PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
introduction
Have you ever been curious about the application scenarios of PHP in reality? PHP is not only the preferred programming language for beginners, but also the core technology of many large websites and applications. Today, we will explore the application of PHP in actual projects in depth, from basic to advanced, and take you to appreciate the charm of PHP. Through this article, you will learn how PHP can show off its skills in e-commerce, content management systems, API development and other fields, and master some practical skills and best practices.
PHP basic review
PHP, full name Hypertext Preprocessor, is a programming language widely used in server-side scripting languages. It can be embedded in HTML and is very suitable for web development. PHP's syntax is simple, easy to learn and use, which makes it the first choice for many developers.
In PHP, you can easily process form data, manage databases, generate dynamic web pages, etc. Let's review the basic usage of PHP with a simple example:
<?php $name = "John"; echo "Hello, " . $name . "!"; ?>
This code shows how to declare variables and output strings using echo
. Simple and effective, right?
Application of PHP in e-commerce
E-commerce is an area where PHP shows its strengths. Many well-known e-commerce platforms, such as Magento and Shopify, are developed based on PHP. Let's take a look at the specific application of PHP in e-commerce.
Shopping cart function
Shopping cart is one of the core functions of e-commerce websites. PHP can easily process users' shopping cart data, and implement operations such as adding, deleting, and updating products. Here is a simple shopping cart implementation:
<?php session_start(); if (!isset($_SESSION['cart'])) { $_SESSION['cart'] = array(); } if (isset($_POST['add_to_cart'])) { $product_id = $_POST['product_id']; $quantity = $_POST['quantity']; if (isset($_SESSION['cart'][$product_id])) { $_SESSION['cart'][$product_id] = $quantity; } else { $_SESSION['cart'][$product_id] = $quantity; } } // Show cart content foreach ($_SESSION['cart'] as $product_id => $quantity) { echo "Product ID: $product_id, Quantity: $quantity<br>"; } ?>
This example shows how to use sessions to manage shopping cart data. In this way, users can keep the cart in their state between different pages.
Payment Processing
Payment processing is another key feature of e-commerce websites. PHP can be integrated with various payment gateways, such as PayPal, Stripe, etc. Here is a simple PayPal payment processing example:
<?php require 'vendor/autoload.php'; $apiContext = new \PayPal\Rest\ApiContext( new \PayPal\Auth\OAuthTokenCredential( 'YOUR_CLIENT_ID', // ClientID 'YOUR_CLIENT_SECRET' // ClientSecret ) ); $payer = new \PayPal\Api\Payer(); $payer->setPaymentMethod('paypal'); $amount = new \PayPal\Api\Amount(); $amount->setTotal('10.00') ->setCurrency('USD'); $transaction = new \PayPal\Api\Transaction(); $transaction->setAmount($amount); $redirectUrls = new \PayPal\Api\RedirectUrls(); $redirectUrls->setReturnUrl("http://example.com/return") ->setCancelUrl("http://example.com/cancel"); $payment = new \PayPal\Api\Payment(); $payment->setIntent('sale') ->setPayer($payer) ->setTransactions(array($transaction)) ->setRedirectUrls($redirectUrls); try { $payment->create($apiContext); echo $payment->getApprovalLink(); } catch (\PayPal\Exception\PayPalConnectionException $ex) { echo $ex->getMessage(); } ?>
This example shows how to use PayPal's REST API to process payments. In this way, you can easily integrate payment features into your e-commerce website.
Application of PHP in content management system
Content Management Systems (CMS) is another area where PHP is shining. Well-known CMS such as WordPress, Drupal and Joomla are all developed based on PHP. Let's take a look at the specific application of PHP in CMS.
Dynamic content generation
A core function of CMS is to generate content dynamically. PHP can easily read data from a database and generate dynamic web pages. Here is a simple example of dynamic content generation:
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; // Create a connection $conn = new mysqli($servername, $username, $password, $dbname); // Check the connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } $sql = "SELECT id, title, content FROM posts"; $result = $conn->query($sql); if ($result->num_rows > 0) { // Output data while($row = $result->fetch_assoc()) { echo "<h2 id="row-title">" . $row["title"]. "</h2>"; echo "<p>" . $row["content"]. "</p>"; } } else { echo "0 results"; } $conn->close(); ?>
This example shows how to read article data from a database and generate web content dynamically. This way, you can easily manage and present a large amount of content.
User Management
User management is another important feature of CMS. PHP can easily handle user registration, login, permission management and other operations. Here is a simple user registration example:
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; // Create a connection $conn = new mysqli($servername, $username, $password, $dbname); // Check the connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } if ($_SERVER["REQUEST_METHOD"] == "POST") { $username = $_POST['username']; $password = $_POST['password']; $sql = "INSERT INTO users (username, password) VALUES ('$username', '$password')"; if ($conn->query($sql) === TRUE) { echo "New record insertion successfully"; } else { echo "Error: " . $sql . "<br>" . $conn->error; } } $conn->close(); ?>
This example shows how to handle user registration and store user data into a database. In this way, you can easily manage user information.
Application of PHP in API development
API development is an important part of modern web development. PHP can easily develop RESTful APIs to meet various application needs. Let's take a look at the specific application of PHP in API development.
RESTful API Development
RESTful API is a common API design style. PHP can use a variety of frameworks and libraries to develop RESTful APIs. Here is a simple RESTful API example using the Slim framework:
<?php use \Psr\Http\Message\ServerRequestInterface as Request; use \Psr\Http\Message\ResponseInterface as Response; require 'vendor/autoload.php'; $app = new \Slim\App; $app->get('/hello/{name}', function (Request $request, Response $response, array $args) { $name = $args['name']; $response->getBody()->write("Hello, $name"); return $response; }); $app->run(); ?>
This example shows how to develop a simple RESTful API using the Slim framework. In this way, you can easily handle various HTTP requests and return the corresponding data.
API security
API security is an important aspect of API development. PHP can use various methods to ensure the security of the API, such as authentication, encryption, etc. Here is a simple API authentication example:
<?php use \Psr\Http\Message\ServerRequestInterface as Request; use \Psr\Http\Message\ResponseInterface as Response; require 'vendor/autoload.php'; $app = new \Slim\App; $app->add(new \Slim\Middleware\JwtAuthentication([ "path" => "/api", // The path to verify "secret" => "supersecretkeyyoushouldnotcommittogithub", "algorithm" => ["HS256"], "error" => function ($request, $response, $arguments) { return $response->withJson($arguments["message"], 401); } ])); $app->get('/api/hello', function (Request $request, Response $response, array $args) { $response->getBody()->write("Hello, API!"); return $response; }); $app->run(); ?>
This example shows how to use JWT (JSON Web Token) to implement authentication of APIs. In this way, you can ensure that only authorized users can access your API.
Performance optimization and best practices
Performance optimization and best practices are crucial in practical applications. Let's see how to perform performance optimization and follow best practices in a PHP project.
Performance optimization
Performance optimization can significantly improve the response speed and user experience of PHP applications. Here are some common performance optimization tips:
- Caching : Using cache can reduce the number of database queries and file reads, thereby improving performance. Here is a simple file caching example:
<?php $cacheFile = 'cache/data.json'; $cacheTime = 3600; // Cache Time, unit seconds if (file_exists($cacheFile) && (filemtime($cacheFile) > (time() - $cacheTime))) { $data = json_decode(file_get_contents($cacheFile), true); } else { // Get data from a database or other source $data = array('key' => 'value'); file_put_contents($cacheFile, json_encode($data)); } echo $data['key']; ?>
This example shows how to use file caching to improve performance. In this way, you can reduce frequent access to the database, thereby increasing the response speed of your application.
- Database Optimization : Optimizing database queries and indexes can significantly improve performance. Here is a simple database optimization example:
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; // Create a connection $conn = new mysqli($servername, $username, $password, $dbname); // Check the connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } // Query $sql = "CREATE INDEX idx_title ON posts(title)" using index optimization; $conn->query($sql); // Optimized query $sql = "SELECT id, title FROM posts WHERE title LIKE '%keyword%' LIMIT 10"; $result = $conn->query($sql); if ($result->num_rows > 0) { // Output data while($row = $result->fetch_assoc()) { echo "<h2 id="row-title">" . $row["title"]. "</h2>"; } } else { echo "0 results"; } $conn->close(); ?>
This example shows how to improve database performance by creating indexes and optimizing queries. In this way, you can significantly reduce query time, thereby increasing the response speed of your application.
Best Practices
Following best practices can improve the readability, maintainability, and scalability of your code. Here are some common PHP best practices:
- Code Style : Following a consistent code style can improve the readability of the code. Here is a simple code style example:
<?php // Use camel nomenclature function calculateTotalPrice($price, $quantity) { return $price * $quantity; } // Use meaningful variable name $totalPrice = calculateTotalPrice(10.99, 2); echo "Total price: $" . $totalPrice; ?>
This example shows how to use camel nomenclature and meaningful variable names to improve the readability of your code. In this way, you can make your code easier to understand and maintain.
- Error handling : Good error handling can improve the robustness of the application. Here is a simple error handling example:
<?php function divide($a, $b) { if ($b == 0) { throw new Exception("The divisor cannot be zero"); } return $a / $b; } try { $result = divide(10, 0); echo "Result: " . $result; } catch (Exception $e) { echo "Error: " . $e->getMessage(); } ?>
This example shows how to use exception handling to handle errors. In this way, you can make your application more robust and better handle various exceptions.
Summarize
Through this article, we have in-depth discussion on the practical application of PHP in fields such as e-commerce, content management systems and API development. From shopping cart functionality to payment processing, from dynamic content generation to user management, from RESTful API development to API security, we demonstrate the power and flexibility of PHP.
Performance optimization and best practices are crucial in practical applications. We introduced some common performance optimization tips such as caching and database optimization, and shared some PHP best practices such as code style and error handling.
I hope that through this article, you can better understand the application scenarios of PHP in real life and master some practical techniques and best practices. Whether you are a beginner or an experienced developer, PHP can provide you with powerful tools and unlimited possibilities.
The above is the detailed content of PHP in Action: Real-World Examples and Applications. For more information, please follow other related articles on the PHP Chinese website!
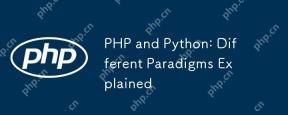
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
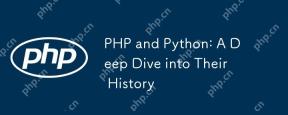
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
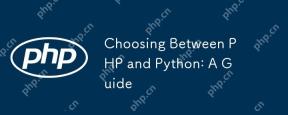
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
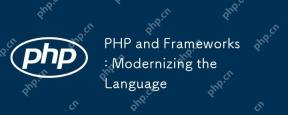
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
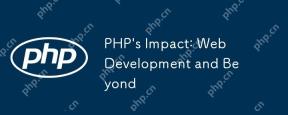
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
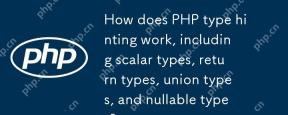
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
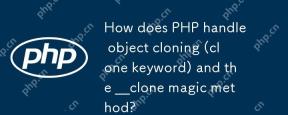
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
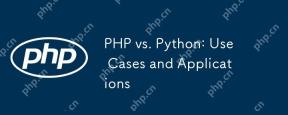
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
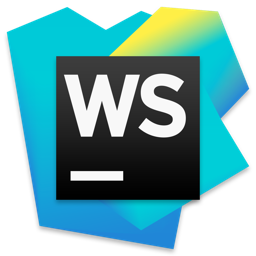
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
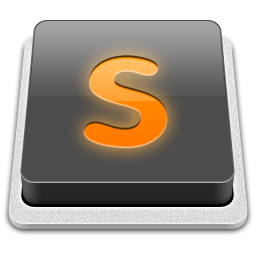
SublimeText3 Mac version
God-level code editing software (SublimeText3)