Best practices for golang function caching and database interaction
In Go, function caching is an effective way to optimize database interaction, storing frequently accessed data in memory to reduce queries. For this purpose, you can use sync.Map, which is a concurrency-safe and fast key-value store. When using function caching, you need to consider data consistency, cache size, and expiration policies to create an efficient and reliable caching system.
Best practices for function caching and database interaction in Go language
Introduction
In large-scale Go applications In the program, optimizing the interaction with the database is crucial. One effective method is to use function caching, which stores frequently accessed data in memory, thereby reducing queries to the database.
Use of function cache
To create a function cache, you can use the sync.Map
type. This is a concurrency-safe and fast key-value store suitable for caching.
import ( "sync" ) var cache = sync.Map{}
Practical Case
Suppose we have a GetUserDetails
function to obtain user information from the database. We can use function caching to improve its performance:
func GetUserDetails(userID int64) (*User, error) { // 检查缓存 if result, ok := cache.Load(userID); ok { return result.(*User), nil } // 从数据库获取数据 user, err := db.GetUser(userID) if err != nil { return nil, err } // 将数据添加到缓存中 cache.Store(userID, user) return user, nil }
In this example, the cache.Load()
method checks if there is a given userID
in the cache value. If there is, return it as type *User
. If not, it will fetch the data from the database, store the results in cache, and return the data.
Notes
You need to pay attention to the following when using function cache:
- Data consistency:The data in the cache may be different from the data in the database The data is out of sync because the cache may not be updated immediately.
- Cache size: The cache should be large enough to accommodate frequently accessed data, but not so large that it exceeds memory.
- Expiration policy: For data that may change, an expiration policy should be implemented to clean out old cache entries.
Conclusion
The performance of Go applications can be significantly improved by applying function caching to interactions with the database. By following best practices and carefully weighing considerations, you can create an efficient and reliable caching system.
The above is the detailed content of Best practices for golang function caching and database interaction. For more information, please follow other related articles on the PHP Chinese website!
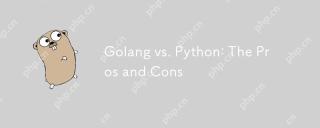
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
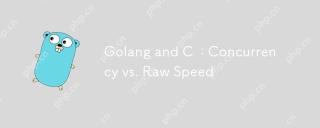
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
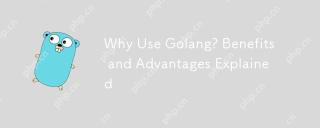
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
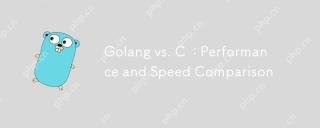
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
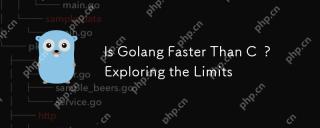
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
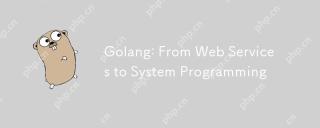
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
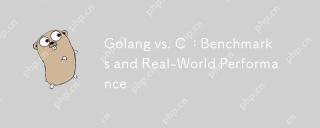
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
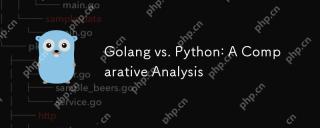
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
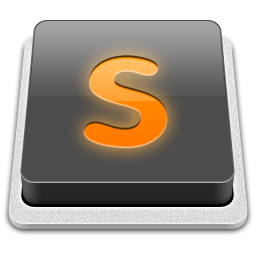
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor