The operation of a computer program requires the operation of values such as the number 3.14 or the text "hello world". In programming languages, the type of values that can be represented and manipulated is called data type (type). The most basic of programming languages The feature is to host multiple data types. When a program needs to save a value for future use, it assigns it ("saves" the value to) a variable. A variable is a symbolic name for a value, and a reference to the value can be obtained by name. How variables work is a fundamental feature of programming languages. This chapter will refer to the previous section to help understand the content of this chapter, and will be explained in more depth later.
Javascript data is divided into two categories: primitive type and object type
The original classes in JavaScript include numbers, strings, and Boolean values. This chapter will have a separate chapter dedicated to numbers, strings, and Boolean values in JavaScript. JavaScript also has two special primitive values, null (empty) and Undefined (undefined). They are not numbers, strings, or Boolean values. They each represent a unique member of their own special type.
Javascript is an object in addition to numbers, strings, Boolean values, null, and undefined. An object is a collection of properties. Each attribute is composed of a "name/value pair" (the value can be a primitive value, such as a number, a string, or an object). One of the more special objects (global object will be introduced in Miss 5, and will be described in more detail in Section 6)
Ordinary JavaScript objects are unnecessary collections of "named values". JavaScript also defines a special object - an array, which represents an ordered collection of numbered values. JavaScript defines a special syntax for arrays. Make arrays have some unique behavioral properties that are different from ordinary objects.
Javascript also defines a special object-function. A function is an object that has executable code associated with it. The function is called to run the executable code and return the result of the operation. Like arrays, functions behave differently from other objects. JavaScript defines a special syntax for using functions. For javascript functions. Most importantly, they are all true values, and JavaScript can treat them like ordinary objects.
If a function initializes (using the new operator) a new object, we call it a constructor. Each constructor defines a class of objects—a collection of objects that the constructor initializes. Classes can be thought of as subtypes of object types. In addition to the array class and function class, JavaScript also defines three other useful classes. Date defines an object that represents a date. Regular (regExp) object defines a regular expression. The error class defines objects that represent runtime errors and syntax errors in JavaScript programs. You can define the required classes by defining your own constructor.
The JavaScript interpreter has its own memory management mechanism that can automatically perform garbage collection on memory. This means that the program can create objects on demand, and the programmer does not have to worry about the destruction of these objects and memory recycling. When there is no longer any reference to an object, the interpreter knows that the object is no longer useful, and will automatically reclaim the memory resources it occupies.
JavaScript is an object-oriented language. Loosely speaking, this means that we do not need to globally define functions to operate different types of values. The data type itself can define methods to use values. For example, to sort the elements in an array a, there is no need to pass a Enter the sort() function, but call a method sort()
a.sort(); //Object-oriented version of sort(a)
Technically, only JavaScript objects can have methods. However, numbers, strings, and Boolean values also have their own methods. In JavaScript, only null and undefined are values that cannot have methods.
Javascript types can be divided into primitive types and object types, and can be divided into types that can have methods and types that cannot have methods. It can also be divided into mutable and immutable types. The value of a variable type can be modified. Objects and arrays are variable types: JavaScript programs can change the attribute values of objects and the values of array elements.
Numbers, Boolean values, null and undefined are immutable types. For example, modifying the contents of an array doesn't make sense in itself. A string can be thought of as an array of characters, and you can think of it as changeable. However, in JavaScript, strings are immutable. You can access the text at any position in the string, but JavaScript does not provide a method to modify the text content of the string.
Javascript can freely perform data type conversion. For example, if a number is used where the program expects a string, JavaScript will automatically convert the number to a string. If a non-Boolean value is used where a Boolean value is expected, JavaScript will convert accordingly. Flexible type grabbing and swapping rules in JavaScript for "equality"
Javascript variables are untyped. Variables can be assigned human and type values. Use the var keyword to declare variables. JavaScript uses syntactic scope. Variables that are not declared within any function are called global variables, which are visible anywhere in the JavaScript program.
1. Numbers
Unlike other programming languages, JavaScript does not distinguish between integer values and floating point values. Numerical values in JavaScript are represented by floating point values. When a number appears directly in a JavaScript program, we call it a numeric literal. JavaScript supports numeric literals in multiple formats. (Note: Adding a minus sign (-) directly before any number gives you their negative value) But the minus sign is the unary negation operator. , is not part of the numeric literal grammar. )
i integer direct quantity
Use an array sequence to represent a decimal integer in JavaScript
In addition to decimal integer literals, JavaScript also recognizes values based on the hexadecimal mechanism (16) as the base. The so-called hexadecimal number is prefixed with "0X" or "0x", followed by the direct quantity of the hexadecimal number string. Hexadecimal values are composed of numbers 0-9 and letters between a(A)-f(F). The letters a-f represent the numbers 10-15. The following is an example of a hexadecimal integer literal
0xff //15*16 15=255
0xCAFE911
Although ECMAScript does not support octal literals, some implementations of JavaScript can allow integers to be represented in octal (base 8) form. Octal literals start with the number 0, followed by a sequence of digits between 0 and 7.
0377 // 3*64 7*8 7 =255 (decimal)
Since some javascript implementations support octal scalars and some do not, it is best not to use integer scalars prefixed with 0. After all, we have no way of knowing whether the current javascript implementation supports octal. parse. In the strict mode of ECMAScript 6, octal literals are explicitly prohibited.
ii. Floating point literal
Floating-point literals can contain decimal points, and they use the traditional writing method of real numbers. A real number consists of an integer part, a decimal point, and a decimal part.
In addition, you can also use exponential notation to represent floating-point literals. That is, the real number is followed by the letter E or e, followed by a positive or negative sign, and then an integer exponent. The value represented by this counting method is the exponential power of the previous real number multiplied by 10.
A more concise syntax can be used to represent
[digits][.digits][(E|e)[( |-)]digits]
3.14
2345.455
.33333333333333333
6.02e23 //6.02*10 to the 23rd power
1.255454E-23 //1.255454*10 to the power of negative 23
Arithmetic operations in iii.javascript
Javascript programs use arithmetic operators provided by the language to perform numerical operations. These operators include - * / and the remainder (remainder after division) operator %
In addition to basic operators, JavaScript also supports more complex arithmetic operations. This complex operation is implemented through functions and constants defined as properties of the Math object.
Math.pow(2, 53) //=>9007199254740992 document.write(Math.pow(2,53) )
Math.round(.6) //=>1.0 Rounding
Math.ceil(.6) //=>1.0 round up
Math.floor(.6) //=>0.0 round down
Math.abs(-5) //=>5 Find the absolute value
Math.max(x, y, z) //Return the maximum value
Math.min(x, y, z) //Return the minimum value
Math.random() //Generate a pseudo-random number greater than 0 and less than 1
Math.PI //Pi
Math.E //e: The base of natural logarithms
Math.sqrt(3) //The square root of 3
Math.pow(3, 1 / 3) //Cube root of 3
Math.sin(0) //Trigonometric functions, as well as Math.cos, Math.atan, etc.
Math.log(10) //=>2.302585092994046 The natural logarithm of base 10
Math.log(512) / Math.LN2 //The logarithm of 512 with base 2
Math.log(100) / Math.LN10 //The logarithm of 100 with base 10
Math.exp(3) //The third power of e
Arithmetic operations in JavaScript will not report errors when overflowing, underflowing, or dividing by zero. However, the numerical operation result exceeds the upper limit of the number that can be expressed in JavaScript (overflow), and the result is a special infinite value (infinty) value, which is represented by infinty in JavaScript. Similarly, when the value of a negative number exceeds the range of negative numbers that JavaScript can express, the result is negative infinity, which is represented by -Infinty in JavaScript. Infinite values behave as we would expect: addition, subtraction, multiplication, and division based on them result in infinity (signs preserved)
Underflow is a situation that occurs when the result of an operation is infinitely close to zero and smaller than the minimum value that JavaScript can represent. When a negative number underflows, JavaScript returns a special value, "negative zero", which is almost exactly the same as normal zero. JavaScript programmers rarely use negative zero.
Javascript predefines the global variables Infinaty and NaN, which are used to express positive infinity and non-numeric values. In ECMAScipt3, these two values can be read and written. ECMAScript5 fixes this problem by defining them as read-only. The property values defined by the Number object in ECMAScipt3 are also read-only. Here are some examples:
Infinity //Initialize a read/write variable to infinite
Number.POSITIVE_INFINITY //Same value, read-only
1 / 0 //This is also the same value
Number.MAX_VALUE 1 //The calculation result is still Infinity
Number.NEGATIVE_INFINITY //Represents negative infinity
-Infinity
-1/0
-Number.MAX_VALUE -1
Number.NaN //Same value, but read-only
//The calculation result is still NaN
Number.MIN_VALUE/2 //Underflow occurred. The calculation result is 0
-Number.MIN_VALUE/2 //Negative zero
-1/Infinity //Negative zero
-0
The negative zero value is also somewhat special. It is equal to positive and negative zero (even judged using JavaScript's strict equality test), which means that the two values are almost identical, except as a divisor:
Zero === negz //=>true Positive and negative zero values are equal
1/zero === 1/negz //false Positive infinity and negative infinity are not the same
There are countless real numbers, but JavaScript can only represent a limited number in the form of floating point numbers (18 437 736 874 454 810 627 to be exact). In other words, when real numbers are used in JavaScript, they often It is only an approximate representation of the true value.
Javascript uses IEEE-754 floating point number representation (used by almost all modern programming languages). This is a binary representation that can accurately represent fractions, such as 1/2 1/8 and 1/1024. Unfortunately, the fractions we often use, especially in financial calculations, are all decimal fractions 1/10 ,1/100 etc. Binary representation cannot represent simple numbers like 0.1.
Numbers in javascript have sufficient precision. And can be close to 0.1. But the fact that the number cannot be expressed accurately brings some problems.
alert(x == y) //=>false The two values are not equal
x == .1 //=>false .3-.2 is not equal to .1
y == .1 //=>true .2-.1 is equal to 1
Due to rounding error, the approximate difference between 0.3 and 0.2 is not actually equal to the approximate difference between 0.2 and 0.1 (in a real simulation environment, 0.3-0.2=0.099 999 999 999 999 98). This problem is not unique to JavaScript, but it is important to understand that it occurs in any programming language that uses binary floating point numbers. Also note that the values of x and y in the above code are very close to each other and the final correct values. This calculation result can be used for most computing tasks. This problem only occurs when comparing two values for equality.
Future versions of JavaScript may support decimal number types to avoid this problem, but until then you may prefer to use large integers for important financial calculations. For example, use integer "cents" instead of decimal "dollars" for monetary unit-based operations.
iiiii.Date and time
The core of the JavaScript language contains the Date() constructor, which originally creates date and time objects. The methods of these date objects provide a simple API for date calculations. Date objects cannot be basic data types like numbers.
var zhen = new Date(2011, 0, 1); //January 1, 2011
var later = new Date(2011, 0, 1, 17, 10, 30); //Same day
var now = new Date(); //Current date and time
var elapsed = now - zhen; //Date subtraction. Calculate the number of milliseconds in the interval
later.getFullYear(); //=>2011
later.getMonth(); //=>0 Month starting from 0
later.getDate(); //=>1 The number of days starting from 1
later.getDay(); //=>5 Get the day of the week. 0 represents Sunday, 5 represents Monday 1
later.getHours() //=>Local time
later.getUTCHours() //Use UTC to represent hours, based on the time zone.
2. Text
A string is an immutable ordered sequence of 16-bit values. Each character usually comes from the Unicode character set. JavaScript represents text through the string type. The length of a string is the number of 16-bit values it contains. Javascript strings (and arrays thereof) are indexed starting from 0. The length of an empty string is 0, and there is no "character type" that represents a single character in JavaScript. To represent a 16-bit value, just assign it to a string variable. The length of this string is 1.
Character set, internal code and javascript string
JavaScript uses the UTF-16 encoded Unicode character set. A JavaScript string is a sequence composed of a set of unserialized 16-bit values. The most commonly used Unicode characters are represented by 16-bit internal codes and represent a single character in a string. Unicode characters that cannot be represented as 16-bit characters follow UTF-16 encoding rules - use two 16-bit characters. Values are represented as a sequence (also called a "surrogate pair"). This means that a JavaScript string of length 2 (two 16-bit values) may represent a Unicode character.
var p ="π" ; //π is represented by 16-bit internal code 0x03c0
var e = "e"; //e is represented by a 17-bit internal code 0x1d452
p.length // =>1 p contains a 16-bit value
e.length // =>2 e contains two values after UTF-16 encoding: "ud835udc52"
The various string operation methods defined by JavaScript all operate on 16-bit values, not characters, and surrogate pairs will not be processed separately. Similarly, JavaScript does not perform standardized processing on strings. There is no guarantee that the string is even in legal UTF-16 format
i string literal
A string literal in a JavaScript program is a character sequence enclosed by single quotes or double quotes. A string delimited by single quotes can contain double quotes, and a string delimited by double quotes can also contain Can contain single quotes. Here are a few examples of string literals.
"" //Empty string, 0 characters
'testing'
"3.14"
'name="myform"'
"Wouldn't you prefer O'Reily's book?"
In ECMAScript3, string literals must be written in one line, but in ECMAScript5, string literals can be split into several lines, and each line must end with a backslash (), a backslash and a line terminator None of them are the contents of string literals. If you want them together you can use Escape characters.
It should be noted that when using single quotes to delimit strings, you need to be extra careful about abbreviations and all formatting in English. The English apostrophe and single quote are the same character, so you must use backslash () escape.
ii escape character
In JavaScript strings, backslash () has a special purpose. Adding a character after the backslash no longer represents their literal meaning. For example, n is an escape character, which represents a newline character. .
o //NUL character
b //Backspace character
t //Horizontal tab character
n //Line break character
v //Vertical tab character
F // Perspage Character
\ Backslash
xXX Latin-1 character specified by two hexadecimal digits
xXXXX Unicode characters specified by four hexadecimal XXXX characters
One of the built-in functions of JavaScript is string concatenation. Using the operator with strings means string concatenation. For example
s.length
In addition to the length attribute, strings also provide many methods that can be called.
s.charAt(s.length - 1) //"d"The last character
s.substring(1, 4) //"ell" 2-4 characters
s.slice(1, 4) //ell Same as above
s.slice(-3) // The last 3 characters
s.indexOf(l ")//The position where the 2 characters l first appears
s.lastIndexOf("l") //10 The position where the character l last appeared
s.indexOf("l",3)//After position 3, the position where the l character first appears
s.split(",") //=> ["hello", "world"] is separated into substrings
s.replace("h","H")// =>"Hllo,world" full text character replacement
s.toUpperCase() //=>"HELLO,WORLD"
In JavaScript, strings are fixed. Methods like replace() and toUpperCase() return new strings, and the original characters themselves do not change.
In ECMAScript, characters can be treated as read-only arrays. In addition to using the charAt() method, you can also use square brackets to access individual characters in a string. (16-bit value)
s = "hello,world"
s[0] //=>"h"
s[s.length-1] //=>"d"
Foxfire has supported string indexing in this way a long time ago, and most modern browsers (except IE) have followed Mozailla's footsteps and completed this feature before ECMAScript took shape
iiii pattern matching
Javascript defines the RegExp() constructor, which is used to create objects that represent text pattern matching. These patterns are called "regular expressions" (regular expressions), the regular expression syntax in JavaScript Caiyang Perl. Both the String and RegExp objects define functions for pattern matching and search and replacement using regular expressions.
The RegExp object is not a basic data type in the language. Like Date, it is just a special object with a practical API. The syntax of regular expressions is complex and the API is rich. It will be introduced in detail in Chapter 10. RegExp is a powerful and commonly used text processing tool, this is just an overview.
Although RegExp is not a basic data type in the language, they still have a direct way of writing and can be used directly in JavaScript. The text between two slashes forms the literal of a regular expression. The second slash can also be followed by one or more letters. Used to modify the meaning of matching patterns. For example:
/^HTML/ // Match strings starting with HTML
/[1-9][0-9]*/ // Match a non-zero number, followed by any number of numbers
/bjavascriptb/i/ // Match the word javascript and ignore case
The RegExp object defines many useful methods, and strings also have methods that can accept RegExp parameters. For example:
var text = "testing:1,2,3"; //Text example
var pattern = /d /g / //Match all instances containing one or more digits
pattern.test(text) // =>true: Match successful
text.search(pattern) //=>9: The position of the first successful match
text.match(pattern) //=> ["1", "2", "3"] All matches form an array
text.repeat(pattern,"#"); //=>"testing:#,#,#"
text.split(/D /); //=>["","1","2","3"]: Use non-numeric characters to intercept the string
3.Boolean value
Boolean value refers to true or false, on or off. This type has only two values, the reserved words true or false
The results of comparison statements in JavaScript are usually Boolean values. For example
a==4
This code is used to detect whether the value of variable a is equal to 4. If equal, the value is true, if not, the value is false
Boolean values are usually used in JavaScript control statements, such as if/else statements in JavaScript. If the Boolean value is true, execute the first piece of logic, and if it is false, execute another piece of code, such as
If (a == 4)
b = b 1;
else
a = a 1;
Any JavaScript value can be converted into a Boolean value. The following values are converted into false
undefined
null
0
-0
NaN
""//Empty string
All other values, including all objects (arrays) will be converted to true, false and the above 6 values that can be converted to false are sometimes called "false values". When JavaScript expects to use a boolean value, the false value will Treated as false, true value will be treated as true
Let’s look at an example. Adding that the variable o is an object or null, you can use an if statement to detect whether o is a non-null value.
if(o!==null)...
The inequality operator "!==" compares o and null and the result is true or false. You can ignore the comparison statement here first, null is a false value, and the object is a true value.
if(o)...
For the first case, the code after if will be executed as long as o is not null. The restrictions in the second case are not so strict. This if is executed only if o is not false or any false value (such as null or unfined).
Boolean contains the toString() method, so you can use this method to convert a string to "true" or "false", but it does not contain other useful methods. In addition to this unimportant API, there are three important ones Boolean operator.
&& operator, || operator and unary operator "!" perform Boolean NOT (NOT) operation. If the true value returns false, the false value returns true, such as
If ((x == 0 && y == 0) || !(z == 0)) {
// x and y are both zero or z is non-zero
}
4.null and undefined
Null is a keyword in the JavaScript language. It represents a special value "null value". For null, typeof() operation is performed and object is returned. In other words, null can be considered as a special object value, which means " non-object". But in fact, null is usually considered to be the only member of its free type. It can represent numbers, strings, and objects that are "valueless". Most programming languages contain nulls like JavaScript, and you may be familiar with null or nil.
Javascript also has a second value indicating the vacancy of the value. Used to represent deeper "null values". It is a value of a variable. Indicates that the variable is not initialized. If you want to query the value of an object property or array element and return undefined, it means that the property or element does not exist. undefined is a predefined global variable (it is different from null, it is not a keyword), and its value is undefined. If typeof is used to test for an undefined type, "undefined" is returned, indicating that this value is the only member of this type.
Although null and undefined are different, they both represent "a vacancy of value" and the two are often interchangeable. The equality operator "==" considers the two to be equal (the strict equality operator "===" must be used to distinguish them). Where a value is expected to be of type Boolean, their value is false. Similar to false. Both null and undefined do not contain any properties or methods. In fact, using "." and "[]" to access members or methods of these two values will generate a type error.
You may think that undefined represents system-level, unexpected or error-like value vacancies, and null represents program-level, normal or expected value vacancies. If you want to copy them Variables or properties, or pass them into functions as parameters, null is the best choice.
5. Global object
The previous sections discussed JavaScript element types and primitive values. Object types - objects, arrays and functions/But there is a very important class of objects that must be made clear now: global objects
Global objects play an important role in JavaScript. Properties of global objects are globally defined symbols. JavaScript programs can be used directly. When the JavaScript interpreter starts, it creates a new global object and gives it a defined set of initial properties.
Global properties such as undefined Infinty and NaN
Global functions such as isNaN(), parseInt() and eval()
Constructors such as Date(), RegExp(), String(), Object() and Array()
Global objects, such as Math and JSON
Initial properties of global objects are not reserved words, but they should be treated as such.
At the top level of the code - javascript code that is not within any function, the global object can be referenced through the javascript keyword.
var global = this; //Define a global variable that refers to the global object.
In client-side JavaScript, the window object acts as a global object. This global window object has a familiar window reference to itself. It can replace this to refer to the global object. Window defines the global core properties. But it also defines some other global properties for web browsers and interactive JavaScript.
When first created, the global object defines all the predefined global values in JavaScript. This special object also contains global values defined for the program. If the code declares a global variable. This global variable is a property of the global object.
6. Packaging object
A JavaScript object is a composite value: it is a collection of properties or named values. The attribute value is referenced through ".". When the attribute value is a function, it is a method, and the method in the object o is dispatched through o.m().
We see that strings also have properties and methods.
var s="hello world";
var word = s.substring(s.indexOf("") 1,s.length);//Use the attributes of the string.
document.write(word) //"ello world"
Since a string is not an object, why does it have attributes? As long as the properties of string s are referenced, JavaScript will convert the value of the string into an object by calling new String(s). This object inherits the methods of string. and is used to handle property references. Once the new attribute is referenced. Once the reference ends, the newly created object will be destroyed. (This temporary object is not actually created or destroyed, however the process looks like this.)
Like strings, numbers and Boolean values also have their own methods, creating a temporary object through the Number() and Boolean() constructors. The calls to these methods all come from this temporary object. (null and undefined are not wrapped objects, and accessing their properties will result in a type error)
Look at the following codes and think about their execution process
var s = "test";
s.len = 4; //Set an attribute for it
var t = s.len //Find this attribute
When this code is run, the value of t is undefined. The second line of code creates a temporary string object and gives the value of len as 4, then destroys the object. The third line of code uses the original (unmodified) ) creates a new string object and tries to read the len property.
This attribute naturally does not exist, indicating that the result is undefined. This code illustrates that when reading the attribute values (or methods) of strings, arrays, and Boolean values, it behaves like an object, but if you try to assign a value to its attribute . This operation is ignored; the modification only occurs on the temporary object. This temporary object is not retained.
It should be noted that you can create wrapper objects explicitly through the String(), Number(), and Boolean() constructors:
var s = "test",
n = 1,
b = true;
var S = new String(s);
var N = new Number(n);
var B = new Boolean(b);
Javascript will convert the wrapper to a primitive value when necessary, so the object S N B in the above code often - but not always - behaves the same as s n b. The "==" equals operator converts the original value and Their wrapped objects are treated as equal.
But the "===" operator treats them as unequal, and the typeof operator can see the difference between the original value and the object it wraps.
7. Immutable primitive values and mutable object references.
Javascript's primitive values (undefined null Boolean values, numbers and strings) are fundamentally different from objects (including arrays and functions). The primitive value cannot be changed; no method can (or mutate) a primitive value. This is obviously true for numbers and Boolean values - changing the value of a number itself doesn't make sense, but it's less obvious for strings, which look like arrays of characters. We expect that the characters in the string can be modified by the specified index. In fact, JavaScript prohibits this. All methods in string appear to return a modified string, but actually return a new string.
var s = "hello world";
s.toUpperCase(); //Returns "HELLO WORLD" and does not change the value of s
s //=> "hello world" The original string has not changed
The comparison of original values is a comparison of values. They are equal only when their values are equal. This sounds a bit difficult for numbers, booleans, null and undefined, and there is no other way to compare them. Again, it's less obvious for strings; if you compare two separate strings, JavaScript considers them equal if and only if their lengths and characters at each index are equal.
var o = {x:1} //Define an object
o.x = 2 //Change the object by modifying its properties
o.y = 3 //Change this object again and add a new attribute to it
var a =[1,2,3] //Arrays can also be modified
a[0]=0; //Change an element in the array
a[3]=4; Add a new element to the array
Comparison of objects is not comparison of values: even if two objects contain the same attributes and the same values, they are not equal, and two arrays with exactly equal index elements are not equal
var o ={x:1}, p={x:1}//Two objects with the same attributes
o === p ;//=>false Two separate objects are never equal ( o == p ; =>false)
var a =[],b=[]; //Two separate empty arrays
a === b; //=>false Two separate arrays are never equal
We usually call objects reference types to distinguish them from the basic types of JavaScript. According to the terminology, objects are references, and comparisons of objects are comparisons of references; they are only equal when and when they use the same base object.
var a = []; //Define a variable a
that refers to an empty array var b = a; //Variable b refers to the same array
b[0] = 1;
a[0] //=>1 Variable a will also be modified
a === b //=>true a and b refer to the same array, so they are equal.
Just like the code you just saw above, assigning an object (or array) to a variable is just a reference value assigned: the object itself is not copied once.
If you want to get a copy of an object or array, you must explicitly copy every property of the object or every element of the array. The following example completes the copy of the array through a loop.
var a = ['a', 'b', 'c']; //array to be copied
var b = []; //Copy to the empty array of the target
for (var i = 0; i b[i] = a[i]; //Copy the element to b.
}
Similarly, if we want to compare two individuals or arrays, they must compare their attributes or elements. The following code defines a function that compares an array.
function equalArrays(a, b) {
If (a.length != b.length) return false; //Two arrays with different lengths are not equal
for (var i = 0; i If (a[i] !== b[i]) return false; //If any elements are not equal, the arrays are not equal
return true; // Otherwise they are equal
}
8. Type conversion
Value types in JavaScript are very flexible. We have already seen this with Boolean values: when JavaScript expects a Boolean value, you can provide any type of value. JavaScript will convert the type itself as needed. Some values (truth values) are true, and other values (false values) are converted to false. The same applies to other types. If JavaScript expects a string, it converts the given value to a string. If JavaScript expects an array, it converts the given value to a number (returning NaN if the conversion result is meaningless), some examples are as follows:
10 "object" //=> "10object";
"7" * "4" // =>28 Both strings are converted into numbers
var n = 1 - "x" // =>NaN string x cannot be converted to a number
n " objects" // =>"NaN objects": NaN is converted to the string "NaN"
The following table explains how to perform type conversion in JavaScript. Bold highlights types that may surprise you. Empty cells represent conversions that are unnecessary and not performed.
Value | Convert to string | Number | Boolean value | Object |
undefined null |
"undefined" "null" |
NaN 0 |
false false |
throws TypeError throws TypeError |
true false |
"ture" "false" |
1 0 |
new Boolean(true) new Boolean(false) |
|
""(空字符串) "1.2"(非空,数字) "one"(非空,非数字) |
0 1.2 NaN |
false true true |
new String("") new String("1.2") new String("one") |
|
0 -0 NaN Infinty -Infinty 1(infinity, non-zero) |
"0" "0" "NaN" "Infinity" "-Infinity" "1" |
false false false true true true |
new Number(0); new Number(-0); new Number(NaN) new Number(Infinty) new Number(-Infinty) new Number(1) |
|
{}(any object) [](arbitrary array) [9](1 numeric element) ['a'](other array) function(){}(arbitrary function) |
Refer to the third section of this section "" "9" Use join() method Please refer to the third section of this section |
Refer to the third section of this section 0 9 NaN NaN |
true true true true true |
The conversion from original value to original value mentioned in the above table is relatively simple. We have already discussed the conversion to Boolean value in the third section of this article. The conversion of all primitive values to strings is also clearly defined. The conversion to numbers is more subtle. Strings represented as numbers can be converted directly to numbers, with spaces at the beginning and end allowed. However, any non-null characters at the beginning and end will not be regarded as part of the number, causing the result of the string to be NaN. There are some number conversions that look strange: true is converted to 1, false, and the empty string "" is converted to 0.
The conversion of primitive values to objects is also very simple, primitive values
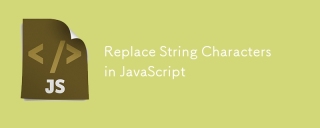
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
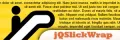
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
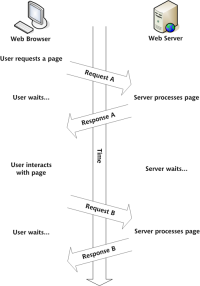
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
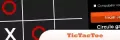
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
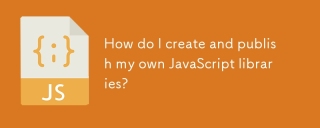
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
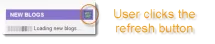
This tutorial demonstrates creating dynamic page boxes loaded via AJAX, enabling instant refresh without full page reloads. It leverages jQuery and JavaScript. Think of it as a custom Facebook-style content box loader. Key Concepts: AJAX and jQuery
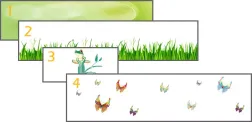
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
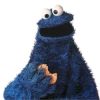
This JavaScript library leverages the window.name property to manage session data without relying on cookies. It offers a robust solution for storing and retrieving session variables across browsers. The library provides three core methods: Session


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
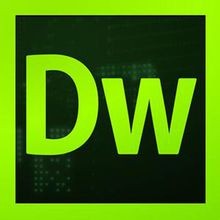
Dreamweaver CS6
Visual web development tools
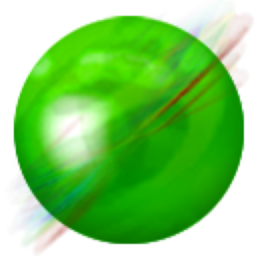
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
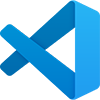
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
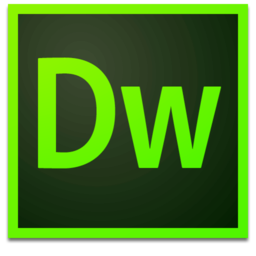
Dreamweaver Mac version
Visual web development tools
