


Best practices for implementing cross-platform desktop applications in Golang
Best practices provide guidance for building cross-platform desktop applications using the Qt framework, including: separating UI and business logic, using Goroutines, using QML, and taking advantage of the concurrency features of the Go language. Practical case shows how to use Qt and Go to build a cross-platform text editor.
Best practices for implementing cross-platform desktop applications in Go language
Introduction
## The #Go language is a powerful programming language that provides features for building efficient, portable applications. This article will introduce the best practices for building cross-platform desktop applications using the Go language and provide practical cases.Using the Qt framework
Qt is a cross-platform application framework that provides rich GUI components and support for multiple operating systems. The Go language is integrated with Qt through the [go-bindings](https://github.com/therecipe/qt) project, allowing developers to build Qt applications using Go.Best Practice
- Separate UI and business logic: Follow the MVC design pattern and separate UI (view) and business logic ( model and controller) separately. This makes the code easier to maintain and test.
- Using Goroutines: Goroutines are lightweight threads in the Go language that allow asynchronous execution of tasks. This is crucial for responsive GUI applications.
- Using QML: QML (Qt metalanguage) is a language for describing UI declaratively. It makes it easier to create complex UI layouts and animations.
- Using Go: The concurrency nature of the Go language makes it ideal for handling events and operations from the GUI. For example, you can use channels and mutexes for communication and synchronization.
Practical case
The following is an example of a cross-platform text editor built using Qt and Go:package main import ( "fmt" "log" "github.com/therecipe/qt/core" "github.com/therecipe/qt/gui" "github.com/therecipe/qt/widgets" ) func main() { app := gui.NewQGuiApplication(len(os.Args), os.Args) w := widgets.NewQMainWindow(nil, 0) te := widgets.NewQTextEdit(w) w.SetCentralWidget(te)
The above is the detailed content of Best practices for implementing cross-platform desktop applications in Golang. For more information, please follow other related articles on the PHP Chinese website!
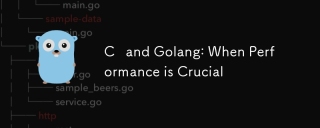
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
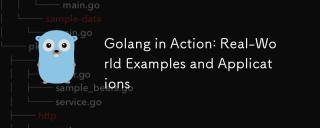
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
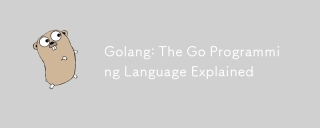
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
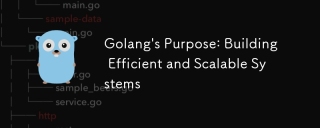
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
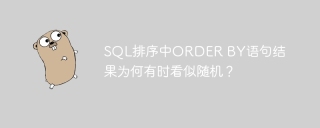
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
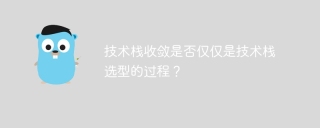
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
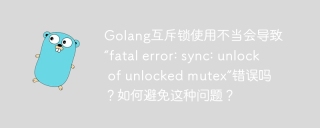
Golang ...
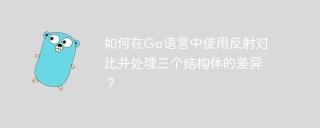
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
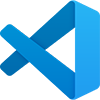
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
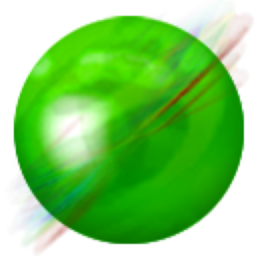
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
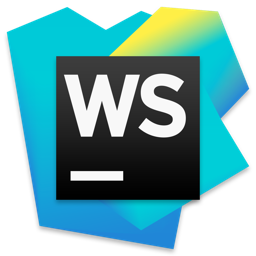
WebStorm Mac version
Useful JavaScript development tools