C#适合需要开发效率和类型安全的项目,而C++适合需要高性能和硬件控制的项目。1) C#提供垃圾回收和LINQ,适用于企业应用和Windows开发。2) C++以高性能和底层控制著称,广泛用于游戏和系统编程。
引言
在选择编程语言时,C#和C++常常被放在一起比较。它们都是强大且广泛使用的语言,但各有其独特的优势和应用场景。今天我们将深入探讨C#和C++,帮助你决定哪个更适合你的项目。通过这篇文章,你将了解到两者的核心特性、性能差异以及在实际开发中的应用场景。
基础知识回顾
C#是由微软开发的面向对象编程语言,首次发布于2000年,主要用于.NET框架下的开发。它结合了C++的强大功能和Java的简洁性,旨在提高开发者的生产力。C#的语法清晰,支持垃圾回收和丰富的库,使其成为开发Windows应用、游戏和企业软件的理想选择。
C++则是一个更古老的语言,首次发布于1985年,由Bjarne Stroustrup开发。它是C语言的扩展,增加了面向对象编程的特性。C++以其高性能和对底层操作的控制而闻名,广泛应用于系统编程、游戏开发和嵌入式系统。
核心概念或功能解析
C#的特性与优势
C#的设计初衷是让开发者更容易编写和维护代码。它的垃圾回收机制解放了开发者,使他们不必手动管理内存,这大大减少了内存泄漏的风险。C#还支持LINQ(Language Integrated Query),这使得数据查询和操作变得异常简单和直观。
// LINQ示例 List<int> numbers = new List<int> { 1, 2, 3, 4, 5 }; var evenNumbers = numbers.Where(n => n % 2 == 0).ToList(); Console.WriteLine(string.Join(", ", evenNumbers)); // 输出: 2, 4
C#的异步编程模型(async/await)也让处理并发任务变得更加简单和高效,这在现代应用开发中尤为重要。
C++的特性与优势
C++的最大优势在于其性能和对硬件的直接控制。它的编译型特性使得代码运行速度极快,这对于需要高性能的应用如游戏引擎、操作系统和嵌入式系统非常关键。C++的模板编程和RAII(Resource Acquisition Is Initialization)技术使得资源管理更加高效和安全。
// RAII示例 class File { public: File(const char* name) : file(fopen(name, "r")) { if (!file) throw std::runtime_error("无法打开文件"); } ~File() { fclose(file); } // 使用文件的其他方法... private: FILE* file; };
C++的多态性和继承机制也使得它在复杂系统的设计中表现出色。
使用示例
C#在企业应用中的应用
C#在企业应用开发中非常流行,特别是在使用ASP.NET开发Web应用时。它的类型安全和丰富的库使得开发大型应用变得更加可控和高效。
// ASP.NET Core示例 using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.DependencyInjection; public class Startup { public void ConfigureServices(IServiceCollection services) { services.AddControllersWithViews(); } public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } else { app.UseExceptionHandler("/Home/Error"); app.UseHsts(); } app.UseHttpsRedirection(); app.UseStaticFiles(); app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapControllerRoute( name: "default", pattern: "{controller=Home}/{action=Index}/{id?}"); }); } }
C++在游戏开发中的应用
C++在游戏开发中占据主导地位,因为它能提供高性能和对硬件的精细控制。许多著名的游戏引擎如Unreal Engine和CryEngine都是用C++编写的。
// 游戏循环示例 #include <iostream> class Game { public: void run() { while (isRunning) { processInput(); update(); render(); } } private: bool isRunning = true; void processInput() { // 处理用户输入 } void update() { // 更新游戏状态 } void render() { // 渲染游戏画面 } }; int main() { Game game; game.run(); return 0; }
常见错误与调试技巧
在C#中,常见的错误包括空引用异常和异步编程中的死锁。使用调试工具如Visual Studio的调试器可以帮助你快速定位和解决这些问题。
在C++中,常见的错误包括内存泄漏和指针错误。使用智能指针和内存分析工具如Valgrind可以帮助你避免这些问题。
性能优化与最佳实践
C#的性能优化
C#的性能优化主要集中在减少垃圾回收的频率和优化LINQ查询。使用struct
而不是class
来定义小型数据结构可以减少内存分配,使用Span<t></t>
和ReadOnlySpan<t></t>
可以提高字符串和数组操作的性能。
// 使用Span<T>优化字符串操作 public static int CountVowels(ReadOnlySpan<char> text) { int count = 0; foreach (char c in text) { if ("aeiouAEIOU".IndexOf(c) != -1) { count++; } } return count; }
C++的性能优化
C++的性能优化主要集中在减少内存分配和提高缓存命中率。使用std::vector
而不是动态数组可以减少内存碎片,使用const
和constexpr
可以帮助编译器进行更多的优化。
// 使用std::vector优化内存管理 #include <vector> void processData(const std::vector<int>& data) { for (const auto& item : data) { // 处理数据 } }
最佳实践
无论是C#还是C++,编写可读性和可维护性高的代码都是至关重要的。使用有意义的变量名和函数名,编写详细的注释和文档,遵循代码风格指南,这些都是提高代码质量的关键。
在选择C#还是C++时,需要考虑项目的具体需求。如果你的项目需要高性能和对硬件的直接控制,C++可能是更好的选择。如果你的项目更注重开发效率和类型安全,C#则更为合适。
通过这篇文章的探讨,希望你能更好地理解C#和C++的优劣势,从而做出更明智的选择。无论你选择哪种语言,祝你在编程之路上一切顺利!
The above is the detailed content of C# vs. C : Choosing the Right Language for Your Project. For more information, please follow other related articles on the PHP Chinese website!
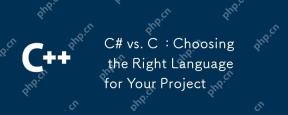
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
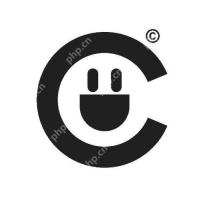
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
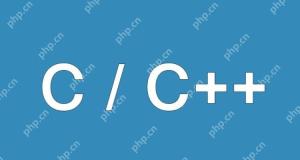
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
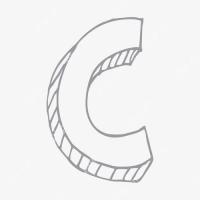
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.
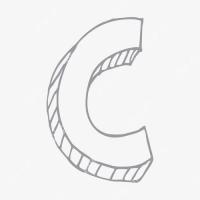
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
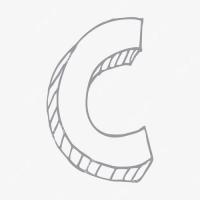
C performs well in real-time operating system (RTOS) programming, providing efficient execution efficiency and precise time management. 1) C Meet the needs of RTOS through direct operation of hardware resources and efficient memory management. 2) Using object-oriented features, C can design a flexible task scheduling system. 3) C supports efficient interrupt processing, but dynamic memory allocation and exception processing must be avoided to ensure real-time. 4) Template programming and inline functions help in performance optimization. 5) In practical applications, C can be used to implement an efficient logging system.
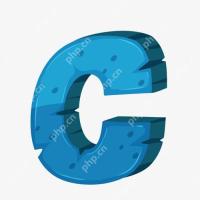
ABI compatibility in C refers to whether binary code generated by different compilers or versions can be compatible without recompilation. 1. Function calling conventions, 2. Name modification, 3. Virtual function table layout, 4. Structure and class layout are the main aspects involved.
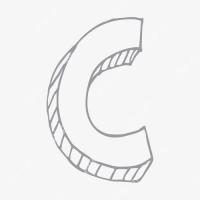
DMA in C refers to DirectMemoryAccess, a direct memory access technology, allowing hardware devices to directly transmit data to memory without CPU intervention. 1) DMA operation is highly dependent on hardware devices and drivers, and the implementation method varies from system to system. 2) Direct access to memory may bring security risks, and the correctness and security of the code must be ensured. 3) DMA can improve performance, but improper use may lead to degradation of system performance. Through practice and learning, we can master the skills of using DMA and maximize its effectiveness in scenarios such as high-speed data transmission and real-time signal processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
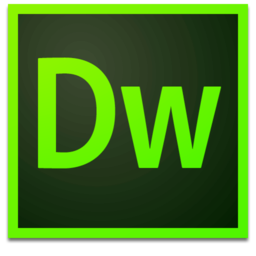
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
