Benefits of using enumeration types as function return values: Improve readability: Use meaningful name constants to enhance code understanding. Type safety: Ensure return values fit within the expected range and avoid unexpected behavior. Save memory: Enumerated types generally take up less storage space. Easy to extend: New values can be easily added to the enumeration.
The benefits of C functions returning enumeration types
When the function needs to return a value that is not a basic data type but does not want to create its own It is useful to use enumeration types when defining the values of a class. Enumerations allow us to create a set of values with named constants that can be used to represent a specific state or situation.
Advantages of using enumeration types:
- Improved readability: By using meaningful names, the code can be improved Readability and understandability.
- Type safety: Enumeration types ensure that the returned values fall within the expected range, avoiding unexpected behavior and errors.
- Memory Savings: Enumeration types typically use a smaller number of bits to store values, thus saving memory.
- Easy to extend: New values can be easily added to the enumeration when needed.
Example:
Consider a function that computes the result of a mathematical operation. We can use enumeration types to represent the results of operations.
enum class MathResult { Success, DivByZero, Overflow, Underflow }; MathResult CalculateResult(double num1, double num2, char op) { switch (op) { case '+': return (num1 + num2 > DBL_MAX) ? MathResult::Overflow : MathResult::Success; case '-': return (num1 - num2 < DBL_MIN) ? MathResult::Underflow : MathResult::Success; case '*': return (num1 * num2 > DBL_MAX) ? MathResult::Overflow : MathResult::Success; case '/': if (num2 == 0) { return MathResult::DivByZero; } return (num1 / num2 > DBL_MAX) ? MathResult::Overflow : MathResult::Success; } } int main() { double num1 = 10.0; double num2 = 2.0; char op = '+'; MathResult result = CalculateResult(num1, num2, op); switch (result) { case MathResult::Success: std::cout << "Operation successful" << std::endl; break; case MathResult::DivByZero: std::cout << "Division by zero error" << std::endl; break; case MathResult::Overflow: std::cout << "Overflow error" << std::endl; break; case MathResult::Underflow: std::cout << "Underflow error" << std::endl; break; } return 0; }
This will output:
Operation successful
The above is the detailed content of What are the benefits when a C++ function returns an enumeration type?. For more information, please follow other related articles on the PHP Chinese website!
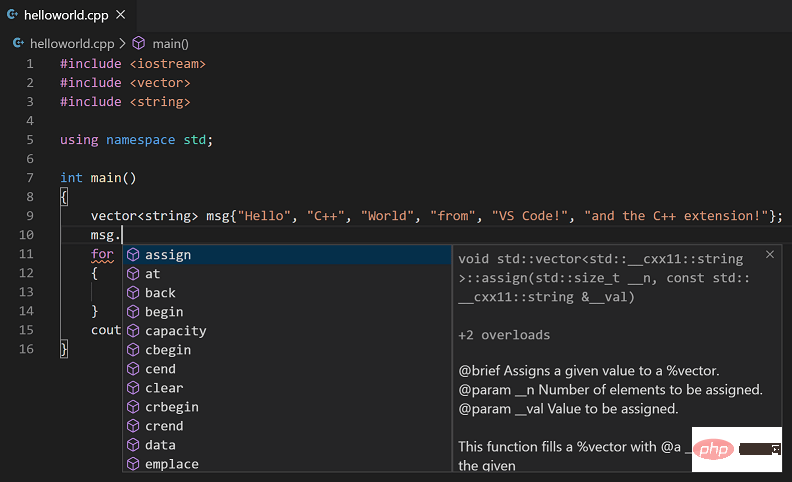
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
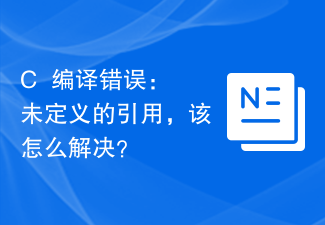
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
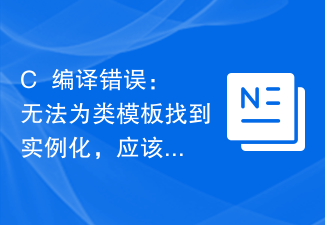
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
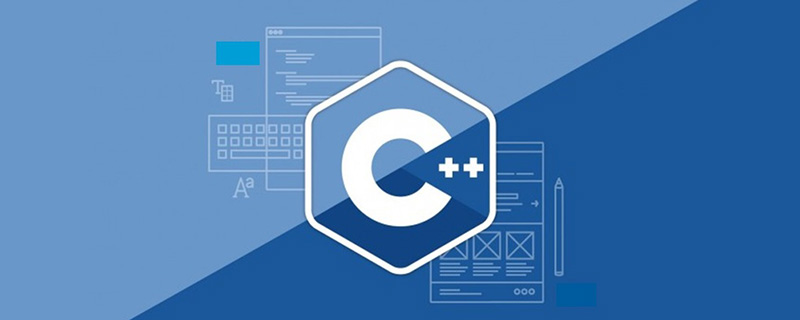
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
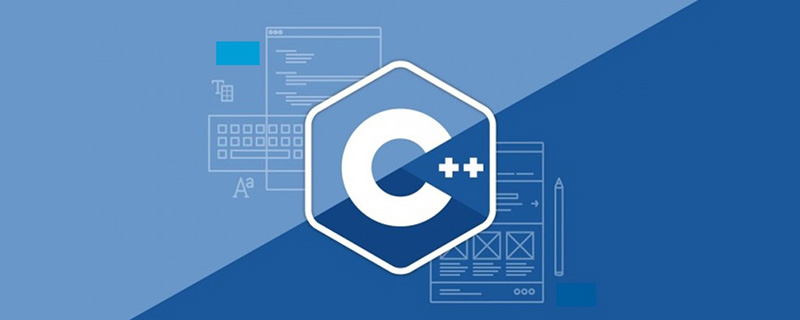
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
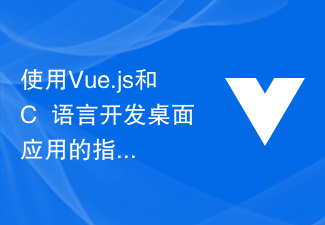
使用Vue.js和C++语言开发桌面应用的指南随着互联网的发展,前端技术也在不断更新和进步。而Vue.js作为一种轻量级、高效、易用的前端框架,在开发Web应用方面具有很大的优势。然而,在一些特定的场景中,我们可能需要开发一些更加复杂的桌面应用程序,这时候就需要结合C++语言来实现一些底层功能。本文将会介绍如何使用Vue.js和C++语言开发桌面应用,并提供
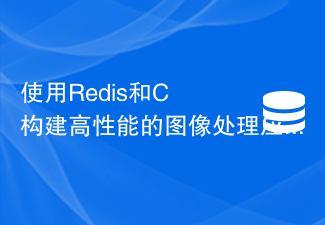
使用Redis和C++构建高性能的图像处理应用图像处理是现代计算机应用中的重要环节之一。由于图像处理的复杂性和计算量大,如何在保证高性能的同时提供稳定的服务是一个挑战。本文将介绍如何使用Redis和C++构建高性能的图像处理应用,并提供一些代码示例。Redis是一个开源的内存数据库,具有高性能和高可用性的特点。它支持各种数据结构,如字符串、哈希表、列表等,同
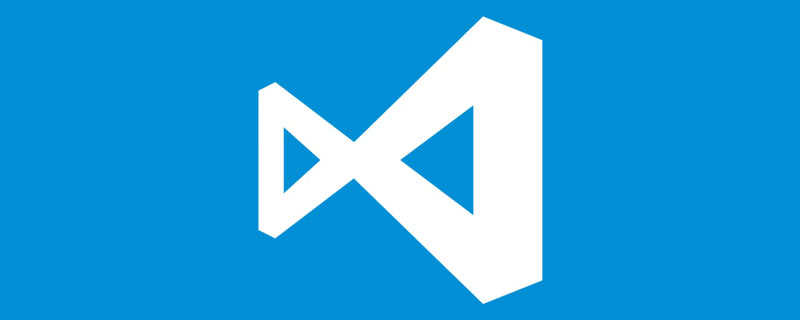
VSCode历史版本的下载安装 VSCode安装 下载 安装 参考资料 VSCode安装 Windows版本:Windows10 VSCode版本:VScode1.65.0(64位User版本) 本文


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
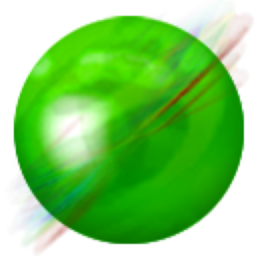
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
