Best practices for Java server-side exception handling include: 1. Use specific exceptions; 2. Handle clear exceptions; 3. Log exceptions; 4. Return user-friendly responses; 5. Avoid suppressing exceptions. The practical example shows an application that handles user registration, effectively managing exceptions through explicit exception handling and HTTP status code return.
Best Practices for Java Server-Side Exception Handling
Introduction
Exceptions Processing is crucial in building robust and user-friendly server-side applications. Java provides rich exception handling functions. This article will introduce best practices and practical cases to guide you to effectively manage server-side exceptions.
Best Practices
1. Use specific exceptions
- Avoid using generic exception types such as Exception or RuntimeException.
- Use specific exceptions to convey information about the cause of the exception, such as NullPointerException or IllegalArgumentException.
2. Handle explicit exceptions
- Handle exceptions that you can expect through try-catch blocks.
- Use the finally block to release resources regardless of whether an exception occurs.
3. Record exceptions
- Use the logging framework to record all unhandled exceptions.
- Contains details such as exception type, message, and stack trace.
4. Return a user-friendly response
- Use HTTP status codes (such as 400 or 500) to indicate errors.
- Return clear and useful error messages to help users understand the problem.
5. Avoid suppressing exceptions
- Do not catch and rethrow exceptions, as this can obscure important information.
- Use Throwable.addSuppressed() to log suppressed exceptions.
Practical Case
Consider a simple application that handles user registration. The following code shows an exception handling scenario:
@PostMapping("/register") public User registerUser(@RequestBody User user) { try { userService.saveUser(user); return user; } catch (DuplicateUsernameException e) { return ResponseEntity.badRequest().body(e.getMessage()); } catch (Exception e) { logger.error("Error while registering user", e); return ResponseEntity.internalServerError().build(); } }
- Explicit exception: DuplicateUsernameException is used to handle the specific exception situation of duplicate user names.
- Explicit handling: DuplicateUsernameException is caught explicitly, returning an HTTP 400 error and error message.
- Unhandled exceptions: Other exceptions are caught and logged in the server-side log, returning an HTTP 500 error.
The above is the detailed content of Best practices for Java server-side exception handling. For more information, please follow other related articles on the PHP Chinese website!
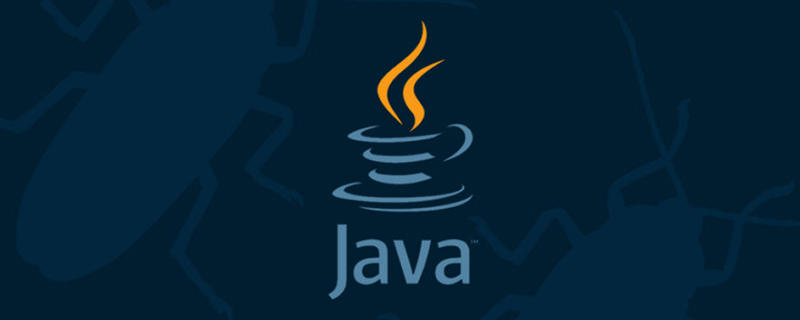
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
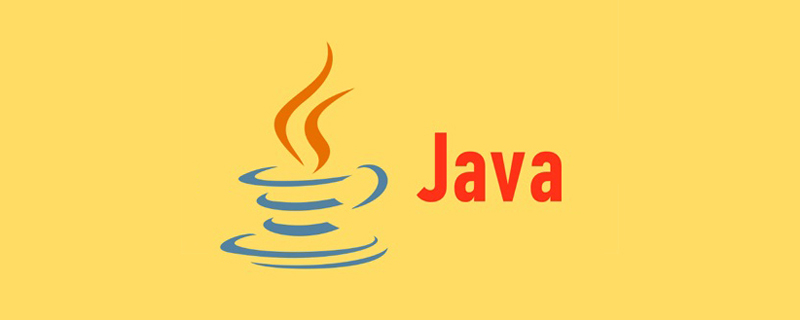
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
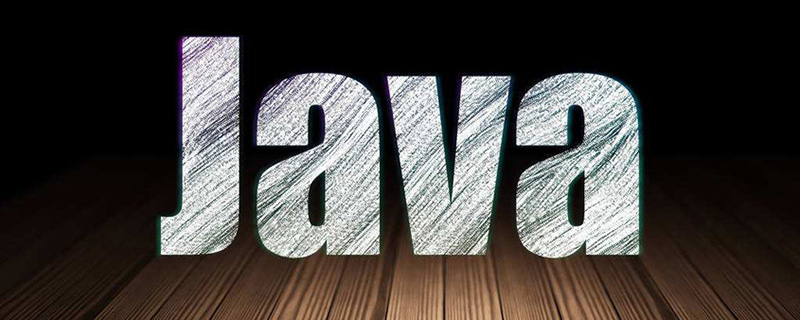
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
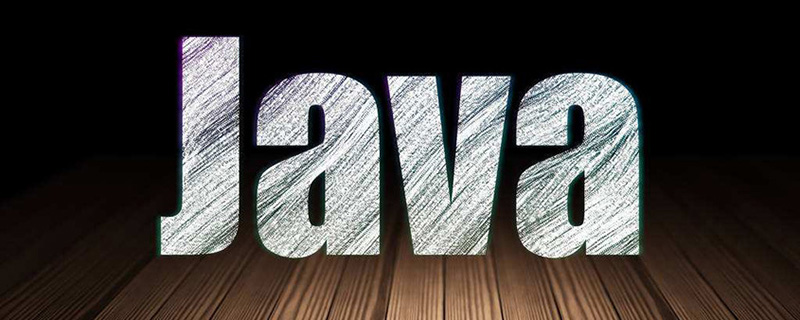
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
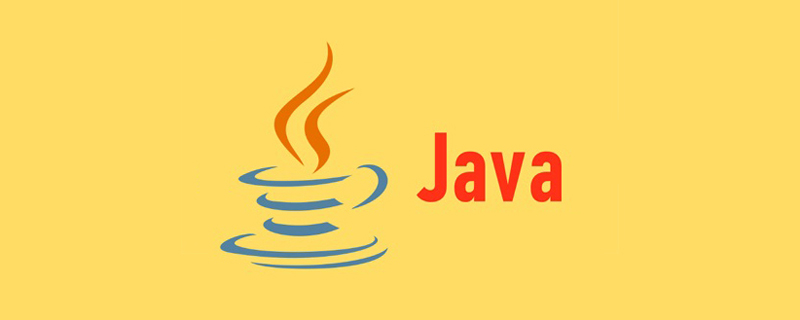
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
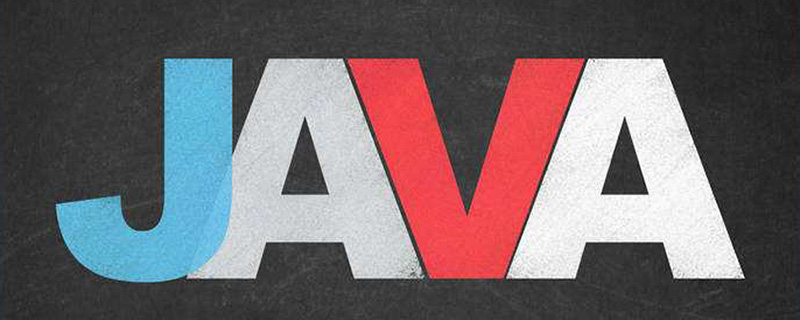
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
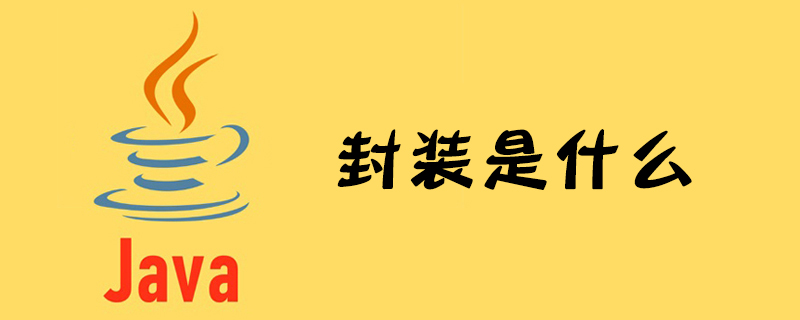
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
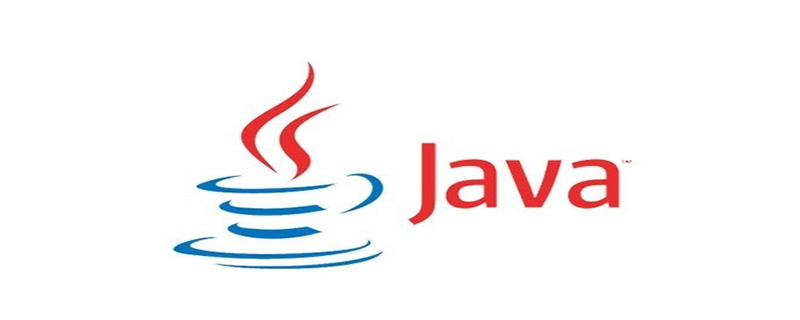
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
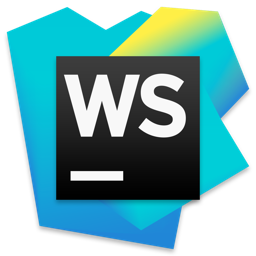
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
