What are the best practices for Java functions in serverless architecture?
Best practices for using Java functions in a serverless architecture include: keeping functions stateless, limiting execution time, optimizing memory usage, and implementing error handling. By employing asynchronous programming, throughput can be improved. For example, the code shows a stateless Java function that uses a DynamoDB table to manage state.
Best Practices for Java Functions in Serverless Architecture
Serverless architecture is known for its on-demand scalability, cost-effectiveness, and It is increasingly popular due to its ease of maintenance. Java is a powerful language for building serverless functions, but in order to get the most out of a serverless architecture, it's important to understand best practices.
Best Practices:
1. Keep functions stateless:
Serverless functions should be stateless, which means They should not save any state information. This helps achieve scalability and resiliency. You can do this by storing the state in an external database or service, such as Amazon DynamoDB or Redis.
2. Limit function execution time:
Serverless platforms usually have strict limits on function execution time. It is important to ensure that your function completes execution within limits, otherwise it may result in a cold start or timeout.
3. Optimize memory usage:
Serverless platforms bill functions based on memory allocation. Optimizing memory usage can be achieved by tuning Java Virtual Machine (JVM) parameters, using lightweight frameworks, and carefully managing resources.
4. Use asynchronous programming:
Asynchronous programming allows your functions to respond to parallel requests, thereby increasing throughput. Asynchronous programming can be achieved by using Java's CompletableFuture or the Reactor library.
5. Handling errors:
Serverless platforms do not automatically handle errors. You need to implement error handling logic, log errors and return responses appropriately.
Practical case:
The code in the figure below shows an example Java function that adopts a stateless design and manages state through a DynamoDB table:
import com.amazonaws.services.dynamodbv2.AmazonDynamoDB; import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder; import com.amazonaws.services.dynamodbv2.document.DynamoDB; import com.amazonaws.services.dynamodbv2.document.Item; import com.amazonaws.services.dynamodbv2.document.Table; import com.amazonaws.services.lambda.runtime.Context; import com.amazonaws.services.lambda.runtime.RequestHandler; public class ExampleFunction implements RequestHandler<String, String> { private static final AmazonDynamoDB client = AmazonDynamoDBClientBuilder.standard().build(); private static final DynamoDB dynamoDB = new DynamoDB(client); private static final Table table = dynamoDB.getTable("ExampleTable"); @Override public String handleRequest(String input, Context context) { // Read and update state from DynamoDB Item item = table.getItem("key", input); int count = item.getInt("count") + 1; item.update("count", count); table.putItem(item); // Return the updated count return Integer.toString(count); } }
The above is the detailed content of What are the best practices for Java functions in serverless architecture?. For more information, please follow other related articles on the PHP Chinese website!
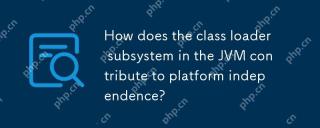
The class loader ensures the consistency and compatibility of Java programs on different platforms through unified class file format, dynamic loading, parent delegation model and platform-independent bytecode, and achieves platform independence.
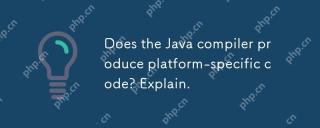
The code generated by the Java compiler is platform-independent, but the code that is ultimately executed is platform-specific. 1. Java source code is compiled into platform-independent bytecode. 2. The JVM converts bytecode into machine code for a specific platform, ensuring cross-platform operation but performance may be different.
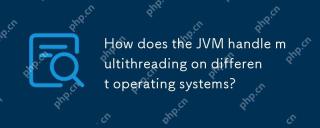
Multithreading is important in modern programming because it can improve program responsiveness and resource utilization and handle complex concurrent tasks. JVM ensures the consistency and efficiency of multithreads on different operating systems through thread mapping, scheduling mechanism and synchronization lock mechanism.
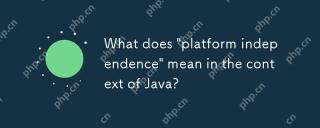
Java's platform independence means that the code written can run on any platform with JVM installed without modification. 1) Java source code is compiled into bytecode, 2) Bytecode is interpreted and executed by the JVM, 3) The JVM provides memory management and garbage collection functions to ensure that the program runs on different operating systems.
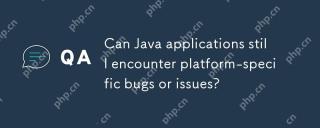
Javaapplicationscanindeedencounterplatform-specificissuesdespitetheJVM'sabstraction.Reasonsinclude:1)Nativecodeandlibraries,2)Operatingsystemdifferences,3)JVMimplementationvariations,and4)Hardwaredependencies.Tomitigatethese,developersshould:1)Conduc
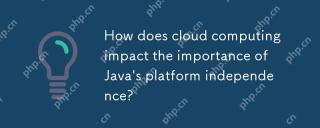
Cloud computing significantly improves Java's platform independence. 1) Java code is compiled into bytecode and executed by the JVM on different operating systems to ensure cross-platform operation. 2) Use Docker and Kubernetes to deploy Java applications to improve portability and scalability.
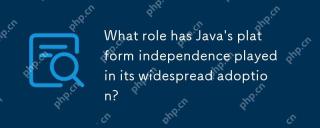
Java'splatformindependenceallowsdeveloperstowritecodeonceandrunitonanydeviceorOSwithaJVM.Thisisachievedthroughcompilingtobytecode,whichtheJVMinterpretsorcompilesatruntime.ThisfeaturehassignificantlyboostedJava'sadoptionduetocross-platformdeployment,s
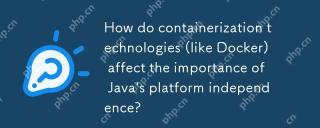
Containerization technologies such as Docker enhance rather than replace Java's platform independence. 1) Ensure consistency across environments, 2) Manage dependencies, including specific JVM versions, 3) Simplify the deployment process to make Java applications more adaptable and manageable.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
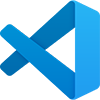
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!