


C functions handle user input and events with the following benefits: Modular and reusable code: Break down tasks, simplify testing and improve code quality. Input validation and exception handling: Ensure user input is valid and provide consistent error handling. Event handling: Use event handlers to create interactive applications in response to user interaction or system state changes.
Advantages of C functions in handling user input and events
When developing C applications, functions are used in handling user input and events play a key role. Functions provide the advantages of modular and reusable code, allowing developers to build robust applications more efficiently.
Modular and reusable code
Functions break complex tasks into smaller, manageable parts. This allows developers to easily isolate and test each operation, reducing errors and improving code quality. Additionally, functions can be reused, saving programming time and promoting code consistency.
Input validation and exception handling
C function can implement the input validation mechanism to ensure that user input is valid. For example, invalid input can be prevented by passing user input as arguments to functions and bounds checking the values. Functions also handle errors and exceptions efficiently, providing consistent error messages and a more user-friendly interface.
Event handling
C functions are particularly powerful in handling events, which are blocks of code triggered by user actions or external stimuli. Functions that respond to events are called event handlers, and they allow developers to create interactive applications that react to user interaction or changes in system state.
Practical case
The following C code demonstrates how to use functions to handle user input and respond to events:
// 处理用户输入的函数 int getUserInput() { int input; cout << "Enter a number: "; cin >> input; return input; } // 事件处理程序函数 void onButtonClicked() { cout << "Button clicked!" << endl; } int main() { // 获取用户输入 int num = getUserInput(); // 根据用户输入执行操作 if (num % 2 == 0) { cout << "The number is even." << endl; } else { cout << "The number is odd." << endl; } // 处理按钮点击事件 onButtonClicked(); return 0; }
In this example, The getUserInput()
function is responsible for obtaining user input, and the onButtonClicked()
function serves as the handler for the button click event. The modular and reusable nature of functions makes code easier to maintain and ensures a consistent user experience.
The above is the detailed content of What advantages do C++ functions have when handling user input and events?. For more information, please follow other related articles on the PHP Chinese website!
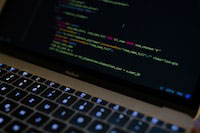
pythonGUI编程简述GUI(GraphicalUserInterface,图形用户界面)是一种允许用户通过图形方式与计算机交互的方式。GUI编程是指使用编程语言来创建图形用户界面。Python是一种流行的编程语言,它提供了丰富的GUI库,使得PythonGUI编程变得非常简单。PythonGUI库介绍Python中有许多GUI库,其中最常用的有:Tkinter:Tkinter是Python标准库中自带的GUI库,它简单易用,但功能有限。PyQt:PyQt是一个跨平台的GUI库,它功能强大,
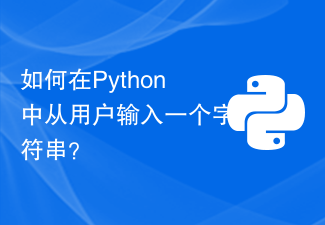
在Python中,有几种方法可以从用户输入一个字符串。最常见的方法是使用内置函数input()。该函数允许用户输入一个字符串,然后将其存储为一个变量以供程序使用。示例下面是一个在Python中从用户输入字符串的示例−#Defineavariabletostoretheinputname=input("Pleaseenteryourname:")#Printtheinputprint("Hello,"+name+"!Goodtoseeyou.&qu
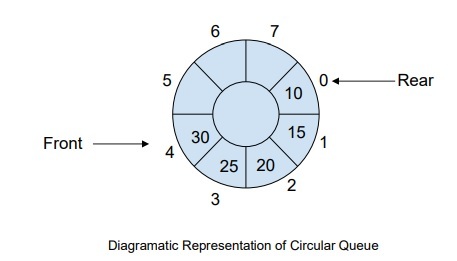
介绍CircularQueue是对线性队列的改进,它被引入来解决线性队列中的内存浪费问题。循环队列使用FIFO原则来插入和删除其中的元素。在本教程中,我们将讨论循环队列的操作以及如何管理它。什么是循环队列?循环队列是数据结构中的另一种队列,其前端和后端相互连接。它也被称为循环缓冲区。它的操作与线性队列类似,那么为什么我们需要在数据结构中引入一个新的队列呢?使用线性队列时,当队列达到其最大限制时,尾指针之前可能会存在一些内存空间。这会导致内存损失,而良好的算法应该能够充分利用资源。为了解决内存浪费
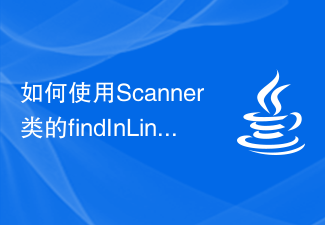
如何使用Scanner类的findInLine()方法在用户输入中查找指定的字符串Scanner类是Java中常用的输入处理类,它提供了多种方法来从输入流中读取数据。其中,findInLine()方法可以用来在用户输入中查找指定的字符串。本文将介绍如何使用Scanner类的findInLine()方法,并附上相应的代码示例。在开始使用Scanner类的fin
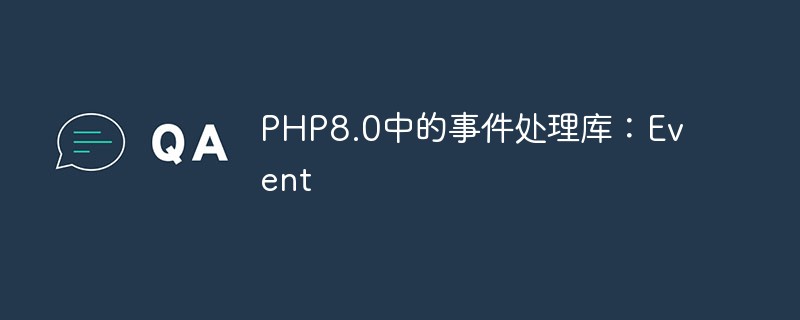
PHP8.0中的事件处理库:Event随着互联网的不断发展,PHP作为一门流行的后台编程语言,被广泛应用于各种Web应用程序的开发中。在这个过程中,事件驱动机制成为了非常重要的一环。PHP8.0中的事件处理库Event将为我们提供一个更加高效和灵活的事件处理方式。什么是事件处理在Web应用程序的开发中,事件处理是一个非常重要的概念。事件可以是任何一种用户行
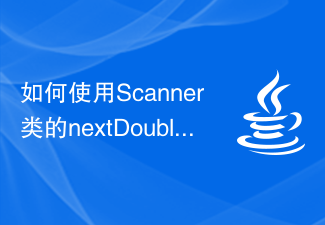
如何使用Scanner类的nextDouble()方法从用户输入中读取浮点数在Java中,Scanner类是一个非常常用的类,用于从用户输入中读取数据。Scanner类提供了许多不同的方法,可以读取不同类型的数据。其中,nextDouble()方法可以用于读取浮点数。下面是一个简单的示例代码,展示了如何使用Scanner类的nextDouble()方法从用户
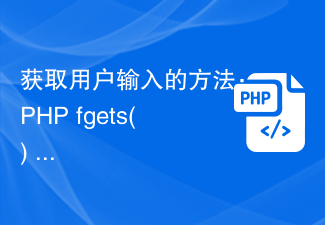
在网页制作中,获取用户的输入是一个重要的环节。PHP是一种功能强大的脚本语言,它可以与HTML配合使用,实现不同的任务,包括获取用户的输入。在PHP中,有许多函数可以用来获取用户的输入,其中一个非常常见的函数是fgets()。本文将详细介绍PHPfgets()函数的用法和使用注意事项。一、fgets()函数的基础用法PHPfgets()
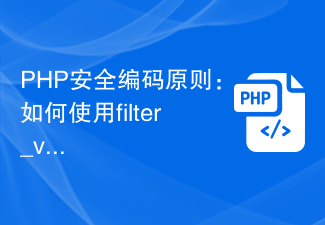
PHP安全编码原则:如何使用filter_var函数过滤并检验用户输入概述:随着互联网的快速发展,以及Web应用的广泛应用,安全问题变得越来越重要。而对用户输入进行有效且安全的过滤和验证是确保Web应用程序安全的关键之一。本文将介绍PHP中的filter_var函数,以及如何使用它来过滤和验证用户输入,从而提供更加安全的编码实践。filter_var函数:f


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
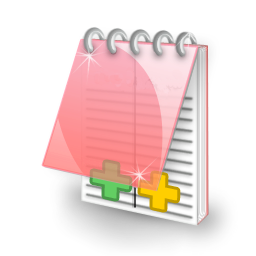
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
