HTTP is a stateless protocol, which means that each time the client retrieves a web page, a separate server connection must be opened, so the server does not record any information from previous client requests.
There are three ways to maintain the session between the client and the server:
Cookies
The web server can assign a unique session ID as a cookie to represent each client and is used to identify subsequent requests from this client.
This may not be an effective way, because many times browsers do not necessarily support cookies, so we do not recommend using this method to maintain the session.
Hidden form fields
A web server can send a hidden HTML form field and a unique session ID, like this:
<input type="hidden" name="sessionid" value="12345">
This entry means that when the form is submitted, The specified name and value will be automatically included in the GET or POST data. Whenever the browser sends a request, the value of session_id can be used to save traces of different browsers.
This method may be an effective method, but the form submission event will not be generated when clicking the hyperlink in the <A HREF> tag, so hidden form fields do not support universal session tracking.
Rewriting URL
You can add some extra data after each URL to distinguish the session, and the server can associate the session identifier based on this data.
For example, http://w3cschool.cc/file.htm;sessionid=12345, the session identifier is sessionid=12345, the server can use this data to identify the client.
In comparison, rewriting the URL is a better way. It can work even if the browser does not support cookies, but the disadvantage is that you must dynamically specify the session ID for each URL, even if this is a simple HTML page.
session object
In addition to the above methods, JSP uses the HttpSession interface provided by servlet to identify a user and store all access information of this user.
By default, JSP allows session tracking, and a new HttpSession object will be automatically instantiated for new clients. Disabling session tracking requires explicitly turning it off, which is achieved by setting the session attribute value in the page directive to false, as follows:
<%@ page session="false" %>
The JSP engine exposes the implicit session object to developers. Since the session object is provided, developers can easily store or retrieve data.
The following table lists some important methods of the session object:
S.N. | Methods & describe |
---|
1 | public Object getAttribute(String name)
Returns the object bound to the specified name in the session object. If it does not exist, returns null |
2 | public Enumeration getAttributeNames()
Returns all the object names in the session object |
3 | public long getCreationTime()
Returns the time when the session object was created, in milliseconds, starting from the early morning of January 1, 1970 |
4 | public String getId()
Returns the ID of the session object |
5 | public long getLastAccessedTime()
Returns the last access time of the client, in milliseconds, starting from the early morning of January 1, 1970 |
6 | public int getMaxInactiveInterval()
Returns the maximum time interval, in seconds, during which the servlet container will keep the session open |
7 | public void invalidate()
Invalidate the session and unbind any objects bound to the session |
8 | public boolean isNew(
Returns whether it is a new client, or whether the client refuses to join the session |
##
9 | public void removeAttribute(String name)
Remove the object with the specified name in the session
|
10 | public void setAttribute(String name, Object value)
Use the specified name and value to generate an object and bind it to the session
|
11 | public void setMaxInactiveInterval(int interval)
Used to specify the time, in seconds, during which the servlet container will keep the session valid
|
JSP Session Application
This example describes how to use the HttpSession object to obtain the creation time and last access time. We will associate a new session object with the request object if it does not already exist.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.io.*,java.util.*" %>
<%
// 获取session创建时间
Date createTime = new Date(session.getCreationTime());
// 获取最后访问页面的时间
Date lastAccessTime = new Date(session.getLastAccessedTime());
String title = "再次访问php中文网实例";
Integer visitCount = new Integer(0);
String visitCountKey = new String("visitCount");
String userIDKey = new String("userID");
String userID = new String("ABCD");
// 检测网页是否由新的访问用户
if (session.isNew()){
title = "访问php中文网实例";
session.setAttribute(userIDKey, userID);
session.setAttribute(visitCountKey, visitCount);
} else {
visitCount = (Integer)session.getAttribute(visitCountKey);
visitCount += 1;
userID = (String)session.getAttribute(userIDKey);
session.setAttribute(visitCountKey, visitCount);
}
%>
<html>
<head>
<title>Session 跟踪</title>
</head>
<body>
<h1>Session 跟踪</h1>
<table border="1" align="center">
<tr bgcolor="#949494">
<th>Session 信息</th>
<th>值</th>
</tr>
<tr>
<td>id</td>
<td><% out.print( session.getId()); %></td>
</tr>
<tr>
<td>创建时间</td>
<td><% out.print(createTime); %></td>
</tr>
<tr>
<td>最后访问时间</td>
<td><% out.print(lastAccessTime); %></td>
</tr>
<tr>
<td>用户 ID</td>
<td><% out.print(userID); %></td>
</tr>
<tr>
<td>访问次数</td>
<td><% out.print(visitCount); %></td>
</tr>
</table>
</body>
</html>
Try to access http://localhost:8080/testjsp/main.jsp, you will get the following results when you run it for the first time:
Access again and you will get the following results:
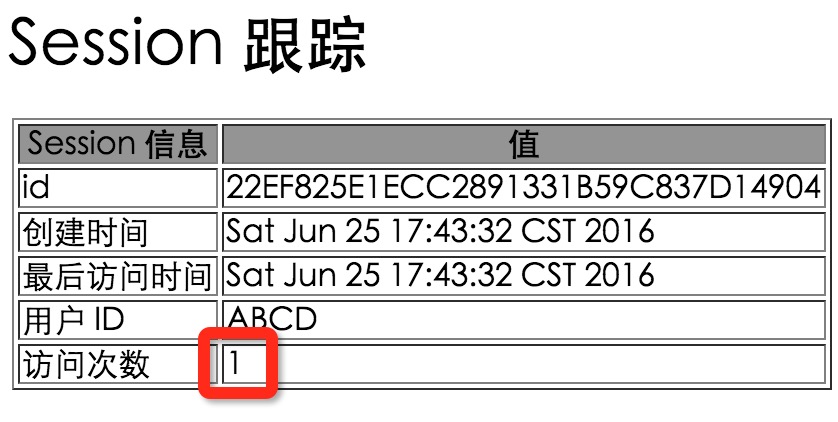
Delete Session data
When a user's session data is processed Finally, you have the following options:
Remove a specific attribute:
Call the public void removeAttribute(String name) method to remove the specified attribute.
Delete the entire session:
Call the public void invalidate() method to invalidate the entire session.
Set the session validity period:
Call the public void setMaxInactiveInterval(int interval) method to set the session timeout.
Logout user:
For servers that support servlet2.4 version, you can call the logout() method to log out the user and invalidate all related sessions.
Configure the web.xml file:
If you are using Tomcat, you can configure the web.xml file as follows:
<session-config>
<session-timeout>15</session-timeout>
</session-config>
The timeout is in minutes, The default timeout in Tomcat is 30 minutes.
The getMaxInactiveInterval() method in Servlet returns the timeout in seconds. If 15 minutes is configured in web.xml, the getMaxInactiveInterval() method will return 900.