


Optimization strategies for finding specific elements in PHP arrays
How to optimize finding elements in PHP arrays? Use built-in functions: in_array(), array_search(), array_key_exists() to create an index array: use array_flip() to convert an associative array hash table: use SplFixedArray to achieve O(1) time complexity search preprocessing: use a binary search tree Wait for data structures to pre-process large static data sets
Optimization strategy for finding specific elements in PHP arrays
In large data sets Looping through a PHP array to find a specific element can be inefficient, especially if the array is large. To address this challenge, there are several optimization strategies that can significantly increase the speed of lookup operations.
1. Use built-in functions
PHP provides several built-in functions that can be used to find elements in arrays, including:
-
in_array()
: Check whether a specific element exists in the array. -
array_search()
: Find the key of a specific element. -
array_key_exists()
: Check whether a specific key exists in the array.
These functions are highly optimized and very efficient for smaller arrays.
2. Create an index array
The index array uses numeric keys to index elements. When you need to perform frequent lookup operations in an array, indexing an array can significantly speed up lookups. You can convert an associative array to an indexed array using the array_flip()
function. For example:
$assocArray = ['name' => 'John Doe', 'age' => 30]; $indexArray = array_flip($assocArray); // 索引数组:['John Doe' => 'name', 30 => 'age']
In an indexed array, you can access elements directly using numeric keys.
3. Hash table
A hash table is a data structure that allows you to find elements with O(1) time complexity. A hash table is a collection of key-value pairs, with each key mapping to a value. To find a specific element, you hash the element's key to an array index that stores the element's value. Hash tables can be implemented in PHP using the SplFixedArray
class.
4. Preprocessing
For large static data sets, you can preprocess the array and create an index or hash table to avoid traversing every lookup operation . For example, you can sort the elements in an array and create a binary search tree.
Practical Case
Suppose you have an array containing 100,000 employees. Each employee has a unique ID. Now you need to find the employee with employee ID 12345.
Before optimization:
<?php $employees = []; // 假设已填充员工数据 $id = 12345; foreach ($employees as $employee) { if ($employee['id'] === $id) { // 找到员工 } } ?>
After optimization (index array):
<?php $employees = array_flip($employees); // 创建索引数组 $id = 12345; if (isset($employees[$id])) { // 找到员工 } ?>
After optimization (preprocessing ):
<?php $employees = []; // 假设已填充员工数据 // 预处理:创建二分查找树 $bst = new BinarySearchTree(); foreach ($employees as $employee) { $bst->insert($employee['id']); } $id = 12345; $employee = $bst->find($id); // O(log n) 时间复杂度查找
By using these optimization strategies, you can significantly increase the speed of finding specific elements in large PHP arrays. Depending on the size of the array and the frequency of lookup operations, different strategies may provide the best performance.
The above is the detailed content of Optimization strategies for finding specific elements in PHP arrays. For more information, please follow other related articles on the PHP Chinese website!
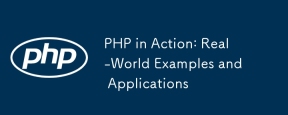
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
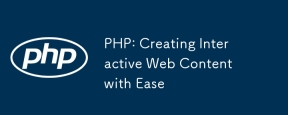
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
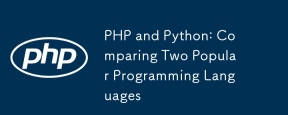
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
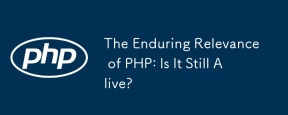
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
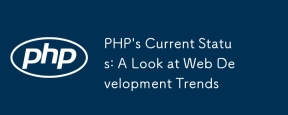
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
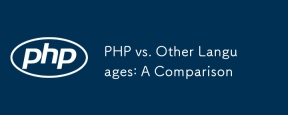
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
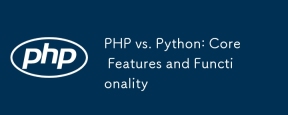
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
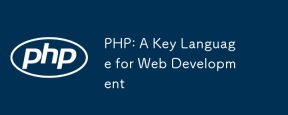
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
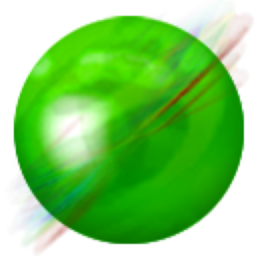
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
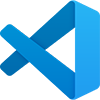
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
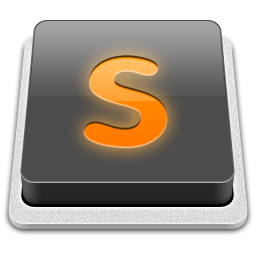
SublimeText3 Mac version
God-level code editing software (SublimeText3)
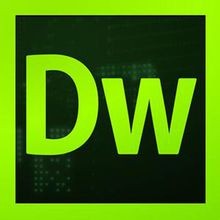
Dreamweaver CS6
Visual web development tools