


Inheritance implementation of golang functions in object-oriented programming
Function inheritance is implemented in Go through nested functions: the structure of the parent class is nested in the subclass, and the properties and methods of the parent class are inherited. Define your own methods in subclasses to implement subclass-specific functions. Use the methods of the parent class to access inherited properties, and use the methods of the subclass to access subclass-specific properties. Functional inheritance is not true inheritance, but implemented through function simulation, which provides flexibility but requires careful design.
Inheritance in object-oriented programming in Go functions
In object-oriented programming (OOP), inheritance is an institution. Allows a class (or object) to obtain properties and methods from other classes (called parent or base classes). In the Go language, traditional object-oriented inheritance cannot be used directly, but functions can be used to simulate classes and inheritance.
Implementing function inheritance
In Go, we can use nested structs and functions to implement function inheritance. As shown below:
// 父类 type Parent struct { name string } // 子类 type Child struct { Parent // 嵌套 Parent struct age int } // 父类的方法 func (p *Parent) GetName() string { return p.name } // 子类的方法 func (c *Child) GetAge() int { return c.age }
Practical case
Consider an example where we have Animal
(parent class) and Dog
(Subclass):
// Animal 类 type Animal struct { name string } // Animal 方法 func (a *Animal) GetName() string { return a.name } // Dog 类 (从 Animal 继承) type Dog struct { Animal // 嵌套 Animal struct breed string } // Dog 方法 func (d *Dog) GetBreed() string { return d.breed } func main() { // 创建 Dog 对象 dog := &Dog{ name: "Buddy", breed: "Golden Retriever", } // 使用父类方法 fmt.Println("Dog's name:", dog.GetName()) // 使用子类方法 fmt.Println("Dog's breed:", dog.GetBreed()) }
Output result:
Dog's name: Buddy Dog's breed: Golden Retriever
Notes
- Use the same fields in nested structs name, Go will automatically promote the fields of the parent class to the child class.
- Using functional inheritance can simulate OOP inheritance, but it is not true inheritance.
- Function inheritance provides flexibility, but requires careful design to avoid naming conflicts and structural complexity.
The above is the detailed content of Inheritance implementation of golang functions in object-oriented programming. For more information, please follow other related articles on the PHP Chinese website!
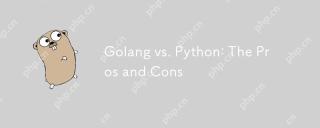
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
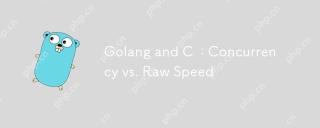
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
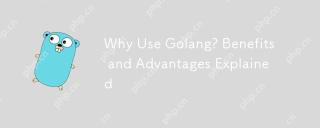
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
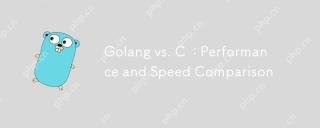
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
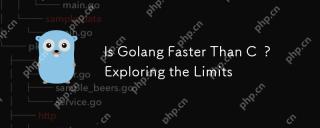
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
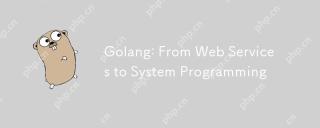
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
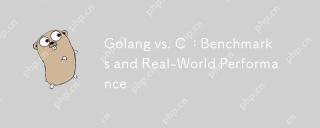
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
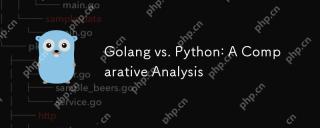
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.