소가 상위 구성 요소에 값을 전달합니다🎜🎜🎜하위 구성 요소가 해당 값을 상위 구성 요소에 전달합니다. 트리거 메서드를 통한 구성 요소🎜rrreeerrreee🎜🎜🎜🎜🎜5. 상위 구성 요소 refs🎜🎜rrreee🎜를 통해 하위 구성 요소 특성 및 메서드 가져오기[관련 권장 사항: 🎜Redis 비디오 튜토리얼🎜]🎜up <children></children>) } } export default Up; ``` ```js import React from 'react'; class Children extends React.Component{ constructor(props){ super(props); this.state = { title: "子组件" } } runChildren = () => { } render(){ return (Children) } } export default Children; ``` 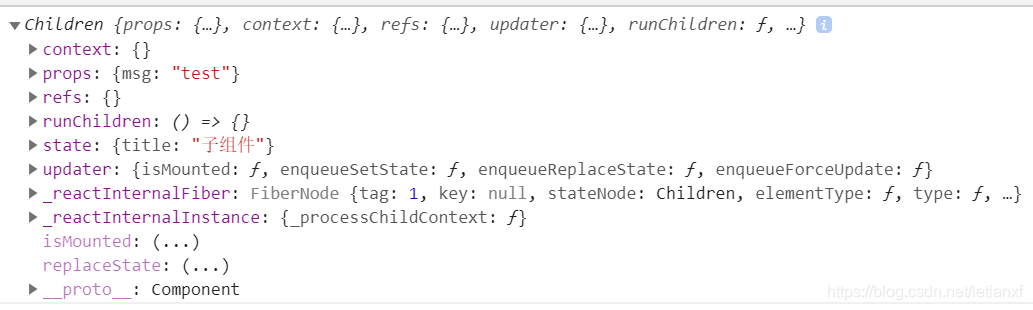
위 내용은 반응의 부모-자식 구성 요소는 무엇입니까의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!