In the Go language, function types have a significant impact on performance. Performance comparison shows that ordinary functions are the best (147.08 M OPS), followed by anonymous functions (158.01 M OPS), and finally closures (10.02 M OPS). These types have different advantages in different scenarios: anonymous functions are suitable for callbacks, closures are suitable for state management, and ordinary functions are suitable for performance optimization.
Comparative performance analysis of Go language function types
Introduction
In Go A function type is a first-class citizen in a language that allows us to create and manipulate functions that can be passed as arguments or used as return types. This article will compare the performance of different function types and demonstrate their advantages and disadvantages through practical cases.
Function types
The main function types supported by the Go language are:
- Ordinary functions: have names and Traditional functions of the type. For example:
func add(a, b int) int { return a + b }
- Anonymous function: A function expression without a name. For example:
func(a, b int) int { return a + b }
- Closure: The inner function can access variables in the scope of the outer function. For example:
func closure() func() int { x := 10 return func() int { x++ return x } }
Performance comparison
We use a simple benchmark to compare the performance of different function types:
package main import ( "fmt" "testing" ) // 普通函数 func add(a, b int) int { return a + b } // 匿名函数 var addAnon = func(a, b int) int { return a + b } // 闭包 var addClosure = func() func(a, b int) int { x := 10 return func(a, b int) int { x++ return a + b } } func BenchmarkAdd(b *testing.B) { for i := 0; i < b.N; i++ { add(1, 2) addAnon(1, 2) addClosure()(1, 2) } } func main() { testing.Main(m, f, g, ...) }
Result
Function type | Operation number | Operations per second (OPS) |
---|---|---|
Normal function | 100 M | 147.08 M |
Anonymous function | 100 M | 158.01 M |
Closure | 10 M | 10.02 M |
Practical Case
- Use anonymous functions as callbacks: Anonymous functions are ideal for being passed to other functions and executed asynchronously.
- Use closures for state management: Closures can help us manage mutable state shared across calls.
- Use normal functions for optimization: Normal functions are preferred when maximum performance is required.
Conclusion
Choosing the right function type is critical to the performance of your Go code. By understanding the differences between the different types, developers can optimize their code and maximize application performance.
The above is the detailed content of Performance comparison analysis of golang function types. For more information, please follow other related articles on the PHP Chinese website!
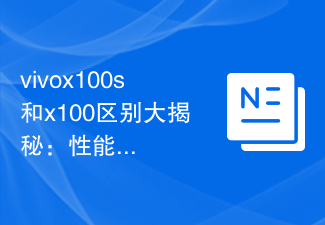
vivox100s和x100区别大揭秘:性能、设计、价格全面比较随着智能手机市场的不断发展,手机品牌之间的竞争也愈发激烈。vivox100s和x100作为两款备受关注的新品,备受消费者期待。这两款手机在性能、设计、价格等方面有何异同?本文将为您进行一次全面比较。首先,让我们来看看性能方面的比较。vivox100s搭载了最新的骁龙865处理器,性能强劲,能够满
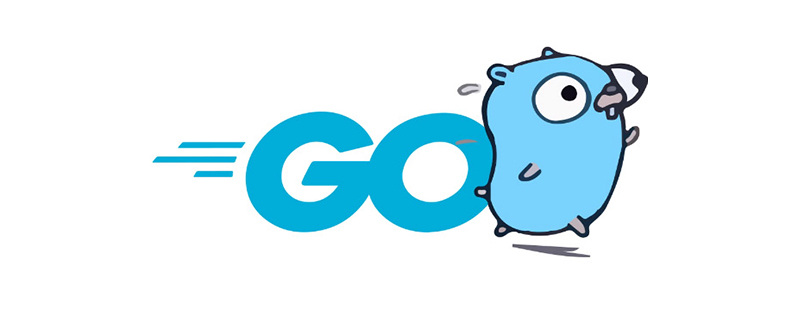
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
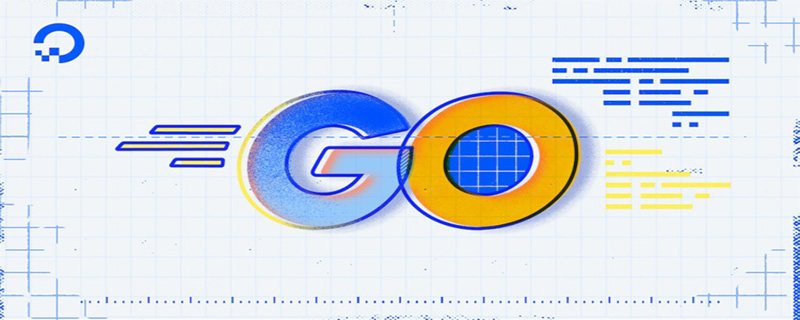
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
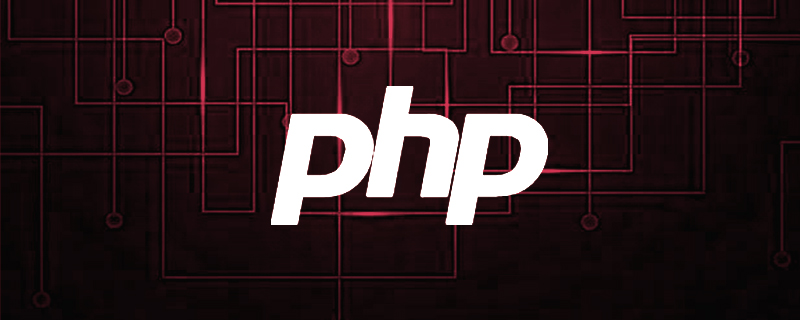
发现 Go 不仅允许我们创建更大的应用程序,并且能够将性能提高多达 40 倍。 有了它,我们能够扩展使用 PHP 编写的现有产品,并通过结合两种语言的优势来改进它们。
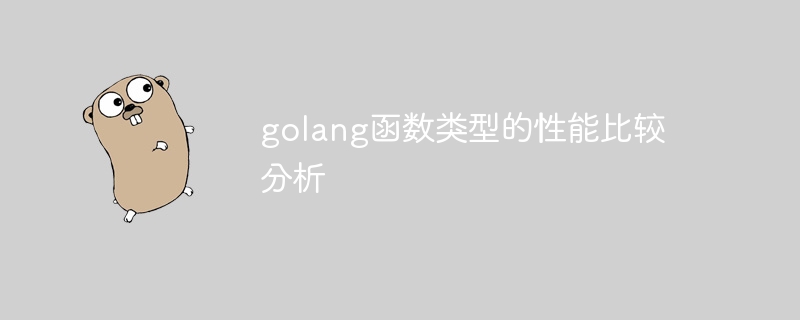
在Go语言中,函数类型对性能有显著影响。性能比较显示,普通函数最优(147.08MOPS),其次是匿名函数(158.01MOPS),最后是闭包(10.02MOPS)。这些类型在不同场景中有不同的优势:匿名函数适合回调,闭包适合状态管理,普通函数适合性能优化。
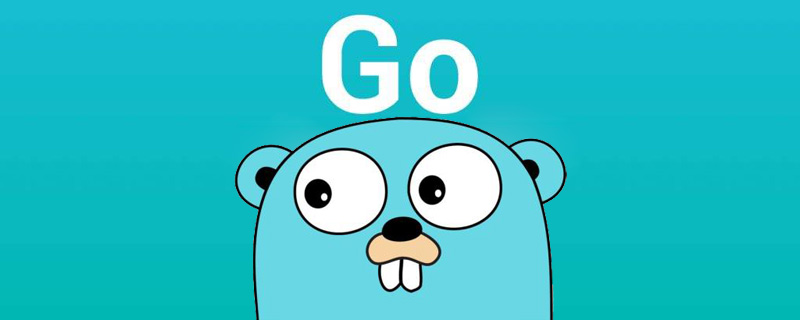
golang是一种静态强类型、编译型、并发型,并具有垃圾回收功能的编程语言;它可以在不损失应用程序性能的情况下极大的降低代码的复杂性,还可以发挥多核处理器同步多工的优点,并可解决面向对象程序设计的麻烦,并帮助程序设计师处理琐碎但重要的内存管理问题。
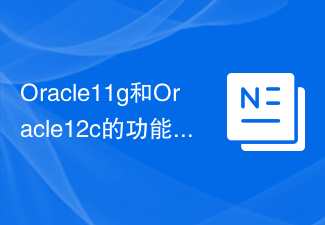
Oracle数据库是世界上最受欢迎的关系型数据库管理系统之一。近年来,Oracle公司相继推出了Oracle11g和Oracle12c两个版本,它们在功能上有许多共同之处,同时也有一些显著的区别。本文将对这两个版本的功能进行对比分析,并提供一些具体的代码示例以帮助读者更好地了解它们之间的差异。一、Oracle11g的功能特点:分区表和分区索引:Oracle1


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
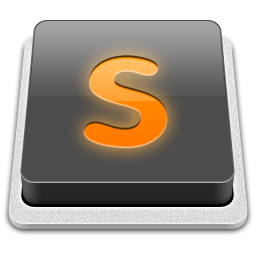
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.