Serial number | Method & Description |
---|
1 | public void setDomain(String pattern)
Set the domain name of the cookie, such as w3cschool.cc |
2 | public String getDomain()
Get the domain name of the cookie, such as w3cschool.cc |
3 | public void setMaxAge(int expiry)
Set the cookie validity period in seconds. The default validity period is the survival time of the current session |
4 | public int getMaxAge()
Get the cookie validity period, in seconds, the default is -1, indicating that the cookie will live until the browser is closed |
##
5 | public String getName()
Returns the name of the cookie. The name cannot be modified after it is created.
|
6 | public void setValue(String newValue)
Set the cookie value
|
7 | public String getValue()
Get the value of cookie
|
8 | public void setPath(String uri)
Set the cookie path, which defaults to all URLs in the current page directory, as well as all subdirectories in this directory
|
##
9 | public String getPath()
Get the path of the cookie
|
10 | public void setSecure(boolean flag)
Indicate whether the cookie should be encrypted for transmission
|
11 | public void setComment(String purpose)
Set a comment describing the purpose of the cookie. Comments will become very useful when the browser displays the cookie to the user
|
12 | public String getComment()
Returns a comment describing the purpose of the cookie, or null if none
|
Setting Cookie using JSP
Setting cookie using JSP involves three steps:
(1) Create a Cookie object: Call the Cookie constructor , using a cookie name and value as parameters, both of which are strings.
Cookie cookie = new Cookie("key","value");
Please remember that neither the name nor the value can contain spaces or the following characters:
[ ] ( ) = , " / ? @ : ;
(2) Set the validity period: Call the setMaxAge() function to indicate the cookie How long (in seconds) it is valid for. The following operation sets the validity period to 24 hours.
cookie.setMaxAge(60*60*24);
(3) Send cookie to HTTP response header: Call response.addCookie() function to add cookie to HTTP response header.
response.addCookie(cookie);
Example demonstration
The main.jsp file code is as follows:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.net.*" %>
<%
// 编码,解决中文乱码
String str = URLEncoder.encode(request.getParameter("name"),"utf-8");
// 设置 name 和 url cookie
Cookie name = new Cookie("name",
str);
Cookie url = new Cookie("url",
request.getParameter("url"));
// 设置cookie过期时间为24小时。
name.setMaxAge(60*60*24);
url.setMaxAge(60*60*24);
// 在响应头部添加cookie
response.addCookie( name );
response.addCookie( url );
%>
<html>
<head>
<title>设置 Cookie</title>
</head>
<body>
<h1>设置 Cookie</h1>
<ul>
<li><p><b>网站名:</b>
<%= request.getParameter("name")%>
</p></li>
<li><p><b>网址:</b>
<%= request.getParameter("url")%>
</p></li>
</ul>
</body>
</html>
The following is a simple HTML form that submits client data to the main.jsp file, and set cookies:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>php中文网(php.cn)</title>
</head>
<body>
<form action="main.jsp" method=GET>
站点名: <input type="text" name="name">
<br />
网址: <input type="text" name="url" />
<input type="submit" value="提交" />
</form>
</body>
</html>
Save the above HTML code to the test.htm file.
Place this file in the WebContent directory of the current jsp project (the same directory as main.jsp).
Submit the form data to the main.jsp file by visiting http://localhost:8080/testjsp/test.html. The demonstration Gif image is as follows:

Try to enter "site name" and "web address", and then click the submit button, it will display "site name" and "web address" in your screen, and set both "site name" and "web address" cookies.
Reading Cookies using JSP
To read cookies, you need to call the request.getCookies() method to obtain an array of javax.servlet.http.Cookie objects. Then iterate through this array and use the getName() method and getValue() method to get the name and value of each cookie.
<h3 Example Demonstration< h3="">Let us read the cookie in the previous example. The following is the cookie.jsp file code:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.net.*" %>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>获取 Cookie</title>
</head>
<body>
<%
Cookie cookie = null;
Cookie[] cookies = null;
// 获取cookies的数据,是一个数组
cookies = request.getCookies();
if( cookies != null ){
out.println("<h2> 查找 Cookie 名与值</h2>");
for (int i = 0; i < cookies.length; i++){
cookie = cookies[i];
out.print("参数名 : " + cookie.getName());
out.print("<br>");
out.print("参数值: " + URLDecoder.decode(cookie.getValue(), "utf-8") +" <br>");
out.print("------------------------------------<br>");
}
}else{
out.println("<h2>没有发现 Cookie</h2>");
}
%>
</body>
</html>
After browser access , the output result is:
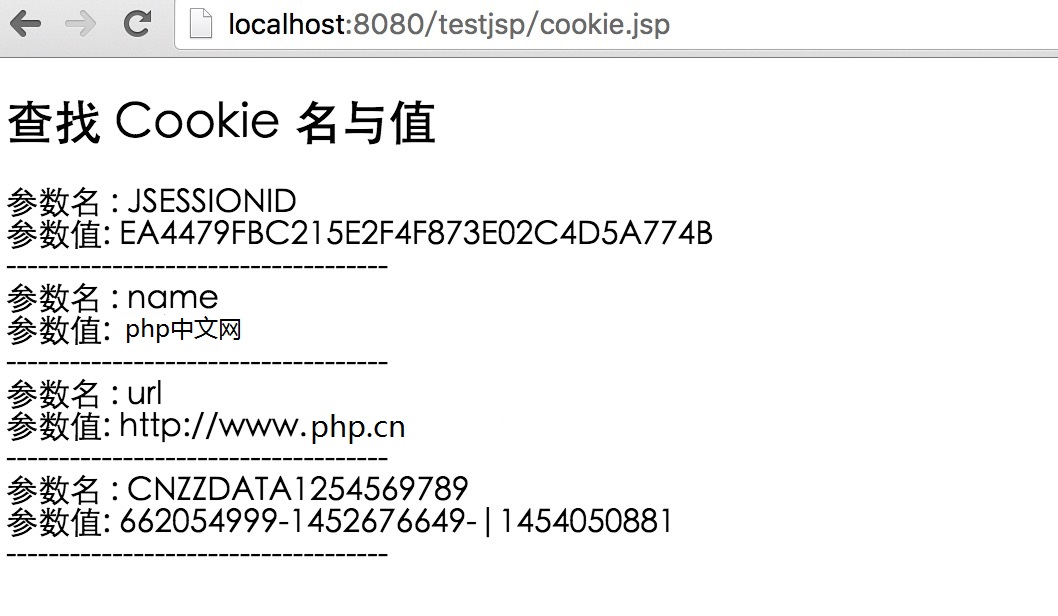
Deleting Cookies using JSP
Deleting cookies is very simple. If you want to delete a cookie, just follow the steps given below:
Get an existing cookie and store it in the Cookie object.
Set the cookie expiry date to 0.
Add this cookie back to the response header.
Example demonstration
The following program deletes a cookie named "name". When you run cookie.jsp for the second time, name will is null.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page import="java.net.*" %>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>获取 Cookie</title>
</head>
<body>
<%
Cookie cookie = null;
Cookie[] cookies = null;
// 获取当前域名下的cookies,是一个数组
cookies = request.getCookies();
if( cookies != null ){
out.println("<h2> 查找 Cookie 名与值</h2>");
for (int i = 0; i < cookies.length; i++){
cookie = cookies[i];
if((cookie.getName( )).compareTo("name") == 0 ){
cookie.setMaxAge(0);
response.addCookie(cookie);
out.print("删除 Cookie: " +
cookie.getName( ) + "<br/>");
}
out.print("参数名 : " + cookie.getName());
out.print("<br>");
out.print("参数值: " + URLDecoder.decode(cookie.getValue(), "utf-8") +" <br>");
out.print("------------------------------------<br>");
}
}else{
out.println("<h2>没有发现 Cookie</h2>");
}
%>
</body>
</html>
Access through the browser, the output result is:
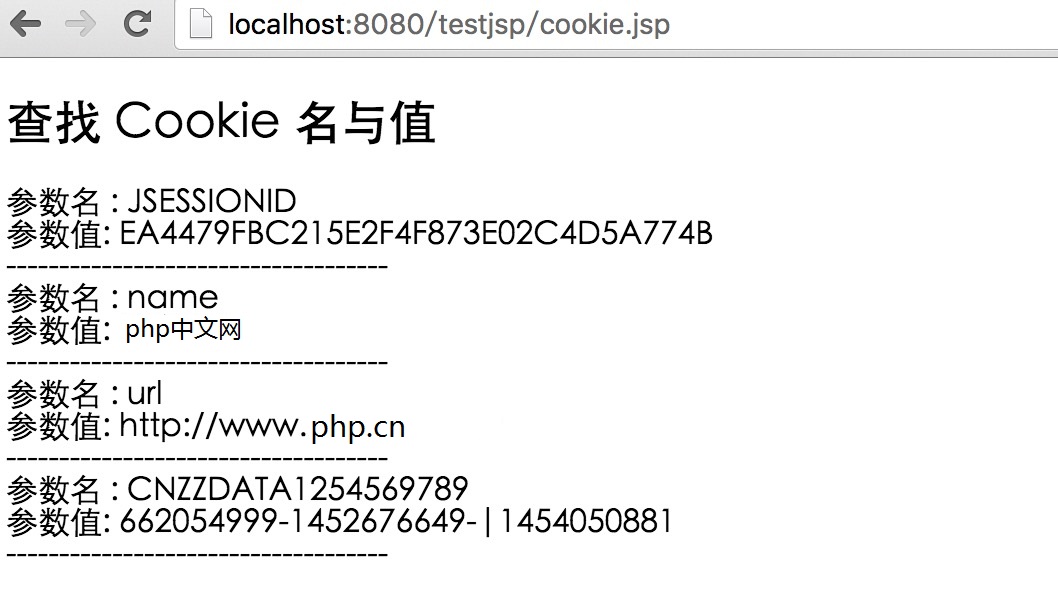
Visit http://localhost:8080/testjsp/cookie.jsp again, you will get the following results:
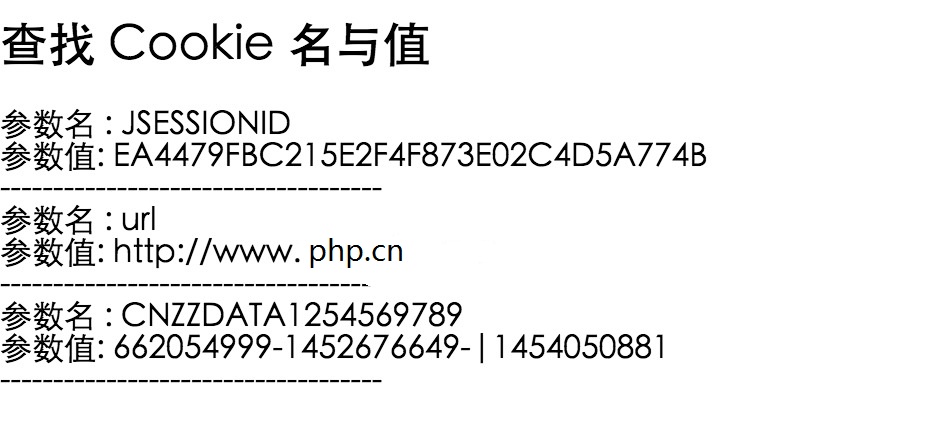
You can see that the cookie named width="70%" "name" has disappeared.
You can also manually delete cookies in your browser. In the IE browser, you can delete all cookies by clicking the Tools menu item, then selecting Internet Options, and clicking Delete Cookies.