


How to use CompletableFuture to implement asynchronous concurrent programming in Java?
Use CompletableFuture to implement asynchronous concurrent programming in Java: Create CompletableFuture: Create a CompletableFuture through CompletableFuture.supplyAsync(), which receives a parameterless method as a parameter and returns a value. Handling completion situations: Use the whenComplete(), thenApply(), thenAccept(), exceptionally(), and handle() methods to handle task completion, exception, and cancellation situations. Combining CompletableFutures: Chain two CompletableFutures using the thenCompose() method. Practical case: Shows how to use CompletableFuture to get a list of users from the database in parallel and get each user's details from a remote API.
#How to use CompletableFuture to implement asynchronous concurrent programming in Java?
Introduction
CompletableFuture is a concurrency utility class introduced in Java 8 that allows you to execute tasks asynchronously and handle their completion. It provides a more elegant and simplified way to manage concurrency than traditional threads.
Basic usage
To create a CompletableFuture, you can use the CompletableFuture.supplyAsync() method, which accepts a Supplier as a parameter. Supplier is a method with no parameters and returns a value.
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> "Hello");
Handling completion
One of the main functions of CompletableFuture is the ability to handle task completion. It provides a variety of methods to handle completion, exception and cancellation situations:
-
whenComplete()
: Execute the specified action after the task is completed, regardless of whether the completion is successful or not. -
thenApply()
: Execute the specified function and return a new value after the task is successfully completed. -
thenAccept()
: Execute the specified consumer after the task is successfully completed. -
exceptionally()
: Execute the specified exception handler after the task fails and return a new value. -
handle()
: Execute the specified function after the task is completed, regardless of whether the task is completed successfully, and return a new value.
Combining CompletableFuture
CompletableFuture can be combined to create more complex parallel tasks. For example, you can use the thenCompose()
method to chain together two CompletableFutures:
CompletableFuture<Integer> future1 = CompletableFuture.supplyAsync(() -> 1); CompletableFuture<Integer> future2 = CompletableFuture.supplyAsync(() -> 2); future1.thenCompose(x -> future2.thenApply(y -> x + y));
Practical case
Suppose we have the following tasks Parallel execution is required:
- Get the user list from the database
- Get the details of each user from the remote API
We can use CompletableFuture to Implement it:
CompletableFuture<List<User>> futureUsers = CompletableFuture.supplyAsync(() -> getUsersFromDatabase()); CompletableFuture<Map<Integer, UserDetail>> futureDetails = CompletableFuture.supplyAsync(() -> { List<User> users = futureUsers.get(); return getUsersDetailsFromApi(users); }); CompletableFuture<List<User>> combinedFuture = futureUsers.thenCombine(futureDetails, (users, details) -> { for (User user : users) { user.setDetails(details.get(user.getId())); } return users; });
This example shows how to use CompletableFuture to fetch data from different sources in parallel and combine them.
The above is the detailed content of How to use CompletableFuture to implement asynchronous concurrent programming in Java?. For more information, please follow other related articles on the PHP Chinese website!
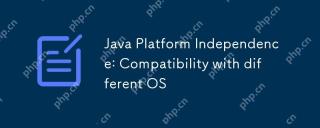
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
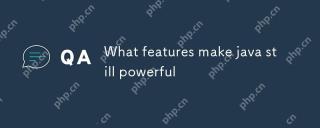
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
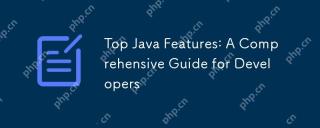
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.
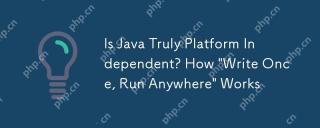
JavaisnotentirelyplatformindependentduetoJVMvariationsandnativecodeintegration,butitlargelyupholdsitsWORApromise.1)JavacompilestobytecoderunbytheJVM,allowingcross-platformexecution.2)However,eachplatformrequiresaspecificJVM,anddifferencesinJVMimpleme
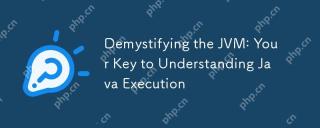
TheJavaVirtualMachine(JVM)isanabstractcomputingmachinecrucialforJavaexecutionasitrunsJavabytecode,enablingthe"writeonce,runanywhere"capability.TheJVM'skeycomponentsinclude:1)ClassLoader,whichloads,links,andinitializesclasses;2)RuntimeDataAr
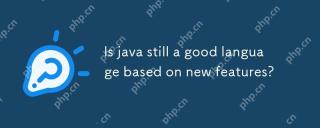
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
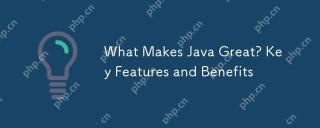
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
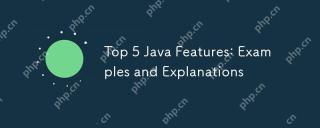
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
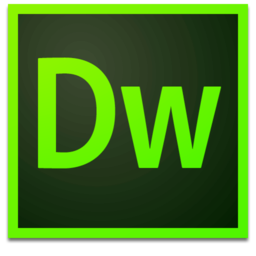
Dreamweaver Mac version
Visual web development tools
