How to manually manage memory in Java?
Manual memory management techniques in Java include: Reference counting: Tracks the number of references to an object, and releases object memory when the reference count reaches 0. Weak reference: A special reference type that does not prevent the object from being recycled when the garbage collector reclaims the object. Strong references: Ensure that an object will not be garbage collected, even if it is no longer needed.
Manual Memory Management in Java
Introduction
Java is a Automatic garbage collection languages usually do not require manual memory management. However, in some cases, manually managing memory can provide performance benefits or increased control over memory usage.
Reference Counting
Reference counting is a manual memory management technique that keeps track of the number of references each object has. When the reference count drops to 0, the object will be garbage collected. For example:
// 创建一个对象并将其存储在引用变量中 String myString = new String("Hello World"); // 获得对该对象的另一个引用 String anotherString = myString; // 释放 myString 对该对象的引用 myString = null; // 此时引用计数为 1(anotherString)
Weak reference
Weak reference is a special reference type that does not prevent the object from being recycled when the garbage collector reclaims it. For example:
// 创建一个弱引用 WeakReference<String> weakReference = new WeakReference<>(myString); // 释放对该对象的最后一个强引用 myString = null; // 此时 weakReference 仍然引用该对象,但该对象可以被垃圾回收
Practical case
Using soft references to cache images
Soft references can be used to cache images. When memory is low, soft reference objects can be garbage collected without reloading the image.
// 创建一个软引用缓存 Map<String, SoftReference<Image>> imageCache = new HashMap<>(); // 获取图像 Image image = getImage(url); // 将图像添加到缓存中 imageCache.put(url, new SoftReference<>(image)); // 获取图像 Image cachedImage = imageCache.get(url).get(); // 如果 cachedImage 为 null,则重新加载图像 if (cachedImage == null) { cachedImage = getImage(url); }
Use strong references to prevent objects from being garbage collected
Strong references ensure that an object will not be garbage collected, even if it is no longer needed. For example, in the singleton pattern, use a strong reference to ensure that only one instance is created.
// 创建单例的私有构造函数 private Singleton() { } // 创建一个强引用 private static final Singleton INSTANCE = new Singleton(); // 获取单例实例 public static Singleton getInstance() { return INSTANCE; }
Note: Manual memory management should be used with caution as it may cause memory leaks or other problems. In most cases, automatic garbage collection is sufficient for Java applications.
The above is the detailed content of How to manually manage memory in Java?. For more information, please follow other related articles on the PHP Chinese website!
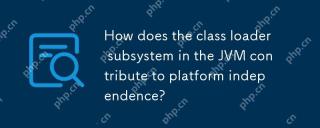
The class loader ensures the consistency and compatibility of Java programs on different platforms through unified class file format, dynamic loading, parent delegation model and platform-independent bytecode, and achieves platform independence.
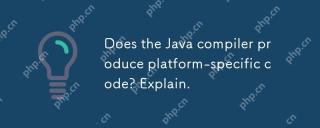
The code generated by the Java compiler is platform-independent, but the code that is ultimately executed is platform-specific. 1. Java source code is compiled into platform-independent bytecode. 2. The JVM converts bytecode into machine code for a specific platform, ensuring cross-platform operation but performance may be different.
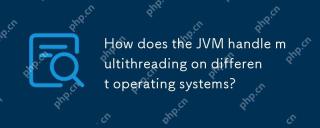
Multithreading is important in modern programming because it can improve program responsiveness and resource utilization and handle complex concurrent tasks. JVM ensures the consistency and efficiency of multithreads on different operating systems through thread mapping, scheduling mechanism and synchronization lock mechanism.
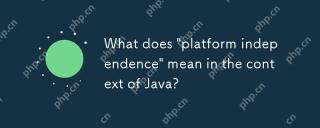
Java's platform independence means that the code written can run on any platform with JVM installed without modification. 1) Java source code is compiled into bytecode, 2) Bytecode is interpreted and executed by the JVM, 3) The JVM provides memory management and garbage collection functions to ensure that the program runs on different operating systems.
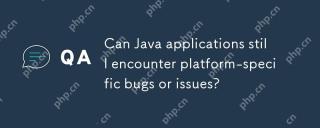
Javaapplicationscanindeedencounterplatform-specificissuesdespitetheJVM'sabstraction.Reasonsinclude:1)Nativecodeandlibraries,2)Operatingsystemdifferences,3)JVMimplementationvariations,and4)Hardwaredependencies.Tomitigatethese,developersshould:1)Conduc
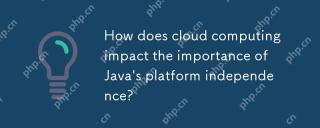
Cloud computing significantly improves Java's platform independence. 1) Java code is compiled into bytecode and executed by the JVM on different operating systems to ensure cross-platform operation. 2) Use Docker and Kubernetes to deploy Java applications to improve portability and scalability.
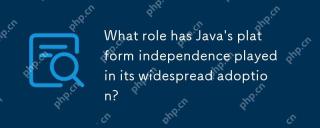
Java'splatformindependenceallowsdeveloperstowritecodeonceandrunitonanydeviceorOSwithaJVM.Thisisachievedthroughcompilingtobytecode,whichtheJVMinterpretsorcompilesatruntime.ThisfeaturehassignificantlyboostedJava'sadoptionduetocross-platformdeployment,s
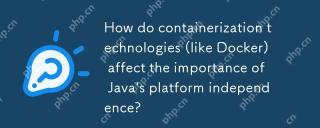
Containerization technologies such as Docker enhance rather than replace Java's platform independence. 1) Ensure consistency across environments, 2) Manage dependencies, including specific JVM versions, 3) Simplify the deployment process to make Java applications more adaptable and manageable.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),