Best practices for error handling in Go include: using the error type, always returning errors, checking for errors, using multi-value returns, using sentinel errors, and using error wrappers. Practical example: In the HTTP request handler, if ReadDataFromDatabase returns an error, return a 500 error response.
Best Practices for Error Handling in Go Functions
In Go, handling errors is crucial to building robust and reliable applications. It's important. Here are some best practices to help you handle errors efficiently:
Using the error
type
Go provides a built-in error
Type used to represent errors. It is an interface that any type can implement, allowing you to create custom error types.
package errors type MyError struct { msg string } func (e MyError) Error() string { return e.msg }
Return an error
Always return an error, even if the name of the function does not indicate that the function may fail. This will enable the caller to determine whether the function executed successfully.
func ReadFile(filename string) (string, error) { data, err := ioutil.ReadFile(filename) return data, err }
Checking for errors
Use the if err != nil
statement to check for errors. If an error occurs, take appropriate action, such as logging the error or returning the error to the caller.
func main() { data, err := ReadFile("data.txt") if err != nil { log.Fatal(err) } }
Use multi-value returns
If you need to return multiple values for an operation that may fail, you can use multi-value returns. The first value returned is the actual result, the second value returned is the error.
func Divide(a, b int) (float64, error) { if b == 0 { return 0, errors.New("division by zero") } return float64(a) / float64(b), nil }
Using sentinel errors
Sentinel errors are predefined error values used to indicate specific types of failures. This helps you easily detect and handle these types of errors.
var ErrNotFound = errors.New("not found")
Using Error Wrapping
When you need to wrap an error to provide additional context, use fmt.Errorf
or errors. Wrap
function. This enables you to create more descriptive and understandable error messages.
func ReadFileFromRemote(filename string) (string, error) { data, err := ReadFileFromCache(filename) if err != nil { return "", fmt.Errorf("failed to read from cache: %w", err) } return data, nil }
Practical Case: Handling Errors in HTTP Requests
Here is an example showing how to use these best practices in an HTTP request handler:
package main import ( "fmt" "net/http" ) // 处理传入的 HTTP 请求 func handler(w http.ResponseWriter, r *http.Request) { data, err := ReadDataFromDatabase(r) if err != nil { // 返回一个带有 500 错误代码的错误响应 http.Error(w, err.Error(), http.StatusInternalServerError) return } // 处理成功请求并返回响应 }
By following these best practices, you can write robust and reliable Go code that handles and reports errors effectively, thereby improving application stability and user experience.
The above is the detailed content of Best practices for error handling in golang functions. For more information, please follow other related articles on the PHP Chinese website!
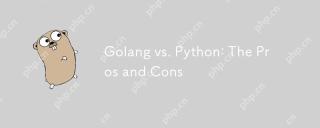
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
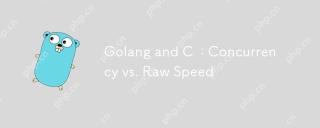
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
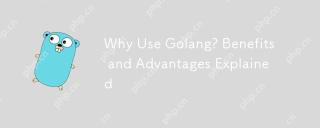
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
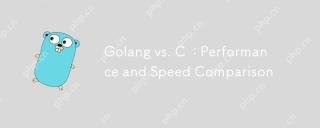
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
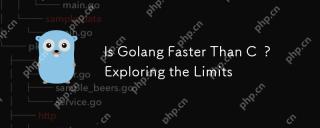
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
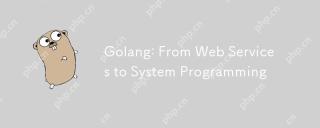
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
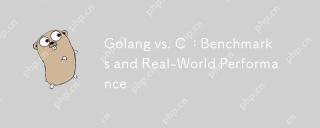
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
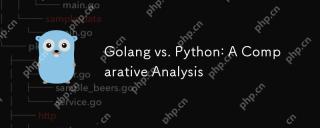
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
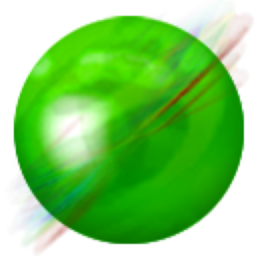
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
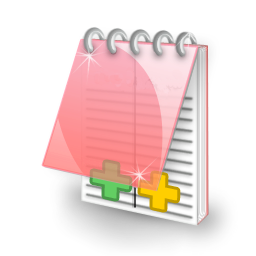
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor