The const modifier indicates a constant and the value cannot be modified; the static modifier indicates the lifetime and scope of the variable. Data members modified by const cannot be modified after initialization. Variables modified by static are initialized when the program starts and destroyed when the program ends. They will exist even if there is no active object and can be accessed across functions. Local variables modified by const must be initialized when declared, while local variables modified by static can be initialized later. Const-modified class member variables must be initialized in the constructor or initialization list, while static-modified class member variables can be initialized outside the class.
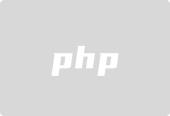
The difference between const and static in c
Simple and clear difference:
- The const modifier represents a constant whose value cannot be modified while the program is running.
- The static modifier indicates the lifetime and scope of a variable.
Detailed explanation:
const Modifier:
- const is used to declare constants, that is A variable whose value cannot be changed.
- const modified data members can only be initialized in the class constructor or initialization list.
- const variables cannot be modified while the program is running, otherwise a compilation error will occur.
- const can be applied to objects, pointers, or references.
static Modifier:
- static is used to declare static variables, that is, variables that exist throughout the program.
- Static variables are initialized when the program starts and destroyed when the program ends.
- Static variables exist even when there is no live object and can be accessed across functions.
- static can be applied to global variables, class member variables and local variables.
Other differences:
- const-modified data members are read-only, while static-modified data members can be read and written.
- Const-modified local variables must be initialized when declared, while static-modified local variables can be initialized later.
- Const-modified class member variables must be initialized in the constructor or initialization list, while static-modified class member variables can be initialized outside the class.
Example:
<code class="cpp">// const 常量
const int MY_CONSTANT = 10;
// static 全局变量
static int global_count;
// static 类成员变量
class MyClass {
public:
static int static_member;
};</code>
In the above example:
- MY_CONSTANT is a constant and cannot be changed once initialized.
- global_count is a static global variable that exists throughout the entire program.
- MyClass::static_member is a static class member variable that can be accessed outside the class.
The above is the detailed content of The difference between const and static in c++. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn