


How to optimize the use of default parameters and variadic parameters in C++ functions
Optimize C default and variable parameter functions: Default parameters: Allow functions to use default values to reduce redundancy. Put default parameters last to improve readability. Use constexpr default parameters to reduce overhead. Use structured binding to improve readability of complex default parameters. Variable parameters: allows a function to accept a variable number of parameters. Try to avoid using variadic arguments and use them only when necessary. Optimize variadic functions using std::initializer_list to improve performance.
Optimize the use of default parameters and variable parameters in C functions
Default parameters
Default parameters allow functions to use default values when specific parameters are not provided. This helps reduce redundant code in function calls. For example:
int add(int a, int b = 0) { return a + b; } int main() { cout << add(1) << endl; // 输出 1 cout << add(1, 2) << endl; // 输出 3 }
Variadic parameters
Variadic parameters allow a function to accept a variable number of parameters. This is useful for functions that need to handle an unknown number of arguments. For example:
int sum(int num, ...) { va_list args; va_start(args, num); int sum = num; int arg; while ((arg = va_arg(args, int)) != 0) { sum += arg; } va_end(args); return sum; } int main() { cout << sum(1) << endl; // 输出 1 cout << sum(1, 2, 3, 4, 5) << endl; // 输出 15 }
Optimization tips
- Try to avoid using variable parameters: Variable parameters will introduce performance overhead, so only when Use them only when necessary.
- Place default parameters at the end: Placing default parameters at the end of the function parameter list can improve readability and maintainability.
- Use constexpr default parameters: If the default value is known and will not change, using the constexpr modifier can reduce overhead.
- Use structured binding: For complex default parameters, you can use structured binding to create more readable code.
Practical case:
Variable parameter optimization:
// 旧版:存在性能开销 int max(int num, ...) { va_list args; va_start(args, num); int max = num; int arg; while ((arg = va_arg(args, int)) != 0) { max = std::max(max, arg); } va_end(args); return max; } // 新版:使用 std::initializer_list 优化 int max(int num, std::initializer_list<int> args) { return *std::max_element(args.begin(), args.end(), [](int a, int b) { return a > b; }); }
Default parameter optimization:
// 旧版:默认值不在最后,可读性差 int add(int b, int a = 0) { return a + b; } // 新版:默认值在最后,可读性好 int add(int a, int b = 0) { return a + b; }
The above is the detailed content of How to optimize the use of default parameters and variadic parameters in C++ functions. For more information, please follow other related articles on the PHP Chinese website!
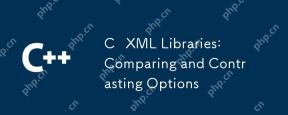
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
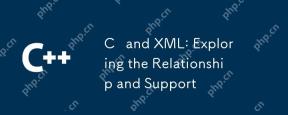
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
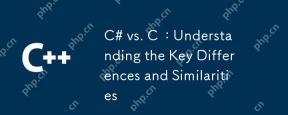
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
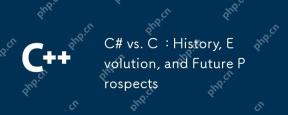
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
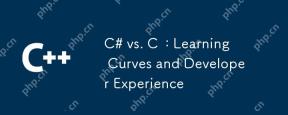
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
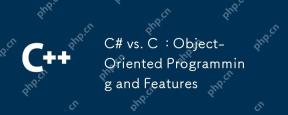
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
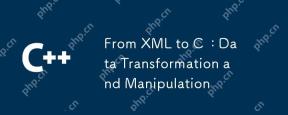
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
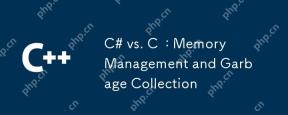
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.