How to call functions in different modules in C++?
Call functions across modules in C: Declare the function: Declare the function to be called in the header file of the target module. Implement function: Implement the function in the source file. Linking modules: Use a linker to link together modules containing function declarations and implementations. Call the function: Include the header file of the target module in the module that needs to be called, and then call the function.
Call functions across modules in C
In C, when the project scale increases, organize the code into different Modules improve maintainability and reusability. Modules also allow functions and variables to be shared between different modules. This tutorial will introduce how to call functions across modules and provide a practical case.
Header files and source files
Each module consists of two files: a header file and a source file. Header files contain declarations of functions and variables, while source files contain their implementations.
Function declaration
To make a function in one module callable from another module, the function must be declared in the header file. A function declaration specifies the function's return type, name, parameters, and return type. For example:
// moduleA.h int sum(int a, int b);
Function implementation
In the source file, implement the function. The source file contains the actual code of the function. For example:
// moduleA.cpp int sum(int a, int b) { return a + b; }
Linking modules
In order for the compiler to know where the function declaration and implementation are, the modules need to be linked together. This can be done using a linker, which combines the object files of different modules into a single executable file. In the command line, use the following command to link the module:
g++ -o executable moduleA.o moduleB.o
Practical case
Suppose we have two modules: moduleA
and moduleB
. There is a function named greet()
in moduleA
, and a function named print()
in moduleB
. We hope to be able to call the greet()
function in moduleA
from moduleB
.
Declare greet()
in moduleA.h
Function:
// moduleA.h void greet(string name);
Implemented in moduleA.cpp
greet()
Function:
// moduleA.cpp void greet(string name) { cout << "Hello, " << name << "!" << endl; }
In moduleB.cpp
, use the header file moduleA.h
and call greet()
Function:
// moduleB.cpp #include "moduleA.h" void print() { greet("John"); }
Link two modules:
g++ -o executable moduleA.o moduleB.o
Run the executable:
./executable
Output:
Hello, John!
The above is the detailed content of How to call functions in different modules in C++?. For more information, please follow other related articles on the PHP Chinese website!
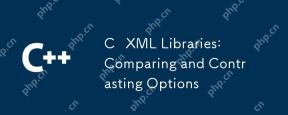
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
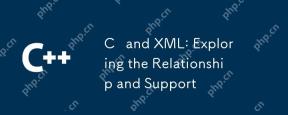
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
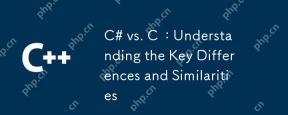
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
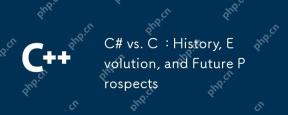
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
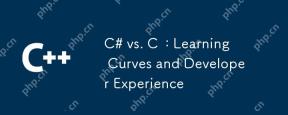
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
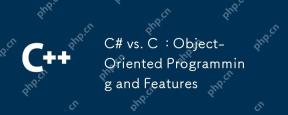
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
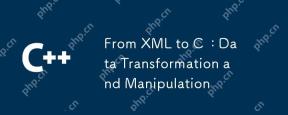
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
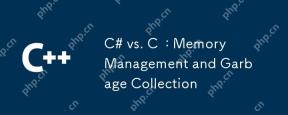
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
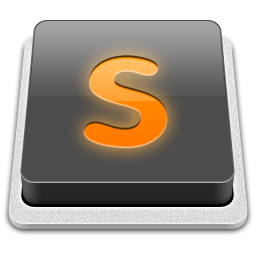
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor