In the Go language, you can use the Slice feature to implement the deletion operation of array elements. Deletion method: 1. Use append() to delete, the syntax is "append(list[:delete index], list[(delete index 1):]...)"; 2. Use copy() to delete, the syntax is "list [:copy(list, list[index:])]"; 3. Use len() to delete, the syntax is "list[:len(list)-N]".
The operating environment of this tutorial: Windows 7 system, GO version 1.18, Dell G3 computer.
In the Go language, you can use the Slice feature to implement the deletion operation of array elements. A slice is a reference to a contiguous fragment of an array, so a slice is a reference type (so more similar to the array type in C/C, or the list type in Python). This fragment can be the entire array, or it can It is a subset of some items identified by the start and end indexes. It should be noted that the items identified by the end index are not included in the slice.
Go language uses slices to delete array elements
Go language does not provide special syntax or interface for deleting slice elements, you need to use the slice itself To delete elements, there are three situations according to the position of the element to be deleted, namely deleting from the beginning, deleting from the middle and deleting from the tail. Among them, deleting elements at the end of the slice is the fastest. [Related recommendations: Go video tutorial, Programming teaching]
Delete from the beginning
You can delete the elements at the beginning Move the data pointer directly:
a = []int{1, 2, 3} a = a[1:] // 删除开头1个元素 a = a[N:] // 删除开头N个元素
You can also move the data pointer without moving it, but to move the subsequent data to the beginning, you can use append to complete it in place (the so-called completion in place refers to the memory interval corresponding to the original slice data) Completed within, will not cause changes in the memory space structure):
a = []int{1, 2, 3} a = append(a[:0], a[1:]...) // 删除开头1个元素 a = append(a[:0], a[N:]...) // 删除开头N个元素
You can also use the copy() function to delete the beginning elements:
a = []int{1, 2, 3} a = a[:copy(a, a[1:])] // 删除开头1个元素 a = a[:copy(a, a[N:])] // 删除开头N个元素
Delete from the middle position
To delete the middle element, you need to move the remaining elements as a whole. You can also use append or copy to complete it in place:
a = []int{1, 2, 3, ...} a = append(a[:i], a[i+1:]...) // 删除中间1个元素 a = append(a[:i], a[i+N:]...) // 删除中间N个元素 a = a[:i+copy(a[i:], a[i+1:])] // 删除中间1个元素 a = a[:i+copy(a[i:], a[i+N:])] // 删除中间N个元素
Delete from the tail
a = []int{1, 2, 3} a = a[:len(a)-1] // 删除尾部1个元素 a = a[:len(a)-N] // 删除尾部N个元素
Deleting the beginning elements and deleting the tail elements can be considered as special cases of deleting the middle elements. Let’s look at an example below.
Array deletion example
[Example] Delete the element at the specified position of the slice.
package main import "fmt" func main() { seq := []string{"a", "b", "c", "d", "e"} // 指定删除位置 index := 2 // 查看删除位置之前的元素和之后的元素 fmt.Println(seq[:index], seq[index+1:]) // 将删除点前后的元素连接起来 seq = append(seq[:index], seq[index+1:]...) fmt.Println(seq) }
Code output result:
The code description is as follows:
Line 1, declares an integer Slice, holds a string containing from a to e.
Line 4, for the convenience of demonstration and explanation, use the index variable to save the position of the element that needs to be deleted.
Line 7, seq[:index] represents the first half of the deleted element, the value is [1 2], seq[index 1:] represents the deleted element The second half of , the value is [4 5].
Line 10, use the append() function to connect the two slices.
Line 12 outputs the connected new slice. At this time, the element with index 2 has been deleted.
The code deletion process can be described using the following figure.
For more programming related knowledge, please visit: Programming Video! !
The above is the detailed content of How to delete elements from golang array. For more information, please follow other related articles on the PHP Chinese website!
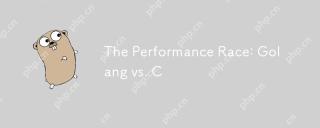
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
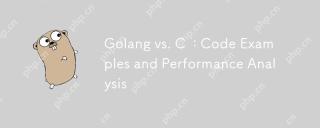
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
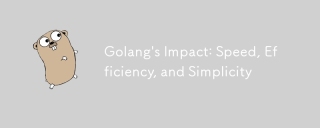
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
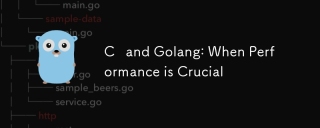
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
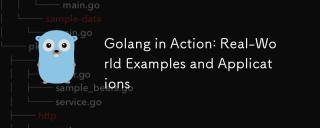
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
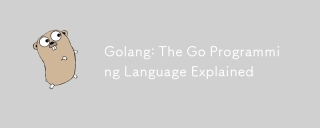
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
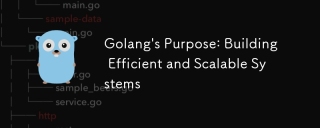
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
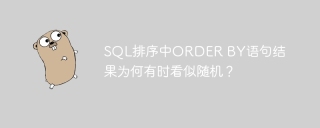
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
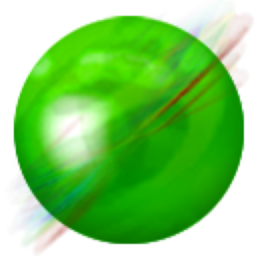
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
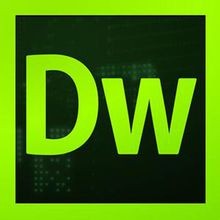
Dreamweaver CS6
Visual web development tools
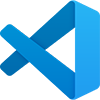
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
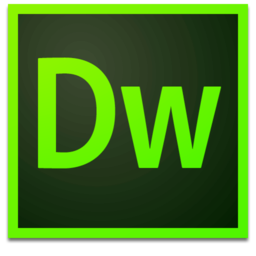
Dreamweaver Mac version
Visual web development tools