Debugging exceptions are a critical part of software development in identifying and fixing code errors. Exception handling in Java is implemented using try-catch statements and provides built-in exception types (such as NullPointerException). To debug exceptions, you can use the IDE's debugger, the printStackTrace() method, or by analyzing the stack trace.
Debugging exceptions in Java
Preface
Debugging exceptions are a software development process A vital part of the code that helps us identify errors in our code and fix them. Java provides a rich exception handling mechanism that allows us to easily handle and debug exceptions.
Basic syntax for exception handling
In Java, exception handling is written using the try-catch
statement. The basic syntax is as follows:
try { // 可能会抛出异常的代码 } catch (ExceptionType exceptionVariable) { // 异常处理代码 }
Common exception types
There are many built-in exception types in Java, such as:
-
NullPointerException
: Thrown when a null object is referenced. -
IndexOutOfBoundsException
: Thrown when accessing an array or collection is out of scope. -
NumberFormatException
: Thrown when trying to parse a non-numeric string into a number.
Debug Exceptions
When an exception is thrown, Java prints out a stack trace. A stack trace contains a series of calls that indicate how an exception was generated.
To debug exceptions, we can:
- Use the IDE's debugger: Most IDEs have built-in debuggers that can set breakpoints in the code and see the value of the variable.
-
Using the
printStackTrace()
method: This method prints the exception and its stack trace to the console.
Practical case
Let's look at an example to demonstrate how to debug NullPointerException
:
public class Main { public static void main(String[] args) { String name = null; System.out.println(name.length()); // NullPointerException } }
When executing this code, a NullPointerException
will be thrown because name
is a null reference.
You can debug this exception by using the IDE's debugger or the printStackTrace()
method.
-
IDE debugger: Set a breakpoint on variable
name
to see that its value is null. - printStackTrace() method: The stack trace printed in the console is as follows:
java.lang.NullPointerException at Main.main(Main.java:9)
The stack trace indicates that the exception is in the Main
class main
Thrown on line 9 of the method.
Conclusion
The exception handling mechanism in Java allows us to easily identify and debug exceptions. By using the try-catch
statement we can handle exceptions and prevent them from causing the program to crash.
The above is the detailed content of How to debug exceptions in Java?. For more information, please follow other related articles on the PHP Chinese website!
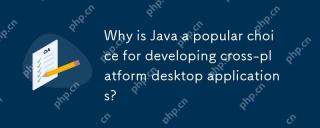
Javaispopularforcross-platformdesktopapplicationsduetoits"WriteOnce,RunAnywhere"philosophy.1)ItusesbytecodethatrunsonanyJVM-equippedplatform.2)LibrarieslikeSwingandJavaFXhelpcreatenative-lookingUIs.3)Itsextensivestandardlibrarysupportscompr
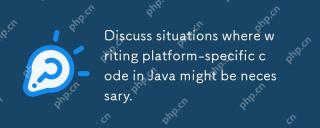
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
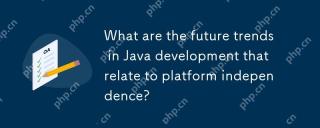
Java will further enhance platform independence through cloud-native applications, multi-platform deployment and cross-language interoperability. 1) Cloud native applications will use GraalVM and Quarkus to increase startup speed. 2) Java will be extended to embedded devices, mobile devices and quantum computers. 3) Through GraalVM, Java will seamlessly integrate with languages such as Python and JavaScript to enhance cross-language interoperability.
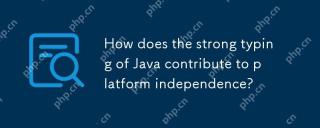
Java's strong typed system ensures platform independence through type safety, unified type conversion and polymorphism. 1) Type safety performs type checking at compile time to avoid runtime errors; 2) Unified type conversion rules are consistent across all platforms; 3) Polymorphism and interface mechanisms make the code behave consistently on different platforms.
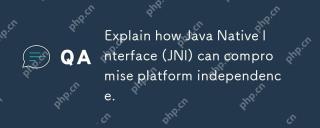
JNI will destroy Java's platform independence. 1) JNI requires local libraries for a specific platform, 2) local code needs to be compiled and linked on the target platform, 3) Different versions of the operating system or JVM may require different local library versions, 4) local code may introduce security vulnerabilities or cause program crashes.
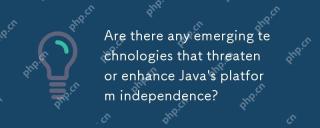
Emerging technologies pose both threats and enhancements to Java's platform independence. 1) Cloud computing and containerization technologies such as Docker enhance Java's platform independence, but need to be optimized to adapt to different cloud environments. 2) WebAssembly compiles Java code through GraalVM, extending its platform independence, but it needs to compete with other languages for performance.
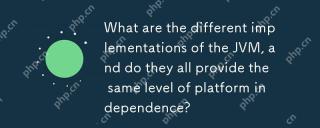
Different JVM implementations can provide platform independence, but their performance is slightly different. 1. OracleHotSpot and OpenJDKJVM perform similarly in platform independence, but OpenJDK may require additional configuration. 2. IBMJ9JVM performs optimization on specific operating systems. 3. GraalVM supports multiple languages and requires additional configuration. 4. AzulZingJVM requires specific platform adjustments.
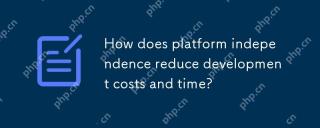
Platform independence reduces development costs and shortens development time by running the same set of code on multiple operating systems. Specifically, it is manifested as: 1. Reduce development time, only one set of code is required; 2. Reduce maintenance costs and unify the testing process; 3. Quick iteration and team collaboration to simplify the deployment process.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
