


Elegant way to calculate array intersection and union using lambda function in PHP
In PHP, lambda functions can be used as an elegant way to handle the intersection and union of arrays. For intersections, use the array_filter() function with the lambda function to filter elements to determine whether they exist in another array; for unions, use the array_reduce() function with the lambda function to merge unique elements between arrays. These methods simplify calculations and improve code flexibility and readability.
The elegant way to calculate the intersection and union of arrays using the lambda function in PHP
The lambda function in PHP, also known as Anonymous functions provide a convenient way to manipulate and transform arrays. By taking advantage of their simplicity, we can implement more complex array operations with less code.
Calculate array intersection
Array intersection is a set of elements that exist simultaneously in two or more arrays. We can calculate the intersection using the array_intersect()
function, but this can be done more elegantly using a lambda function.
<?php $array1 = [1, 2, 3, 4, 5]; $array2 = [3, 4, 5, 6, 7]; $intersection = array_filter($array1, function($value) use ($array2) { return in_array($value, $array2); }); print_r($intersection); ?>
Output:
Array ( [2] => 3 [3] => 4 [4] => 5 )
Calculate the union of arrays
The union of arrays is the set of all elements in two or more arrays. We can compute the union using the array_merge()
function, but the lambda function provides a more flexible approach.
<?php $array1 = [1, 2, 3, 4, 5]; $array2 = [3, 4, 5, 6, 7]; $union = array_reduce($array1, function($result, $value) use ($array2) { if (!in_array($value, $array2)) { $result[] = $value; } return $result; }, $array2); print_r($union); ?>
Output:
Array ( [0] => 3 [1] => 4 [2] => 5 [3] => 6 [4] => 7 [5] => 1 [6] => 2 )
Practical case
Consider an e-commerce website where you need to calculate the intersection of all items in the user's shopping cart to determine Items purchased together. You can do this easily using lambda functions:
<?php $user1Cart = [1, 2, 3, 4, 5]; $user2Cart = [3, 4, 5, 6, 7]; $commonItems = array_filter($user1Cart, function($value) use ($user2Cart) { return in_array($value, $user2Cart); }); // 展示共同购买的商品 foreach ($commonItems as $item) { echo $item . " "; } ?>
Output:
3 4 5
The above is the detailed content of Elegant way to calculate array intersection and union using lambda function in PHP. For more information, please follow other related articles on the PHP Chinese website!
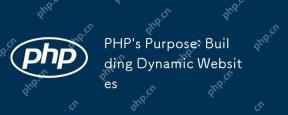
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
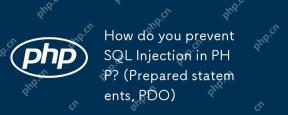
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
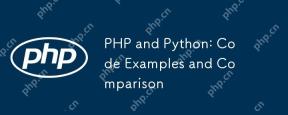
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
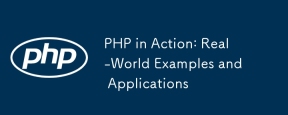
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
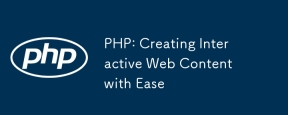
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
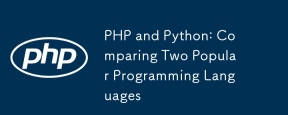
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
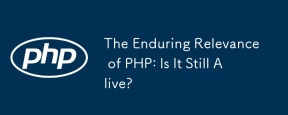
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
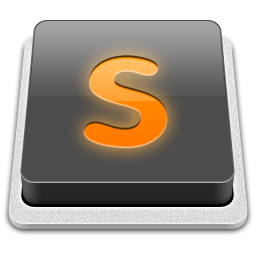
SublimeText3 Mac version
God-level code editing software (SublimeText3)