


PHP array value and key interchange: implementation and performance comparison
In PHP, array keys and values can be exchanged through the following methods: array_flip() function: simple syntax, direct key-value exchange, time complexity O(n). Custom function: flexible and can be customized according to needs, but the time complexity is also O(n). Shift operators: Requires PHP knowledge, more efficient in some cases, still O(n) time complexity.
PHP array value and key exchange: implementation and performance comparison
In PHP, it is often necessary to exchange the array key and value so that for further processing. There are multiple ways to do this, each with its pros and cons.
Method 1: array\_flip() function
array_flip()
The function is a built-in function provided by PHP that is directly used to exchange array values and keys. Using it is very simple, just pass the original array as a parameter:
$originalArray = [ 'name' => 'John Doe', 'age' => 30, ]; $flippedArray = array_flip($originalArray); print_r($flippedArray);
Output:
Array ( [John Doe] => name [30] => age )
Method 2: Custom function
You can also define a custom one function to interchange array values and keys:
function flipArray($array) { $flippedArray = []; foreach ($array as $key => $value) { $flippedArray[$value] = $key; } return $flippedArray; }
This custom function works similarly to the array_flip()
function, but it provides greater flexibility. For example, you can add additional logic to handle special cases or modify the output format as needed.
Method 3: Displacement operator
The displacement operator (=>
) can also be used to interchange array keys and values. This approach requires a certain level of PHP knowledge, but it may be more efficient than others in some cases:
$originalArray = [ 'name' => 'John Doe', 'age' => 30, ]; $flippedArray = []; foreach ($originalArray as $key => $value) { $flippedArray[$value] = $key; }
Practical Case
Suppose there is a An array of product information, whose price needs to be obtained based on the product name. Arrays can be quickly transformed using value and key swapping to easily find the price data you need:
$products = [ 'Apple' => 10, 'Orange' => 5, ]; // 使用 array_flip() 互换键和值 $flippedProducts = array_flip($products); // 根据产品名称获取价格 $price = $flippedProducts['Orange'];
In the above example, $price
will now contain the product Orange
price without traversing the entire original array to find it.
Performance comparison
Here is a quick comparison of performance when using different methods to swap arrays:
Method | Time complexity |
---|---|
##array_flip()
| O(n)|
O(n) | |
O(n) |
The above is the detailed content of PHP array value and key interchange: implementation and performance comparison. For more information, please follow other related articles on the PHP Chinese website!
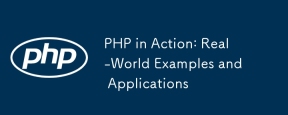
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
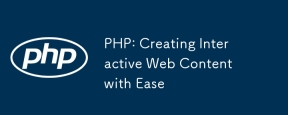
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
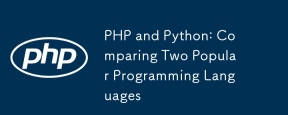
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
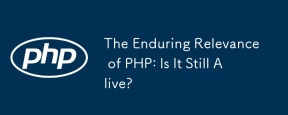
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
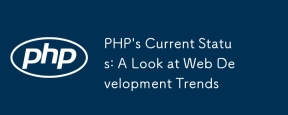
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
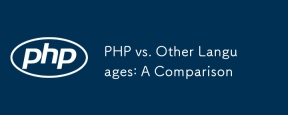
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
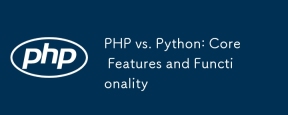
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
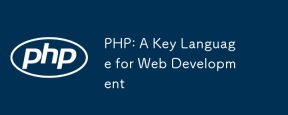
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
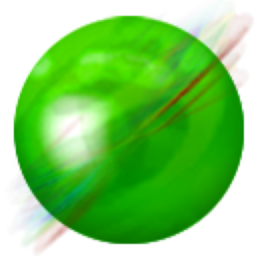
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
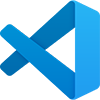
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
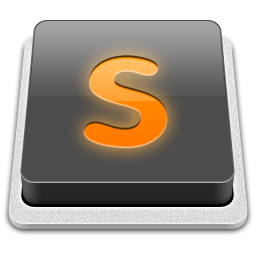
SublimeText3 Mac version
God-level code editing software (SublimeText3)
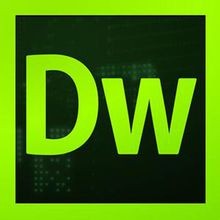
Dreamweaver CS6
Visual web development tools