How to implement multi-threaded programming using C++ functions?
How to use C functions to implement multi-threaded programming? Create a std::thread object with a callable object. Call the join() function and wait for the thread to complete. Use thread synchronization techniques such as mutexes, condition variables, and semaphores to ensure safe access to shared resources. In practical cases, you can create threads to process files in parallel to improve program performance.
Using C functions to implement multi-threaded programming
Introduction
Multi-threaded programming involves creating multiple simultaneous task or thread. In C, multithreading can be implemented by using thread functions. This article explains how to use C functions to create and manage threads.
Creating and starting threads
To create threads, you can use the std::thread
class. This class takes as parameter a callable object that defines the task to be performed by the thread. The following is sample code to create a thread:
#include <thread> void thread_function() { // 线程要执行的任务 } int main() { std::thread t(thread_function); t.join(); // 等待线程完成 return 0; }
When the std::thread
object is created, it calls the specified function and creates a new thread to execute the function. join()
The function waits for threads to complete to ensure that all threads have completed before the main thread exits.
Thread synchronization
In order to ensure safe access to shared resources in a multi-threaded environment, thread synchronization technology needs to be used. C provides several synchronization primitives, including:
- Mutex (mutex): is used to protect exclusive access to shared resources.
- Condition variable (condition variable): Used to wait for conditions associated with shared resources.
- Semaphore: Used to limit concurrent access to shared resources.
Practical case
Suppose we want to create a program that processes files in parallel. We can use multithreading to read and process different parts of the file simultaneously. The following is a program example:
#include <thread> #include <vector> #include <iostream> #include <fstream> using namespace std; vector<string> lines; void read_file(const string& filename) { ifstream file(filename); string line; while (getline(file, line)) { lines.push_back(line); } } void process_lines() { for (auto& line : lines) { // 处理行 cout << line << endl; } } int main() { // 创建读取和处理文件的线程 thread t1(read_file, "file1.txt"); thread t2(process_lines); // 等待线程完成 t1.join(); t2.join(); return 0; }
In this example, the read_file()
function is used to read the file, while the process_lines()
function is used to process each line. Multithreading allows the reading and processing of files to occur simultaneously, thus improving program performance.
The above is the detailed content of How to implement multi-threaded programming using C++ functions?. For more information, please follow other related articles on the PHP Chinese website!
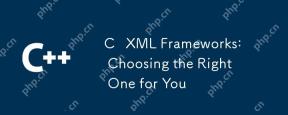
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
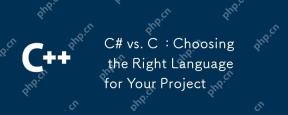
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
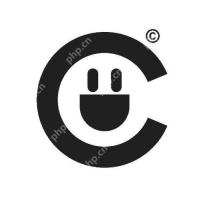
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
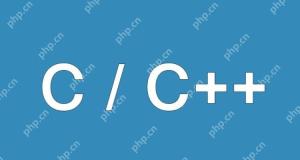
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
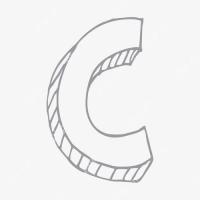
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.
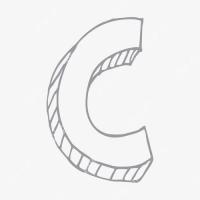
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
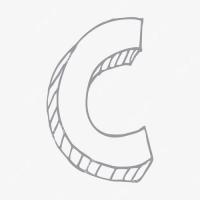
C performs well in real-time operating system (RTOS) programming, providing efficient execution efficiency and precise time management. 1) C Meet the needs of RTOS through direct operation of hardware resources and efficient memory management. 2) Using object-oriented features, C can design a flexible task scheduling system. 3) C supports efficient interrupt processing, but dynamic memory allocation and exception processing must be avoided to ensure real-time. 4) Template programming and inline functions help in performance optimization. 5) In practical applications, C can be used to implement an efficient logging system.
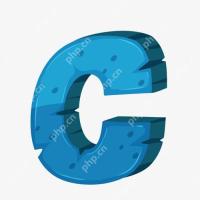
ABI compatibility in C refers to whether binary code generated by different compilers or versions can be compatible without recompilation. 1. Function calling conventions, 2. Name modification, 3. Virtual function table layout, 4. Structure and class layout are the main aspects involved.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
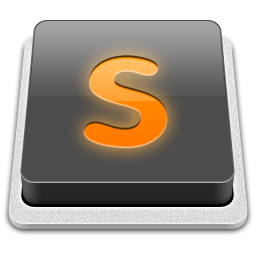
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use
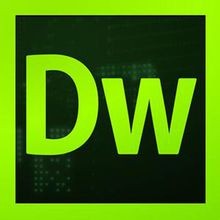
Dreamweaver CS6
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
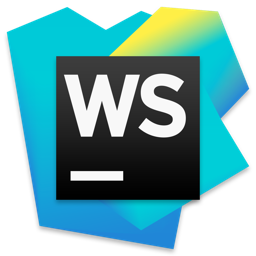
WebStorm Mac version
Useful JavaScript development tools
