This JavaScript library leverages the window.name
property to manage session data without relying on cookies. It offers a robust solution for storing and retrieving session variables across browsers. The library provides three core methods: Session.set()
, Session.get()
, and Session.clear()
, along with a debugging utility, Session.dump()
.
The library's functionality is demonstrated on a dedicated webpage. The code integrates seamlessly, loading just before the closing body tag. It begins by including a JSON library for cross-browser compatibility in serialization. The core session.js
file is then loaded; it's independent of other libraries like jQuery.
The Session
object is defined only if the JSON library is available and no naming conflicts exist. It uses window.top
(or window
as a fallback) to access the session storage. Existing data in window.name
is parsed and loaded into an internal store
object; otherwise, an empty object is created.
A private Save()
function serializes the store
object and saves it to window.name
upon page unload. Cross-browser event listeners ensure this function executes reliably across different browsers. The serialization and saving process is deferred until the page unloads to minimize performance impact.
The public methods (set
, get
, clear
, and dump
) provide a simple interface for interacting with the session data. Session.get()
returns undefined
if a requested session variable is not found.
This library provides a practical and efficient alternative to cookie-based session management in JavaScript. Its independence from other libraries and cross-browser compatibility make it a versatile tool for various web development projects.
Further Resources:
- JavaScript Session Variables Demonstration Page
- Full JavaScript
session.js
Code - Download Full Code (ZIP)
Frequently Asked Questions (FAQs) about using sessionStorage
(Note: The library uses window.name
, not sessionStorage
):
The original article also includes a FAQ section about sessionStorage
. While this library doesn't use sessionStorage
, the FAQ provides valuable information about client-side storage in general. Here's a summarized version:
-
Accessing
sessionStorage
: UsesessionStorage.getItem("key")
. -
Setting
sessionStorage
: UsesessionStorage.setItem("key", "value")
. -
localStorage
vs.sessionStorage
:localStorage
persists across sessions, whilesessionStorage
is cleared when the tab closes. -
Storing Objects/Arrays: Use
JSON.stringify()
to store andJSON.parse()
to retrieve. -
Clearing
sessionStorage
: UsesessionStorage.clear()
. -
Security:
sessionStorage
is not encrypted; avoid sensitive data. - Browser Support: Widely supported in modern browsers.
- Storage Limits: Typically 5-10MB.
-
sessionStorage
and Cookies: Serve different purposes;sessionStorage
is client-side only. -
Browser Crashes: Data is lost on crashes. Consider
localStorage
for persistent storage.
Remember to replace the bracketed placeholders ([https://www.php.cn/link/35068fbf1ec706142e1f75fa23ee1995], [https://www.php.cn/link/a80ff02f8227904e65413f89ee1719e6], [https://www.php.cn/link/66a1942cfad91ff0ee99daf86e674d55]) with the actual links if available.
The above is the detailed content of How to Write a Cookie-less Session Library for JavaScript. For more information, please follow other related articles on the PHP Chinese website!
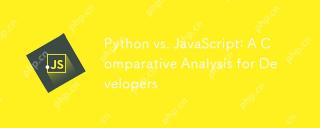
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
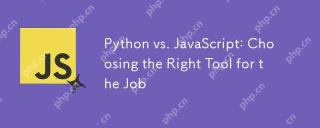
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
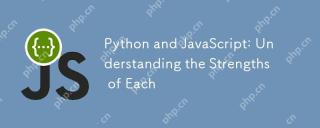
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
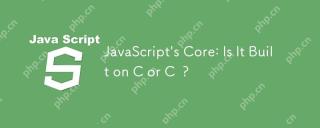
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
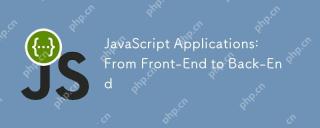
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
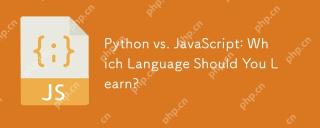
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
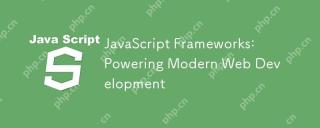
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
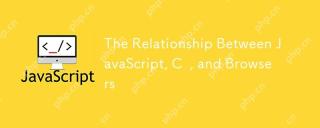
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
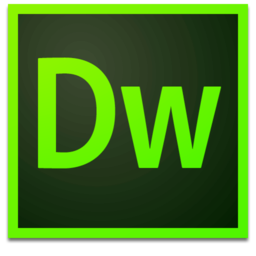
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
