How to use function overloading and rewriting effectively in C++
Function overloading and rewriting guide: Function overloading: Create multiple functions with the same name but different parameters to reduce code redundancy. Function rewriting: Declare a function with the same name in a subclass, modify the behavior of the inherited function, and achieve polymorphism. Practical case: Function overloading: processing different data types. Function rewriting: implementing inheritance polymorphism. Best Practice: Carefully consider overloaded function signatures. Avoid excessive overloading. Use function overrides as needed. Use the override keyword when overriding virtual functions.
A guide to efficient use of function overloading and rewriting in C
Preface
Functions Overloading and rewriting are powerful tools for increasing code flexibility and readability. Understanding them and applying them correctly is crucial to writing high-quality and maintainable C code.
Function Overloading
Overloading allows us to create multiple functions with the same name but different parameters (type or number). It makes it easy for us to use the same functionality in different situations, thereby reducing code redundancy.
Code example:
double sum(int a, int b) { return a + b; } double sum(double a, double b) { return a + b; } double sum(int a, double b) { return a + b; }
In this example, the sum function is overloaded three times, each time accepting a different combination of parameters.
Function rewriting
Rewriting is a function in the subclass that declares a function with the same name as the parent class. This allows us to modify the behavior of inherited functions to suit the specific needs of the subclass.
Code example:
class Shape { public: virtual double area() { return 0; } }; class Circle : public Shape { public: double area() override { return 3.14 * radius * radius; } };
In this example, the Circle class overrides the area function in the Shape class to calculate the area of a circle.
Practical case
Use function overloading to process different data types:
When processing data of different data types, function overloading You can avoid creating multiple functions that have the same functionality but accept arguments of different types.
Implementing inherited polymorphism using function overriding:
Function overriding allows subclasses to customize the behavior of inherited functions to their specific requirements, thereby implementing polymorphism.
Best Practices
- Carefully consider overloaded function signatures to avoid name conflicts.
- Avoid excessive overloading as this will reduce code readability.
- Use function overriding only when you need to override parent class behavior.
- Use the override keyword when overriding virtual functions.
The above is the detailed content of How to use function overloading and rewriting effectively in C++. For more information, please follow other related articles on the PHP Chinese website!
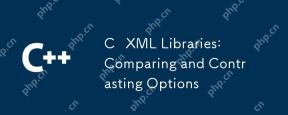
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
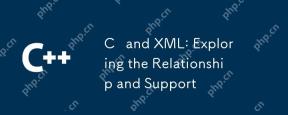
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
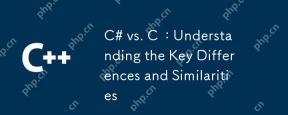
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
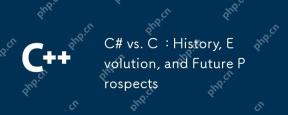
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
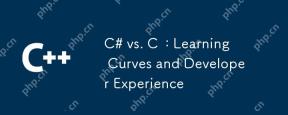
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
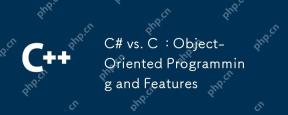
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
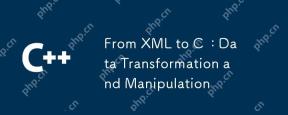
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
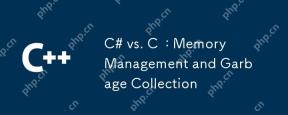
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
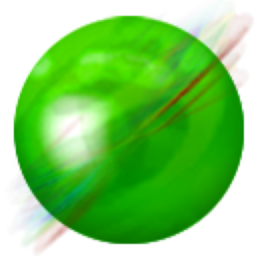
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
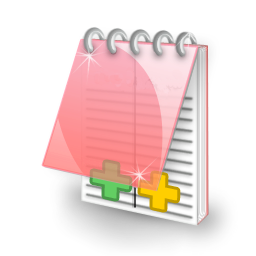
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor